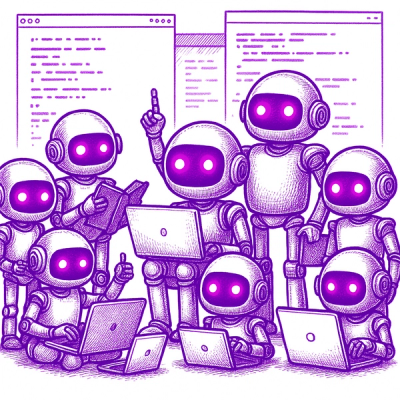
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
pm2-deploy
Advanced tools
The pm2-deploy npm package is a module for PM2, which allows users to perform deployment tasks for their applications. It simplifies the process of updating, reverting, and managing deployments directly from the command line.
Setup
Initializes the remote production environment, setting up the necessary directories and configurations for deployment.
pm2 deploy production setup
Deployment
Deploys the application to the production environment as specified in the ecosystem configuration file.
pm2 deploy ecosystem.config.js production
Update
Updates the deployment with the latest changes from the source without a full redeployment.
pm2 deploy production update
Revert
Reverts to the previous deployment (or a specified number of revisions back) in case of deployment issues.
pm2 deploy production revert 1
Execution of custom commands
Executes a custom command on the remote server, such as reloading all PM2 processes.
pm2 deploy production exec 'pm2 reload all'
Shipit is an automation engine and a deployment tool that offers a similar feature set to pm2-deploy. It provides easy rollbacks, task definitions, and workflow automation. Unlike pm2-deploy, which is tightly coupled with PM2, Shipit can be used independently of any process manager.
Capistrano is a remote server automation and deployment tool written in Ruby. It supports scripting and task automation and is often used for deploying web applications. It is more established than pm2-deploy but requires Ruby environment, whereas pm2-deploy is specific to Node.js environments.
Deployer is a PHP deployment tool with support for several popular frameworks. It is similar to pm2-deploy in that it automates deployment tasks but is used in PHP environments. Deployer offers parallel deployment, atomic deployment, and zero downtime deployments.
This is the module that allows to do pm2 deploy
.
Documentation: http://pm2.keymetrics.io/docs/usage/deployment/
$ npm install pm2-deploy
var deployForEnv = require('pm2-deploy').deployForEnv;
// Define deploy configuration with target environments
var deployConfig = {
prod: {
user: 'node',
host: '212.83.163.168',
ref: 'origin/master',
repo: 'git@github.com:Unitech/eip-vitrine.git',
path: '/var/www/test-deploy'
},
dev: {
user: 'node',
host: '212.83.163.168',
ref: 'origin/master',
repo: 'git@github.com:Unitech/eip-vitrine.git',
path: '/var/www/test-dev'
}
};
// Invoke deployment for `dev` environment
deployForEnv(deployConfig, 'dev', [], function (err, args) {
if (err) {
console.error('Deploy failed:', err.message);
return console.error(err.stack);
}
console.log('Success!');
});
// Rollback `prod` environment
deployForEnv(deployConfig, 'prod', ['revert', 1], function (err, args) {
if (err) {
console.error('Rollback failed:', err.message);
return console.error(err.stack);
}
console.log('Success!');
});
Deploy to a single environment
deployConfig
object object containing deploy configs for all environmentsenv
string the name of the environment to deploy toargs
array custom deploy command-line argumentscb
DeployCallback done callbackReturns boolean return value is always false
Type: Function
FAQs
Deployment system for PM2
The npm package pm2-deploy receives a total of 1,372,408 weekly downloads. As such, pm2-deploy popularity was classified as popular.
We found that pm2-deploy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.