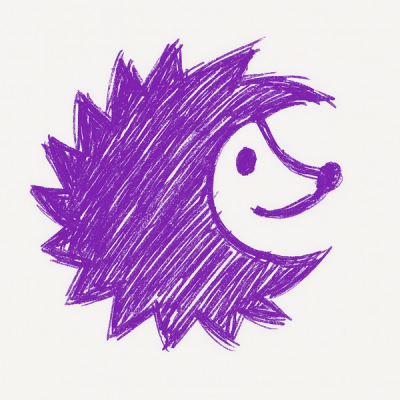
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
postcss-load-config
Advanced tools
The postcss-load-config package is used to load PostCSS configurations automatically. It helps in finding and loading a PostCSS configuration file, which can be in different formats like .postcssrc, postcss.config.js, or directly in package.json. It simplifies the process of setting up PostCSS by abstracting the configuration loading logic.
Loading PostCSS Configurations
This feature allows you to load the PostCSS configuration from a file or package.json. The code sample demonstrates how to use the package to load the configuration and then use it to process CSS with PostCSS.
const postcssLoadConfig = require('postcss-load-config');
postcssLoadConfig().then(({ plugins, options }) => {
// Use the loaded plugins and options to process your CSS with PostCSS
postcss(plugins).process(yourCss, options);
}).catch((error) => {
console.error('Failed to load PostCSS config:', error);
});
Cosmiconfig is a package that searches for and loads configuration for your program. It supports several file formats and locations for the configurations. It is similar to postcss-load-config but is more generic and not limited to PostCSS configurations.
webpack-config-loader is a package that helps load and customize webpack configurations. It is similar to postcss-load-config in that it abstracts the configuration loading process, but it is specific to webpack rather than PostCSS.
require-from-string allows you to require modules from a string of code. It is similar to postcss-load-config in that it can be used to load configurations dynamically, but it does not have the built-in logic to search for configuration files.
npm i -D postcss-load-config
npm i -S|-D postcss-plugin
Install all required PostCSS plugins and save them to your package.json dependencies
/devDependencies
Then create a PostCSS config file by choosing one of the following formats
package.json
Create a postcss
section in your project's package.json
Project (Root)
|– client
|– public
|
|- package.json
{
"postcss": {
"parser": "sugarss",
"map": false,
"plugins": {
"postcss-plugin": {}
}
}
}
.postcssrc
Create a .postcssrc
file in JSON or YAML format
ℹ️ It's recommended to use an extension (e.g
.postcssrc.json
or.postcssrc.yml
) instead of.postcssrc
Project (Root)
|– client
|– public
|
|- (.postcssrc|.postcssrc.json|.postcssrc.yml)
|- package.json
.postcssrc.json
{
"parser": "sugarss",
"map": false,
"plugins": {
"postcss-plugin": {}
}
}
.postcssrc.yml
parser: sugarss
map: false
plugins:
postcss-plugin: {}
[!NOTE] For YAML configs, you must have yaml installed as a peer dependency.
.postcssrc.js
or postcss.config.js
You may need some logic within your config. In this case create JS/TS file named:
.postcssrc.js
.postcssrc.mjs
.postcssrc.cjs
.postcssrc.ts
.postcssrc.mts
.postcssrc.cts
postcss.config.js
postcss.config.mjs
postcss.config.cjs
postcss.config.ts
postcss.config.mts
postcss.config.cts
[!NOTE] For TypeScript configs, you must have tsx or jiti installed as a peer dependency.
Project (Root)
|– client
|– public
|- (.postcssrc|postcss.config).(js|mjs|cjs|ts|mts|cts)
|- package.json
You can export the config as an {Object}
.postcssrc.js
module.exports = {
parser: 'sugarss',
map: false,
plugins: {
'postcss-plugin': {}
}
}
Or export a {Function}
that returns the config (more about the ctx
param below)
.postcssrc.js
module.exports = (ctx) => ({
parser: ctx.parser ? 'sugarss' : false,
map: ctx.env === 'development' ? ctx.map : false,
plugins: {
'postcss-plugin': ctx.options.plugin
}
})
Plugins can be loaded either using an {Object}
or an {Array}
{Object}
.postcssrc.js
module.exports = ({ env }) => ({
...options,
plugins: {
'postcss-plugin': env === 'production' ? {} : false
}
})
ℹ️ When using an
{Object}
, the key can be a Node.js module name, a path to a JavaScript file that is relative to the directory of the PostCSS config file, or an absolute path to a JavaScript file.
{Array}
.postcssrc.js
module.exports = ({ env }) => ({
...options,
plugins: [
env === 'production' ? require('postcss-plugin')() : false
]
})
:warning: When using an
{Array}
, make sure torequire()
each plugin
Name | Type | Default | Description |
---|---|---|---|
to | {String} | undefined | Destination File Path |
map | {String|Object} | false | Enable/Disable Source Maps |
from | {String} | undefined | Source File Path |
parser | {String|Function} | false | Custom PostCSS Parser |
syntax | {String|Function} | false | Custom PostCSS Syntax |
stringifier | {String|Function} | false | Custom PostCSS Stringifier |
parser
.postcssrc.js
module.exports = {
parser: 'sugarss'
}
syntax
.postcssrc.js
module.exports = {
syntax: 'postcss-scss'
}
stringifier
.postcssrc.js
module.exports = {
stringifier: 'midas'
}
map
.postcssrc.js
module.exports = {
map: 'inline'
}
:warning: In most cases
options.from
&&options.to
are set by the third-party which integrates this package (CLI, gulp, webpack). It's unlikely one needs to set/useoptions.from
&&options.to
within a config file. Unless you're a third-party plugin author using this module and its Node API directly dont't setoptions.from
&&options.to
yourself
to
module.exports = {
to: 'path/to/dest.css'
}
from
module.exports = {
from: 'path/to/src.css'
}
{} || null
The plugin will be loaded with defaults
'postcss-plugin': {} || null
.postcssrc.js
module.exports = {
plugins: {
'postcss-plugin': {} || null
}
}
:warning:
{}
must be an empty{Object}
literal
{Object}
The plugin will be loaded with given options
'postcss-plugin': { option: '', option: '' }
.postcssrc.js
module.exports = {
plugins: {
'postcss-plugin': { option: '', option: '' }
}
}
false
The plugin will not be loaded
'postcss-plugin': false
.postcssrc.js
module.exports = {
plugins: {
'postcss-plugin': false
}
}
Ordering
Plugin execution order is determined by declaration in the plugins section (top-down)
{
plugins: {
'postcss-plugin': {}, // [0]
'postcss-plugin': {}, // [1]
'postcss-plugin': {} // [2]
}
}
When using a {Function}
(postcss.config.js
or .postcssrc.js
), it's possible to pass context to postcss-load-config
, which will be evaluated while loading your config. By default ctx.env (process.env.NODE_ENV)
and ctx.cwd (process.cwd())
are available on the ctx
{Object}
ℹ️ Most third-party integrations add additional properties to the
ctx
(e.gpostcss-loader
). Check the specific module's README for more information about what is available on the respectivectx
postcss.config.js
module.exports = (ctx) => ({
parser: ctx.parser ? 'sugarss' : false,
map: ctx.env === 'development' ? ctx.map : false,
plugins: {
'postcss-import': {},
'postcss-nested': {},
cssnano: ctx.env === 'production' ? {} : false
}
})
"scripts": {
"build": "NODE_ENV=production node postcss",
"start": "NODE_ENV=development node postcss"
}
const { readFileSync } = require('fs')
const postcss = require('postcss')
const postcssrc = require('postcss-load-config')
const css = readFileSync('index.css', 'utf8')
const ctx = { parser: true, map: 'inline' }
postcssrc(ctx).then(({ plugins, options }) => {
postcss(plugins)
.process(css, options)
.then((result) => console.log(result.css))
})
"scripts": {
"build": "NODE_ENV=production gulp",
"start": "NODE_ENV=development gulp"
}
const { task, src, dest, series, watch } = require('gulp')
const postcss = require('gulp-postcssrc')
const css = () => {
src('src/*.css')
.pipe(postcss())
.pipe(dest('dest'))
})
task('watch', () => {
watch(['src/*.css', 'postcss.config.js'], css)
})
task('default', series(css, 'watch'))
"scripts": {
"build": "NODE_ENV=production webpack",
"start": "NODE_ENV=development webpack-dev-server"
}
webpack.config.js
module.exports = (env) => ({
module: {
rules: [
{
test: /\.css$/,
use: [
'style-loader',
'css-loader',
'postcss-loader'
]
}
]
}
})
![]() Michael Ciniawsky |
![]() Mateusz Derks |
To report a security vulnerability, please use the Tidelift security contact. Tidelift will coordinate the fix and disclosure.
![]() Ryan Dunckel |
![]() Patrick Gilday |
![]() Dalton Santos |
![]() François Wouts |
FAQs
Autoload Config for PostCSS
The npm package postcss-load-config receives a total of 17,204,586 weekly downloads. As such, postcss-load-config popularity was classified as popular.
We found that postcss-load-config demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.