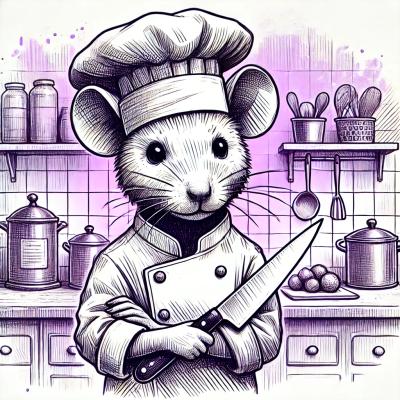
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
postcss-syntax
Advanced tools
The postcss-syntax npm package is a syntax parser for PostCSS that allows you to parse and stringify CSS, LESS, SCSS, and other syntaxes in a unified way. It enables PostCSS to understand these various syntaxes, which can be useful for tasks such as linting, minification, and applying other PostCSS plugins to non-standard CSS files.
Parsing CSS-like syntaxes
This feature allows you to use PostCSS to parse different CSS-like syntaxes such as SCSS or LESS. The code sample demonstrates how to process a source string with PostCSS using the postcss-syntax package to handle different syntaxes.
const postcss = require('postcss');
const syntax = require('postcss-syntax');
postcss(plugins).process(source, { syntax: syntax }).then(result => {
console.log(result.content);
});
Stringifying parsed syntaxes
After parsing CSS-like syntaxes, postcss-syntax can also be used to stringify the parsed abstract syntax tree (AST) back into a CSS string. This is useful for transforming code and then outputting the result.
const postcss = require('postcss');
const syntax = require('postcss-syntax');
const root = postcss.parse(source, { syntax: syntax });
const css = root.toString(syntax);
This package is a SCSS parser for PostCSS. It allows you to work with SCSS syntax specifically, whereas postcss-syntax is more general and can handle multiple syntaxes.
Similar to postcss-scss, postcss-less is a parser that allows PostCSS to understand LESS syntax. It is specifically tailored for LESS, unlike postcss-syntax which is more versatile.
SugarSS is an indented syntax parser for PostCSS. It is a more specialized tool compared to postcss-syntax, focusing on a syntax that is similar to Sass or Stylus but with a different set of features.
postcss-syntax can automatically switch the required PostCSS syntax by file extension/source
First thing's first, install the module:
npm install postcss-syntax --save-dev
If you want support SCSS/SASS/LESS/SugarSS syntax, you need to install these module:
If you want support HTML (and HTML-like)/Markdown/CSS-in-JS file format, you need to install these module:
const postcss = require('postcss');
const syntax = require('postcss-syntax')({
rules: [
{
test: /\.(?:[sx]?html?|[sx]ht|vue|ux|php)$/i,
extract: 'html',
},
{
test: /\.(?:markdown|md)$/i,
extract: 'markdown',
},
{
test: /\.(?:m?[jt]sx?|es\d*|pac)$/i,
extract: 'jsx',
},
{
// custom language for file extension
test: /\.postcss$/i,
lang: 'scss'
},
{
// custom language for file extension
test: /\.customcss$/i,
lang: 'custom'
},
],
// custom parser for CSS (using `postcss-safe-parser`)
css: 'postcss-safe-parser',
// custom parser for SASS (PostCSS-compatible syntax.)
sass: require('postcss-sass'),
// custom parser for SCSS (by module name)
scss: 'postcss-scss',
// custom parser for LESS (by module path)
less: './node_modules/postcss-less',
// custom parser for SugarSS
sugarss: require('sugarss'),
// custom parser for custom language
custom: require('postcss-custom-syntax'),
});
postcss(plugins).process(source, { syntax: syntax }).then(function (result) {
// An alias for the result.css property. Use it with syntaxes that generate non-CSS output.
result.content
});
FAQs
Automatically switch PostCSS syntax by file extensions
The npm package postcss-syntax receives a total of 809,848 weekly downloads. As such, postcss-syntax popularity was classified as popular.
We found that postcss-syntax demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.