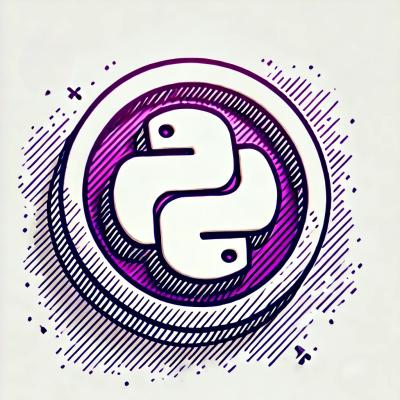
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
prompt-base
Advanced tools
Base prompt module used for creating custom prompt types for Enquirer.
Install with npm:
$ npm install --save prompt-base
See the examples folder for more detailed usage examples.
var Prompt = require('prompt-base');
var prompt = new Prompt({
name: 'color',
message: 'What is your favorite color?'
});
prompt.run()
.then(function(answer) {
console.log(answer);
})
Create a new Prompt with the given question
object, answers
and optional instance of readline-ui.
Params
question
{Object}: Plain object or instance of prompt-question.answers
{Object}: Optionally pass an answers object from a prompt manager (like enquirer).ui
{Object}: Optionally pass an instance of readline-ui. If not passed, an instance is created for you.Example
var prompt = new Prompt({
name: 'color',
message: 'What is your favorite color?'
});
prompt.ask(function(answer) {
console.log(answer);
//=> 'blue'
});
Returns a formatted prompt message.
returns
{String}Default ask
method. This mayb eb overridden in custom prompts.
Initialize a prompt and resolve answers. If question.when
returns false, the prompt will be skipped.
Params
answers
{Object}returns
{Promise}Render the current prompt input. This can be replaced by custom prompts.
Example
prompt.ui.on('keypress', prompt.render.bind(prompt));
Move the cursor in the given direction
when a keypress
event is emitted.
Params
direction
{String}event
{Object}Default return
event handler. This may be overridden in custom prompts.
Params
event
{Object}Default error event handler. If an error
listener exist, an error
event will be emitted, otherwise the error is logged onto stderr
and
the process is exited. This can be overridden in custom prompts.
Params
err
{Object}Default keypress
event handler. This may be overridden
in custom prompts.
Params
event
{Object}Default number
event handler. This may be overridden in
custom prompts.
Params
event
{Object}Default space
event handler. This may be overridden in custom prompts.
Params
event
{Object}When the answer is submitted (user presses enter
key), re-render
and pass answer to callback. This may be replaced by custom prompts.
Params
input
{Object}Default tab
event handler. This may be overridden in custom prompts.
Params
event
{Object}Proxy to readline.write for manually writing output. When called, rl.write() will resume the input stream if it has been paused.
returns
{undefined}Example
prompt.write('blue\n');
prompt.write(null, {ctrl: true, name: 'l'});
Getter for getting the choices array from the question.
returns
{Object}: Choices objectGetter that returns question.message
after passing it to format.
returns
{String}: A formatted prompt message.Getter that returns the prefix to use before question.message
. The default value is a green ?
.
returns
{String}: The formatted prefix.Example
prompt.prefix = '!';
Create a new Separator
object. See choices-separator for more details.
Params
separator
{String}: Optionally pass a string to use as the separator.returns
{Object}: Returns a separator object.Example
new Prompt.Separator('---');
Instantiate
The main purpose of this library is to serve as a base for other libraries to create custom prompt types. However, the main export is a function that can be instantiated to run basic "input" prompts.
var Prompt = require('prompt-base');
var prompt = new Prompt({
name: 'first',
message: 'What is your name?'
});
// callback
prompt.ask(function(answer) {
console.log(answer);
//=> 'Jon'
});
// promise
prompt.run()
.then(function(answers) {
console.log(answers);
//=> {first: 'Jon'}
});
Inherit
var Prompt = require('prompt-base');
function CustomPrompt(/*question, answers, rl*/) {
Prompt.apply(this, arguments);
}
Prompt.extend(CustomPrompt);
How it works
Debugging readline issues can be a pain. If you're experiencing something that seems like a bug, please let us know about it. If you happen to be in the mood for debugging, here are some suggestions and/or places to look to help you figure out what's happening.
Tips
process.exit()
after logging out the value as you're debugging. This not only stops the process immediately, letting you know if the method was even executed, but it's also more likely to make whatever you're logging out visible before it's overwritten by the readlineconsole.log([foo])
instead of console.log(foo)
. I do this when debugging just about anything, as it forces the value to be rendered literally, instead of being formatted as output for the terminal.In prompt-base (this module):
Log out the answer
value or any variants, like this.answer
, or input
in methods like onSubmit
, and submitAnswer
.
console.log([this.answer]);
// etc...
In the .action
method, log out the arguments object:
utils.action = function(state, str, key) {
console.log([arguments]);
process.exit();
// other code
};
In the .normalize
method in readline-utils, log out the arguments object:
utils.normalize = function(s, key) {
console.log([arguments]);
process.exit();
// other code
};
Log out the keypress
events in the listener.
Misc
In libraries with prompt-*
or readline-*
, or enquirer-*
in the name, look for places where something is .emit
ing, or listening with .on
or .only
(which simply wraps .on
to ensure that events aren't stacked when nested prompts are called), and log out the value there.
The following custom prompts were created using this library:
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Please read the contributing guide for advice on opening issues, pull requests, and coding standards.
Commits | Contributor |
---|---|
63 | jonschlinkert |
6 | doowb |
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb#dev verb-generate-readme && verb
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
Jon Schlinkert
Copyright © 2017, Jon Schlinkert. Released under the MIT License.
This file was generated by verb-generate-readme, v0.6.0, on May 11, 2017.
FAQs
Base prompt module used for creating custom prompts.
The npm package prompt-base receives a total of 42,620 weekly downloads. As such, prompt-base popularity was classified as popular.
We found that prompt-base demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.