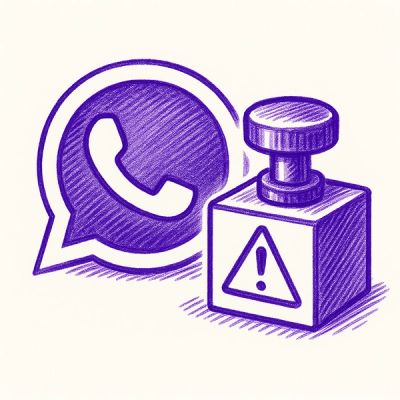
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
protect-group-refundable-react
Advanced tools
React component to display Protect Group Refundable Content, and send booking data
This component is designed to place our Refundable Content on your React app, and allow you to also send transactions into our platform using a simple hook.
npm i --save protect-group-refundable-react
Add the provider somewhere near the root of your application
import {
RefundableOptions,
RefundableProvider
} from 'protect-group-refundable-react'
function MyApp() {
const options: RefundableOptions = {
environment: 'test',
currencyCode: 'GBP',
languageCode: 'en',
offeringMethod: 'OPT-OUT',
productCode: 'PKG',
vendorCode: '<YOUR-VENDOR-CODE>',
eventDateFormat: 'DD/MM/YYYY',
premium: 10,
whiteLabelled: false,
displayTrustPilot: true,
displayRefundText: true,
allowNonRefundableLabelClick: false,
labelText: 'Default',
vertical: 'Default',
compact: false
}
return (
<RefundableProvider options={options}>
<TheRestOfYourApp/>
</RefundableProvider>
)
}
Add the RefundableContent component where you would like our Refundable Content to be placed on your page. Use the provided hook to write a transaction into our platform
import {
RefundableContent,
ProtectionChangeEventArgs,
useRefundableActions,
WriteTransactionRequest
} from 'protect-group-refundable-react'
function MyCheckoutPage() {
const {writeTransaction} = useRefundableActions()
const handleProtectionChange = (args: ProtectionChangeEventArgs) => {
//do something with the args
}
const handleMakePayment = async () => {
try {
// Do your payment thing
const request: WriteTransactionRequest = {
bookingReference: 'your-booking-reference',
eventDate: '31/12/2022',
customerFirstName: 'Test',
customerLastName: 'Test'
}
const response = await writeTransaction(request)
// Do something with the response
} catch (e) {
// Something went wrong somewhere
}
}
return (
<MyPageWrapper>
//some components
<RefundableContent bookingCost={10} onProtectionChange={handleProtectionChange}/>
//some components
<MakePaymentButton onClick={handleMakePayment}></MakePaymentButton>
</MyPageWrapper>
)
}
Property | Type | Required? | Default | Description |
---|---|---|---|---|
environment | Environment | yes | Send requests to either our test or production api. Should be either 'test' or 'prod' | |
currencyCode | string | yes | A valid currency code | |
languageCode | string | yes | A valid language code. Currently supported languages are 'en', 'fr', 'es' and 'pt' | |
offeringMethod | OfferingMethod | yes | Controls whether a refundable booking is pre-selected or not | |
productCode | ProductCode | yes | The type of product offered to the customer | |
vendorCode | string | yes | Your vendor code supplied by our commercial team. Please ensure the correct vendor code is supplied for the environment | |
eventDateFormat | string | no | Our api expects a date format of ISO 861 yyyy-MM-ddTHH:mm:ss.FFFFFzzz. If you are unable to supply a date in this format you can optionally configure the format here. The format you supply must match a javascript date format from the moment.js library | |
premium | number | yes | The protection rate applied to the cost of a booking | |
whiteLabelled | boolean | no | false | Our Refundable Content comes styled. If you wish to theme the content to match our site, set this to true. Each of the sections in the content will then have well names classes you can target |
displayTrustPilot | boolean | no | true | Displays our TrustPilot widget |
displayRefundText | boolean | no | false | Displays a line of text showing the average time it takes to process a refund application |
allowNonRefundableLabelClick | boolean | no | false | Allow the entire label of the non-refundable booking option to be selected |
labelText | LabelText | no | 'Default' | Allows a subtly different title to be displayed |
vertical | Vertical | no | 'Default' | We can tailor the wording of different verticals to provide an optimum experience for a customer |
compact | boolean | no | false | We can tailor a more compact version the wording on different verticals to provide an optimum experience for a customer |
Property | Type | Required? | Default | Description |
---|---|---|---|---|
bookingCost | number | yes | The cost of the booking | |
onProtectionChange | function | yes | Function that is invoked when any of the components internal state changes |
The parameter passed into the onProtectionChange function
interface ProtectionChangeEventArgs {
protectionSelected: boolean|null
protectionValue: number
bookingCost: number
totalValue: number
formattedProtectionValue: string
formattedBookingCost: string
formattedTotalValue: string
}
protectionSelected will only ever be null when the offeringMethod is SELECT and the user has not clicked one of the options
useRefundableActions
returns an object containing functions to interact with our platform
interface WriteTransactionRequest {
bookingReference: string
eventDate: string
customerFirstName: string
customerLastName: string
}
interface WriteTransactionResponse {
refundableLink: string
refundableConfirmationHtml: string
}
interface RefundableActions {
writeTransaction: (request: WriteTransactionRequest) => Promise<WriteTransactionResponse>
cancelTransaction: (bookingReference: string) => Promise<any>
}
import {useRefundableActions} from 'protect-group-refundable-react'
function YourComponent() {
const {writeTransaction, cancelTransaction} = useRefundableActions()
const handleCancelClick = async () => {
try {
await cancelTransaction('[your-booking-reference]')
} catch (e) {
// This might happen if you try and cancel immediately after calling writeTransaction
// The transaction might not have been written into our platform just yet, it's usually there within a couple of seconds
}
}
}
useRefundableContext
provides access to the RefundableContextOptions
interface, which has functions allowing
you to update the booking cost and currency code using functions, if required at runtime
interface RefundableOptions {
environment: Environment
vendorCode: string
currencyCode: string
languageCode: string
productCode: ProductCode
offeringMethod: OfferingMethod
premium: number
eventDateFormat?: string
displayTrustPilot?: boolean
displayRefundText?: boolean
labelText?: LabelText
vertical?: Vertical
whiteLabelled?: boolean
allowNonRefundableLabelClick?: boolean
compact?: boolean
}
interface RefundableContextOptions extends RefundableOptions {
bookingData: BookingData
isWhiteLabelled: boolean
isCompact: boolean
toggleProtectionSelected: (isSelected: boolean) => void
updateBookingCost: (bookingCost: number) => void
updateCurrency: (currencyCode: string) => void
}
import {useRefundableContext} from 'protect-group-refundable-react'
function YourComponent() {
const {updateBookingCost} = useRefundableContext()
}
FAQs
React component to display Protect Group Refundable Content, and send booking data
The npm package protect-group-refundable-react receives a total of 39 weekly downloads. As such, protect-group-refundable-react popularity was classified as not popular.
We found that protect-group-refundable-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.