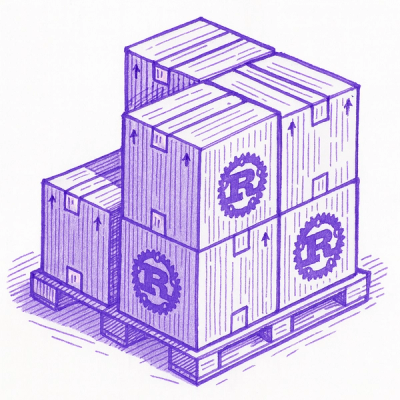
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
The 'q' npm package is a library for creating and managing promises in JavaScript. It provides a robust set of tools for working with asynchronous operations, allowing developers to write cleaner, more maintainable code by avoiding the 'callback hell' that can occur with deeply nested callbacks.
Creating Promises
This feature allows the creation of a new promise using Q.defer(). The deferred object has a promise property and methods for resolving or rejecting the promise.
const Q = require('q');
const deferred = Q.defer();
function asyncOperation() {
// Perform some asynchronous operation
setTimeout(() => {
// Resolve the promise after 1 second
deferred.resolve('Operation completed');
}, 1000);
return deferred.promise;
}
asyncOperation().then(result => console.log(result));
Promise Chaining
This feature demonstrates how promises can be chained together, with the output of one promise being passed as input to the next.
const Q = require('q');
function firstAsyncOperation() {
var deferred = Q.defer();
setTimeout(() => deferred.resolve(1), 1000);
return deferred.promise;
}
function secondAsyncOperation(result) {
var deferred = Q.defer();
setTimeout(() => deferred.resolve(result + 1), 1000);
return deferred.promise;
}
firstAsyncOperation()
.then(secondAsyncOperation)
.then(result => console.log('Final result:', result));
Error Handling
This feature shows how to handle errors in promise-based workflows. The catch method is used to handle any errors that occur during the promise's execution.
const Q = require('q');
function mightFailOperation() {
var deferred = Q.defer();
setTimeout(() => {
if (Math.random() > 0.5) {
deferred.resolve('Success!');
} else {
deferred.reject(new Error('Failed!'));
}
}, 1000);
return deferred.promise;
}
mightFailOperation()
.then(result => console.log(result))
.catch(error => console.error(error.message));
Bluebird is a fully-featured promise library with a focus on innovative features and performance. It is known for being one of the fastest promise libraries and includes utilities for concurrency, such as Promise.map and Promise.reduce, which are not present in 'q'.
When is another lightweight Promise library that offers similar functionality to 'q'. It provides a solid API for creating and managing promises but is generally considered to have a smaller footprint and to be more modular than 'q'.
This package is a simple implementation of Promises/A+. It is smaller and may be more straightforward than 'q' for those who only need basic promise functionality without the additional utilities provided by 'q'.
0.8.7
FAQs
A library for promises (CommonJS/Promises/A,B,D)
The npm package q receives a total of 10,292,222 weekly downloads. As such, q popularity was classified as popular.
We found that q demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.