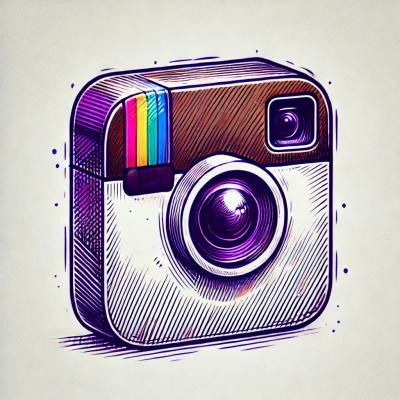
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
This API is an easy way to use the Questrade API immediately.
If you're looking for the old API docs, click here.
Simply start by installing the questrade library:
npm install --save questrade
You will then need to get an API key.
Important note about key management:
After that's it's really simple to use, but you WILL need to save the new API key and use it every time you try to reconnect. The API key Questrade initially gives you will no longer be valid after you call connect
.
const Questrade = require('questrade');
const qt = new Questrade('<your-api-key-here>');
// Connect to Questrade
const newKey = await qt.connect()
// Save newKey for next time
// Access your account here
const account = await qt.getPrimaryAccount()
const accounts = await qt.getAccounts()
const balances = await account.getBalances()
// Get Market quotes
const msft = await qt.getSymbol('MSFT')
const quote = await msft.getQuote()
const options = await msft.getOptionChain()
// ... etc. See the full documentation for all the calls you can make!
const account = await qt.getPrimaryAccount() // Account
const markets = await qt.getMarkets()
const accounts = await qt.getAccounts() // => [Account]
const balances = await account.getBalances()
const activities = await account.getActivities()
const orders = await qt.getOpenOrders() // [Order]
const orders = await qt.getOrders() // [Order]
const orders = await qt.getClosedOrders() // [Order]
const order = await qt.getOrder(orderId) // => Order
await qt.createOrder(newOrder)
await qt.updateOrder(orderId, newOrder)
await qt.removeOrder(orderId)
await qt.testOrder(order)
const symbol = await qt.getSymbol('MSFT') // => Symbol
const symbols = await qt.getSymbols(['MSFT', 'AAPL', 'BMO']) // => [Symbol]
const symbols = qt.searchSymbols('MS') // => [Symbol]
// Example fetching TSLA options
const tsla = await qt.getSymbol('tsla')
const chain = await tsla.getOptionChain()
const jan25 = chain['2025-01-17']
const jan25600 = jan25['600']
const quote = await jan25600.getQuote()
For those accounts that have L1 data access (either practice account or Advanced market data packages) you can stream live market data.
// ... connect to Questrade first!
// Websocket port changes by API and by symbol. So you have to get the port every time you need different data stream
var getWebSocketURL = function (symbolId, cb) {
var webSocketURL;
request({
method: 'GET',
url: qt.apiUrl + '/markets/quotes?ids=' + symbolId + '&stream=true&mode=WebSocket',
auth: {
bearer: qt.accessToken
}
}, function (err, http, body) {
if (err) {
cb(err, null);
} else {
response = JSON.parse(body);
webSocketURL = qt.api_server.slice(0, -1) + ':' + response.streamPort + '/' + qt.apiVersion + '/markets/quotes?ids=' + symbolId + 'stream=true&mode=WebSocket';
cb(null, webSocketURL);
}
});
}
getWebSocketURL('9291,8049', function (err, webSocketURL) { // BMO.TO & AAPL
console.log(webSocketURL);
const WebSocket = require('ws');
const ws = new WebSocket(webSocketURL);
ws.on('open', function open() {
ws.send(qt.accessToken);
});
ws.on('message', function incoming(data) {
console.log(data);
// Do what you want with the data
});
// CLOSING WebSocket Connections otherwise will remain open
process.on('exit', function () {
if (ws) {
console.log('CLOSE WebSocket');
ws.close();
}
});
//catches ctrl+c event
process.on('SIGINT', function () {
if (ws) {
console.log('CLOSE WebSocket SIGINT');
ws.close();
}
});
//catches uncaught exceptions
process.on('uncaughtException', function () {
if (ws) {
console.log('CLOSE WebSocket');
ws.close();
}
});
});
false
v1
connect
, you can use an access token directly, perhaps through an implicit OAuth flow.Are welcome!
FAQs
Questrade API
The npm package questrade receives a total of 4 weekly downloads. As such, questrade popularity was classified as not popular.
We found that questrade demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.