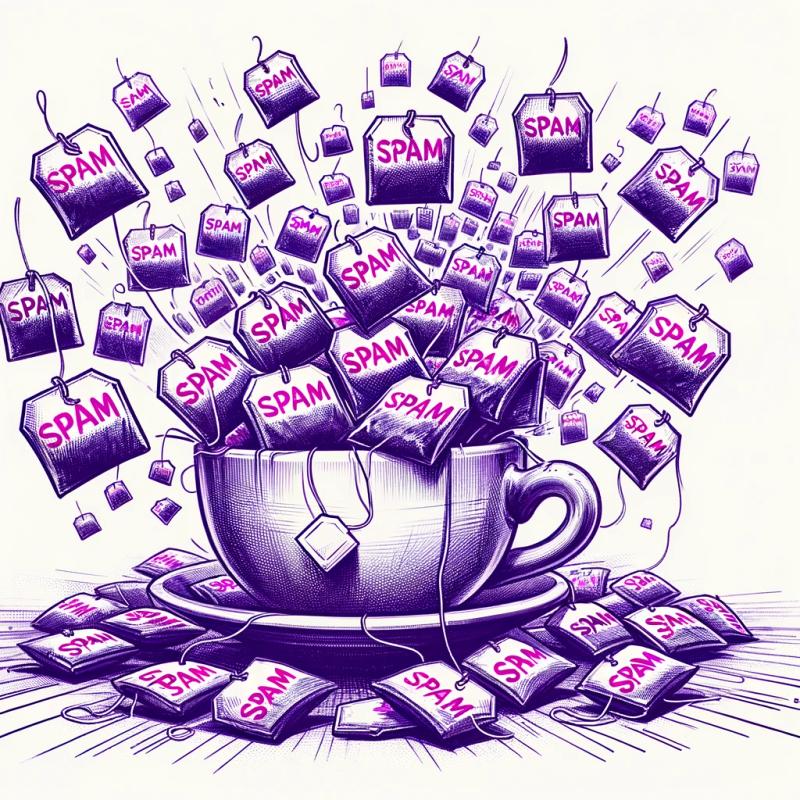
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ramblechat-api
Advanced tools
Readme
The RambleChat API gives anyone the ability to create a chat experience for their visitors/customers/users beyond what is provided by RambleChat.
These instructions are intended to get you started by creating a demo node application that can interact directly with your RambleChat account.
You'll need to install NodeJS if you haven't already.
The example below was written using es6 style modules and imports along with
const
and let
. Check Node Green to find out what
features your version of Node supports. Only a few sections of codes need to
be ammended to be supported with ES5.
npm install ramblechat-api --save
You'll also want socket.io-client:
npm install socket.io-client --save
If you want to run the tests for this project you'll need to install the dev dependencies as well followed by:
npm run test
This will get you connected to RambleChat and ready to start sending and receiving events.
const io = require('socket.io-client');
const CONSTANTS = require('ramblechat-api/visitor/constants');
const { BACKEND_REALTIME_EVENT,
REALTIME_ACTION_REQUEST,
VISITOR_REALTIME_EVENT } = require('ramblechat-api');
const { API_VERSION } = require('ramblechat-api/visitor');
const socket = io('https://realtime.ramblechat.com:8443/visitor', {
reconnection: false,
query: {
apiVersion: API_VERSION,
code: '', // or your team code
}
});
This will send a request to chat to your RambleChat dashboard. If a member of your team is logged in and available they'll be prompted to accept a chat.
const targetTeam = '<the team you want to connect with>';
socket.on("connect", () => {
socket.on(BACKEND_REALTIME_EVENT, (action) => console.log(action));
const startChat = {
type: CONSTANTS.VISITOR_CREATE_CHAT,
flowType: REALTIME_ACTION_REQUEST,
meta: {
realtime: true,
authenticated: false,
},
payload: {
code: targetTeam,
email: '[<visitors email address>] - OPTIONAL'
}
};
socket.emit(VISITOR_REALTIME_EVENT, startChat, (response) => {
console.log(response.payload);
});
});
You may want to know ahead of time if anyone is available to chat. If you're making a custom visitor client or workflow you'll want to use this event type for telling your users whether or not anyone is available. Probably not a great experience if it just sits there, right?
const targetTeam = '<the team you want to connect with>';
socket.on("connect", () => {
socket.on(BACKEND_REALTIME_EVENT, (action) => console.log(action));
const requestInfo = {
type: CONSTANTS.VISITOR_GET_TEAM_INFO,
flowTppe: REALTIME_ACTION_REQUEST,
meta: {
realtime: true
},
payload: {
code: targetTeam
}
};
socket.emit(VISITOR_REALTIME_EVENT, requestInfo, (response) => {
console.log(response.payload);
});
});
More documentation will be made availabe via gitbook in the future.
FAQs
RambleChat API for custom client applications
The npm package ramblechat-api receives a total of 0 weekly downloads. As such, ramblechat-api popularity was classified as not popular.
We found that ramblechat-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.