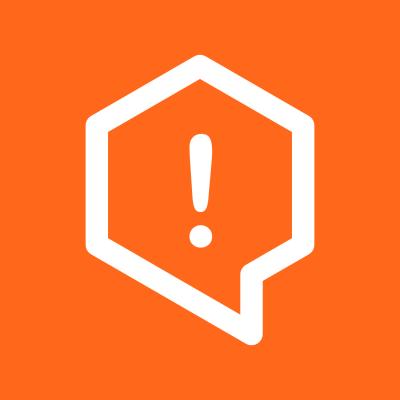
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
random-bytes
Advanced tools
The 'random-bytes' npm package is used to generate cryptographically strong pseudo-random bytes. It is useful for creating secure tokens, unique identifiers, and other random data needed in various applications.
Generate Random Bytes
This feature allows you to generate a specified number of random bytes. In this example, 16 random bytes are generated and converted to a hexadecimal string.
const randomBytes = require('random-bytes');
randomBytes(16, (err, buffer) => {
if (err) throw err;
console.log(buffer.toString('hex'));
});
Generate Random Bytes Synchronously
This feature allows you to generate random bytes synchronously. In this example, 16 random bytes are generated synchronously and converted to a hexadecimal string.
const randomBytes = require('random-bytes');
try {
const buffer = randomBytes.sync(16);
console.log(buffer.toString('hex'));
} catch (err) {
console.error(err);
}
The 'crypto' module is a built-in Node.js module that provides cryptographic functionality, including a method to generate random bytes. It is more comprehensive than 'random-bytes' and includes various cryptographic operations such as hashing, encryption, and decryption.
The 'uuid' package is used to generate RFC-compliant UUIDs (Universally Unique Identifiers). While it does not generate random bytes directly, it uses random data to create unique identifiers, making it useful for similar purposes like generating unique tokens.
The 'secure-random' package generates cryptographically strong random numbers and bytes. It offers similar functionality to 'random-bytes' but also includes additional features like generating random numbers within a specified range.
Generate strong pseudo-random bytes.
This module is a simple wrapper around the Node.js core crypto.randomBytes
API,
with the following additions:
Promise
interface for environments with promises.$ npm install random-bytes
var randomBytes = require('random-bytes')
Generates strong pseudo-random bytes. The size
argument is a number indicating
the number of bytes to generate.
randomBytes(12, function (error, bytes) {
if (error) throw error
// do something with the bytes
})
Generates strong pseudo-random bytes and return a Promise
. The size
argument is
a number indicating the number of bytes to generate.
Note: To use promises in Node.js prior to 0.12, promises must be
"polyfilled" using global.Promise = require('bluebird')
.
randomBytes(18).then(function (string) {
// do something with the string
})
A synchronous version of above.
var bytes = randomBytes.sync(18)
FAQs
URL and cookie safe UIDs
The npm package random-bytes receives a total of 2,190,980 weekly downloads. As such, random-bytes popularity was classified as popular.
We found that random-bytes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.