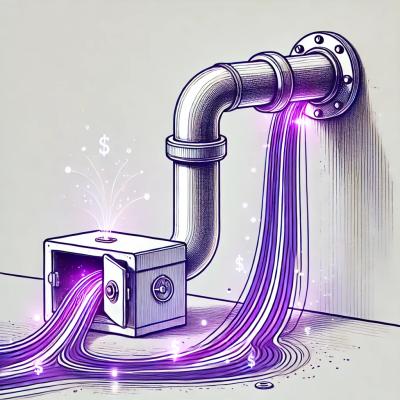
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
A tiny library which provides utility types/functions for request and response query handling.
Rapiq (Rest Api Query) is a library to build an efficient interface between client- & server-side applications. It defines a scheme for the request, but not for the response.
Table of Contents
npm install rapiq --save
To read the docs, visit https://rapiq.tada5hi.net
fields
filters
relations
pagination
sort
It is based on the JSON-API specification.
This is a small outlook on how to use the library. For detailed explanations and extended examples, read the docs.
The first step is to construct a BuildInput object for a generic Record <T>
.
Pass the object to the buildQuery method to convert it to a transportable string.
The BuildInput<T>
can contain a configuration for each
Parameter/
URLParameter.
FieldsBuildInput<T>
FiltersBuildInput<T>
PaginationBuildInput<T>
RelationsBuildInput<T>
SortBuildInput<T>
NOTE: Check out the API-Reference of each parameter for acceptable input formats and examples.
After building, the string can be passed to a backend application as http query string argument. The backend application can process the request, by parsing the query string.
The following example is based on the assumption, that the following packages are installed:
It should give an insight on how to use this library.
Therefore, a type which will represent a User
and a method getAPIUsers
are defined.
The method should perform a request to the resource API to receive a collection of entities.
import axios from "axios";
import {
buildQuery,
BuildInput
} from "rapiq";
export type Realm = {
id: string,
name: string,
description: string,
}
export type Item = {
id: string,
realm: Realm,
user: User
}
export type User = {
id: number,
name: string,
email: string,
age: number,
realm: Realm,
items: Item[]
}
type ResponsePayload = {
data: User[],
meta: {
limit: number,
offset: number,
total: number
}
}
const record: BuildInput<User> = {
pagination: {
limit: 20,
offset: 10
},
filters: {
id: 1
},
fields: ['id', 'name'],
sort: '-id',
relations: ['realm']
};
const query = buildQuery(record);
// console.log(query);
// ?filter[id]=1&fields=id,name&page[limit]=20&page[offset]=10&sort=-id&include=realm
async function getAPIUsers(
record: BuildInput<User>
): Promise<ResponsePayload> {
const response = await axios.get('users' + buildQuery(record));
return response.data;
}
(async () => {
let response = await getAPIUsers(record);
// do something with the response :)
})();
The next section will describe, how to parse the query string on the backend side.
The last step of the whole process is to parse the transpiled query string, to an efficient data structure.
The result object (ParseOutput
) can contain an output for each
Parameter/
URLParameter.
FieldsParseOutput<T>
FiltersParseOutput<T>
PaginationParseOutput<T>
RelationsParseOutput<T>
SortParseOutput<T>
NOTE: Check out the API-Reference of each parameter for output formats and examples.
The following example is based on the assumption, that the following packages are installed:
For explanation purposes, three simple entities with relations between them are declared to demonstrate the usage on the backend side.
entities.ts
import {
Entity,
PrimaryGeneratedColumn,
Column,
OneToMany,
JoinColumn,
ManyToOne
} from "typeorm";
@Entity()
export class User {
@PrimaryGeneratedColumn({unsigned: true})
id: number;
@Column({type: 'varchar', length: 30})
@Index({unique: true})
name: string;
@Column({type: 'varchar', length: 255, default: null, nullable: true})
email: string;
@Column({type: 'int', nullable: true})
age: number
@ManyToOne(() => Realm, { onDelete: 'CASCADE' })
realm: Realm;
@OneToMany(() => User, { onDelete: 'CASCADE' })
items: Item[];
}
@Entity()
export class Realm {
@PrimaryColumn({ type: 'varchar', length: 36 })
id: string;
@Column({ type: 'varchar', length: 128, unique: true })
name: string;
@Column({ type: 'text', nullable: true, default: null })
description: string | null;
}
@Entity()
export class Item {
@PrimaryGeneratedColumn({unsigned: true})
id: number;
@ManyToOne(() => Realm, { onDelete: 'CASCADE' })
realm: Realm;
@ManyToOne(() => User, { onDelete: 'CASCADE' })
user: User;
}
import { Request, Response } from 'express';
import {
applyQuery,
useDataSource
} from 'typeorm-extension';
/**
* Get many users.
*
* Request example
* - url: /users?page[limit]=10&page[offset]=0&include=realm&filter[id]=1&fields=id,name
*
* @param req
* @param res
*/
export async function getUsers(req: Request, res: Response) {
const dataSource = await useDataSource();
const repository = dataSource.getRepository(User);
const query = repository.createQueryBuilder('user');
// -----------------------------------------------------
// parse and apply data on the db query.
const { pagination } = applyQuery(query, req.query, {
defaultPath: 'user',
fields: {
allowed: ['id', 'name', 'realm.id', 'realm.name'],
},
filters: {
allowed: ['id', 'name', 'realm.id'],
},
relations: {
allowed: ['items', 'realm']
},
pagination: {
maxLimit: 20
},
sort: {
allowed: ['id', 'name', 'realm.id'],
}
});
// -----------------------------------------------------
const [entities, total] = await query.getManyAndCount();
return res.json({
data: {
data: entities,
meta: {
total,
...pagination
}
}
});
}
Made with 💚
Published under MIT License.
FAQs
A tiny library which provides utility types/functions for request and response query handling.
The npm package rapiq receives a total of 56,702 weekly downloads. As such, rapiq popularity was classified as popular.
We found that rapiq demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.