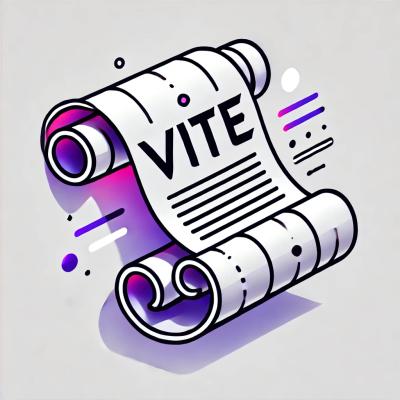
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
raptor-args
Advanced tools
A flexible and simple command line arguments parser that generates friendly help messages.
npm install raptor-args --save
// Create a parser:
var parser = require('raptor-args').createParser(options);
var parsed = parser.parse(argsArray);
// parsed will be an object with properties corresponding to provided arguments
Parse arguments provided by process.argv
:
Given the following JavaScript code to parse the args:
// Create a parser and parse process.argv
require('raptor-args').createParser({
'--foo -f': 'boolean',
'--bar -b': 'string'
})
.parse();
And the following command:
node app.js --foo -b b
The output will be:
//Output:
{
foo: true,
bar: 'baz'
}
You can also parse your own array of arguments instead of using process.argv
:
// Create a parser and parse provided args
require('raptor-args').createParser({
'--foo -f': 'boolean',
'--bar -b': 'string'
})
.parse(['--foo', '-b', 'baz']);
//Output:
{
foo: true,
bar: 'baz'
}
You can also be more descriptive and add usage, examples, error handlers and validation checks:
// Create a parser:
require('raptor-args')
.createParser({
'--help': {
type: 'string',
description: 'Show this help message'
},
'--foo -f': {
type: 'string',
description: 'Some helpful description for "foo"'
},
'--bar -b': {
type: 'string',
description: 'Some helpful description for "bar"'
}
})
.usage('Usage: $0 [options]')
.example(
'First example',
'$0 --foo hello')
.example(
'Second example',
'$0 --foo hello --bar world')
.validate(function(result) {
if (result.help) {
this.printUsage();
process.exit(0);
}
if (!result.foo || !result.bar) {
this.printUsage();
console.log('--foo or --bar is required');
process.exit(1);
}
})
.onError(function(err) {
this.printUsage();
console.error(err);
process.exit(1);
})
.parse();
Running the above program with the --help
argument will produce the following output:
Usage: args [options]
Examples:
First example:
args --foo hello
Second example:
args --foo hello --bar world
Options:
--help Show this help message [string]
--foo -f Some helpful description for "foo" [string]
--bar -b Some helpful description for "bar" [string]
Aliases can be provided as space-separated values for an option:
// Create a parser:
var parser = require('raptor-args').createParser({
'--foobar --foo -f': 'string', // "--foobar" has two aliases: "--foo" and "-f"
'--hello -h': 'string', // "--hello" has one alias: "-h"
});
parser.parse('--foo FOO -h HELLO'.split(' '));
// Output:
{
foobar: 'FOO',
hello: 'HELLO'
}
// **NOTE**: Only the first entry is used to determine the target property name--not the aliases.
An argument value of "true" or "false" is automatically converted to the corresponding boolean type. If a argument is prefixed with "no-" then it will be set to false
.
// Create a parser:
var parser = require('raptor-args').createParser({
'--foo': 'boolean',
'--bar': 'boolean'
});
parser.parse('--foo --no-bar'.split(' '));
// Output:
{
foo: true,
bar: false
}
Any argument with multiple values will result in an Array
value, but if you want to force an array for a single value then you can append "[]" to the option type as shown in the following sample code:
// Create a parser:
var parser = require('raptor-args').createParser({
'--foo': 'string[]'
});
parser.parse('--foo a'.split(' '));
// Output:
{
foo: ['a']
}
parser.parse('--foo a b c'.split(' '));
// Output:
{
foo: ['a', 'b', 'c']
}
A parser will throw an error for unrecognized arguments unless wildcards are used as shown in the examples below.
// Create a parser:
var parser = require('raptor-args').createParser({
'--foo -f *': 'string[]' // Any unrecognized argument at the beginning is an alias for "foo"
});
parser.parse('a b --foo c'.split(' '));
// Output:
{
foo: ['a', 'b', 'c']
}
// Create a parser:
var parser = require('raptor-args').createParser({
'*': null
});
parser.parse('a b --foo FOO --bar BAR'.split(' '));
// Output:
{
'*': ['a', 'b'],
foo: 'FOO',
bar: 'BAR'
}
Square brackets can be used to begin and end complex types:
// Create a parser:
var parser = require('raptor-args').createParser({
'--foo -f': 'boolean',
'--plugins --plugin -p': {
options: {
'--module -m *': 'string',
'-*': null
}
}
});
var parsed = parser.parse('--foo --plugins [ --module plugin1 -x -y ] [ plugin2 -z Hello ]'.split(' '));
// Output:
{
foo: true,
plugins: [
{
module: 'plugin1',
x: true,
y: true
},
{
module: 'plugin2',
z: 'Hello'
}
]
}
optimist:
var result = require('optimist')(args)
.alias('h', 'help')
.describe('h', 'Show this help message')
.boolean('h')
.alias('f', 'foo')
.describe('f', 'Some helpful description for "foo"')
.string('f')
.alias('b', 'bar')
.describe('b', 'Some helpful description for "bar"')
.string('b')
.argv;
raptor-args:
var result = require('raptor-args')
.createParser({
'--help': { type: 'string', description: 'Show this help message' },
'--foo -f': { type: 'string', description: 'Some helpful description for "foo"' },
'--bar -b': { type: 'string', description: 'Some helpful description for "bar"' }
})
.parse();
optimist
with documentation for those who speak Pirate.--hello=world
-x256
var parser = require('../')
.createParser({
'--foo -f': {
type: 'boolean',
defaultValue: true
}
});
For module help, check out the test cases under the "test" directory.
MIT
FAQs
A concise command line arguments parser with robust type handling
We found that raptor-args demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.