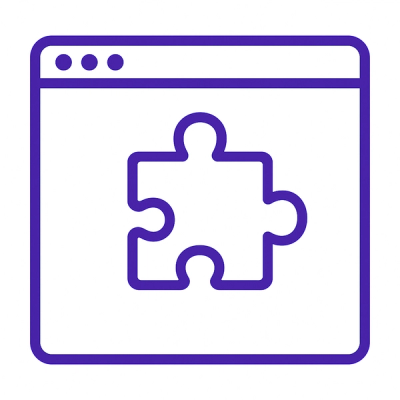
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
react-ace
Advanced tools
The react-ace package is a React component for the Ace editor, which is a powerful code editor written in JavaScript. It allows you to embed the Ace editor into your React applications, providing a rich set of features for code editing, syntax highlighting, and more.
Basic Usage
This code demonstrates the basic usage of the react-ace package. It imports the necessary modules and sets up an Ace editor with JavaScript mode and the GitHub theme.
import React from 'react';
import AceEditor from 'react-ace';
import 'ace-builds/src-noconflict/mode-javascript';
import 'ace-builds/src-noconflict/theme-github';
function MyEditor() {
return (
<AceEditor
mode="javascript"
theme="github"
name="editor"
editorProps={{ $blockScrolling: true }}
/>
);
}
export default MyEditor;
Customizing Editor Options
This code sample shows how to customize various options of the Ace editor, such as font size, print margin, gutter, active line highlighting, and enabling autocompletion and snippets.
import React from 'react';
import AceEditor from 'react-ace';
import 'ace-builds/src-noconflict/mode-javascript';
import 'ace-builds/src-noconflict/theme-github';
function MyEditor() {
return (
<AceEditor
mode="javascript"
theme="github"
name="editor"
fontSize={14}
showPrintMargin={true}
showGutter={true}
highlightActiveLine={true}
setOptions={{
enableBasicAutocompletion: true,
enableLiveAutocompletion: true,
enableSnippets: true,
showLineNumbers: true,
tabSize: 2,
}}
/>
);
}
export default MyEditor;
Handling Editor Events
This example demonstrates how to handle events in the Ace editor. The onChange event is used to log the new value of the editor whenever it changes.
import React from 'react';
import AceEditor from 'react-ace';
import 'ace-builds/src-noconflict/mode-javascript';
import 'ace-builds/src-noconflict/theme-github';
function MyEditor() {
const onChange = (newValue) => {
console.log('change', newValue);
};
return (
<AceEditor
mode="javascript"
theme="github"
name="editor"
onChange={onChange}
/>
);
}
export default MyEditor;
The react-codemirror2 package is a React component for the CodeMirror editor. It provides similar functionalities to react-ace, such as syntax highlighting, code folding, and customizable themes. However, CodeMirror is known for its flexibility and extensive list of supported languages and modes.
The react-monaco-editor package is a React component for the Monaco editor, which is the code editor that powers Visual Studio Code. It offers advanced features like IntelliSense, parameter hints, and a rich API for extensions. Compared to react-ace, react-monaco-editor provides a more feature-rich and modern editing experience.
The react-simple-code-editor package is a lightweight code editor component for React. It is built on top of the Prism syntax highlighter and is designed to be simple and easy to use. While it lacks some of the advanced features of react-ace, it is a good choice for basic code editing needs.
A set of react components for Ace
NOTE FOR VERSION 8! : We have stopped support for Brace and now use Ace-builds. Please read the documentation on how to migrate. Examples are being updated.
DEMO of React Ace Split Editor
npm install react-ace ace-builds
yarn add react-ace ace-builds
import React from "react";
import { render } from "react-dom";
import AceEditor from "react-ace";
import "ace-builds/src-noconflict/mode-java";
import "ace-builds/src-noconflict/theme-github";
import "ace-builds/src-noconflict/ext-language_tools";
function onChange(newValue) {
console.log("change", newValue);
}
// Render editor
render(
<AceEditor
mode="java"
theme="github"
onChange={onChange}
name="UNIQUE_ID_OF_DIV"
editorProps={{ $blockScrolling: true }}
/>,
document.getElementById("example")
);
Checkout the example
directory for a working example using webpack.
How to add modes, themes and keyboard handlers
Support us with a monthly donation and help us continue our activities. [Become a backer]
Become a sponsor and get your logo on our README on Github with a link to your site. [Become a sponsor]
FAQs
A react component for Ace Editor
The npm package react-ace receives a total of 530,488 weekly downloads. As such, react-ace popularity was classified as popular.
We found that react-ace demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.