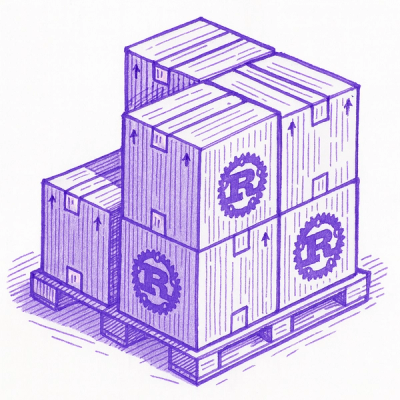
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
react-adopt
Advanced tools
[Render Props](https://reactjs.org/docs/render-props.html) are the new hype of React's ecossystem, that's a fact. So, when you need to use more than one render props component together, this can be borring and generate something like a *"render hell"*.
:sunglasses: React Adop - Compose render props components like a pro
Render Props are the new hype of React's ecossystem, that's a fact. So, when you need to use more than one render props component together, this can be borring and generate something like a "render hell".
React Adopt it's just a simple method that you can compose your components and return all props in a function by mapping each child prop returned by your component.
Install as project dependency:
$ yarn add react-adopt
Then you can use the method to compose your components
import React from 'react'
import { render } from 'react-dom'
import { adopt } from 'react-adopt'
import { Filter } from './my-awesome-filter-component'
const Composed = adopt({
kids: <Filter list={people} by={p => p.age < 18} />,
adults: <Filter list={people} by={p => p.age >= 18} />
})
const App = () => (
<Composed>
{({ kids, adults }) => (
<div>
<ul>{kids.map(p => <li>{p.name}</li>)}</ul>
<ul>{adults.map(p => <li>{p.name}</li>)}</ul>
</div>
)}
</Composed>
)
FAQs
- [Why](#--why) - [Solution](#--solution) - [Demos](#--demos) - [Usage](#--usage-demo) - [Working with new Context api](#working-with-new-context-api) - [Custom render and retrieving props from composed](#custom-render-and-retrieving-props-from-composed)
The npm package react-adopt receives a total of 5,794 weekly downloads. As such, react-adopt popularity was classified as popular.
We found that react-adopt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.