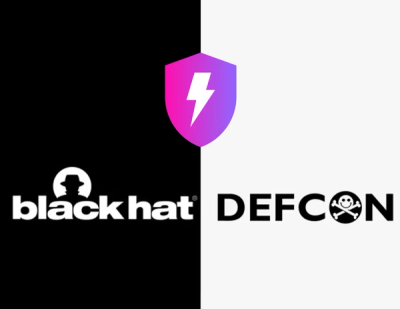
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
react-bootstrap-selectbox
Advanced tools
A wrapper for react-select
npm install --save react-bootstrap-selectbox
import React, { useState } from 'react'
import { SelectBox } from 'react-bootstrap-selectbox'
import 'react-bootstrap-selectbox/dist/index.css'
const SelectBoxExample = (props) => {
const companies = [
{ company_name: "Facebook", company_id: "0" },
{ company_name: "Google", company_id: "1" }
]
const [company, setCompany] = useState(null);
const handleSelection = (data, event) => {
console.log(event.name) //company
console.log(data) // { label: "Google", value: "1" }
setCompany(data)
}
return (
<SelectBox
name="company"
onSelection={handleSelection}
options={companies}
bindLabel="company_name" // pass your label key default key label
bindValue="company_id" // pass your value key default key value
placeholder="Select Company" // default Select... - optional
value={company} // default null
label="Company" // default '' - optional
/>
)
}
export default SelectBoxExample;
import React, { useState } from 'react'
import { SelectBox } from 'react-bootstrap-selectbox'
import 'react-bootstrap-selectbox/dist/index.css'
const SelectBoxExample2 = (props) => {
const companies = [
{ label: "Facebook", value: "0" },
{ label: "Google", value: "1" }
]
const [company, setCompany] = useState(null);
const handleSelection = (data, event) => {
console.log(event.name) //company
console.log(data) // { label: "Google", value: "1" }
setCompany(data)
}
return (
<SelectBox
name="company"
onSelection={handleSelection}
options={companies}
placeholder="Select Company"
value={company}
label="Company"
/>
)
}
export default SelectBoxExample2;
isSearchable - true (default)
isClearable - true (default)
isMulti - false (default) // for multiple selection
FAQs
A wrapper for react-select
The npm package react-bootstrap-selectbox receives a total of 0 weekly downloads. As such, react-bootstrap-selectbox popularity was classified as not popular.
We found that react-bootstrap-selectbox demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.