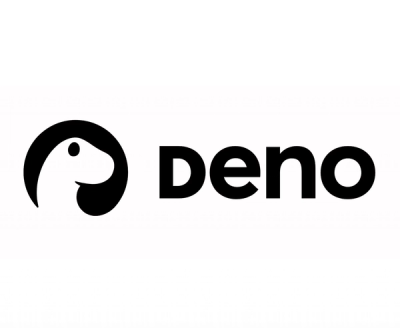
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
react-client-async
Advanced tools
Previously, async component
were only supported on the server (Next.js). With this package, you can now easily use it on the client side as well.
npm i react-client-async
useAsync
HookYou can use the useAsync
hook to create a task.
console.log(useAsync(fn, args, options));
Async
ComponentYou can use the Async
component to render an async component.
<Async
$fc={fc} // may be an async function component
$waiting={waiting} // waiting component
$fallback={fallback} // fallback component
{...props} // props for the function component
/>
Demo
of Recursive Async ComponentA component can be used with memo
and async
together!
const Rec: AsyncFC<{ n: number; }> = memo(
async ({ [$signal]: signal, n }) =>
n <= 0 ? (
<> {/* break the recursion */}
<div>0</div>
</>
) : (
<> {/* delay and recursion */}
{await delayWithSignal(99, signal)}
{n}<Async $fc={Rec} n={n - 1} />{n}
</>
),
);
useAsyncIterable
hookIterable async
componentasync function* IterableComponent() {
yield* OtherIterableComponent();
yield await component1();
yield await component2();
yield <div>...</div>;
}
Looking forward to your feedback or contribution! 🚀🚀🚀
bun
runtime: npm install -g bun
bun install
bun dev
bun run build:app
bun run build:lib
bun run deploy
bun publish
FAQs
React tools for async rendering in client side! 🚀
The npm package react-client-async receives a total of 9 weekly downloads. As such, react-client-async popularity was classified as not popular.
We found that react-client-async demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.