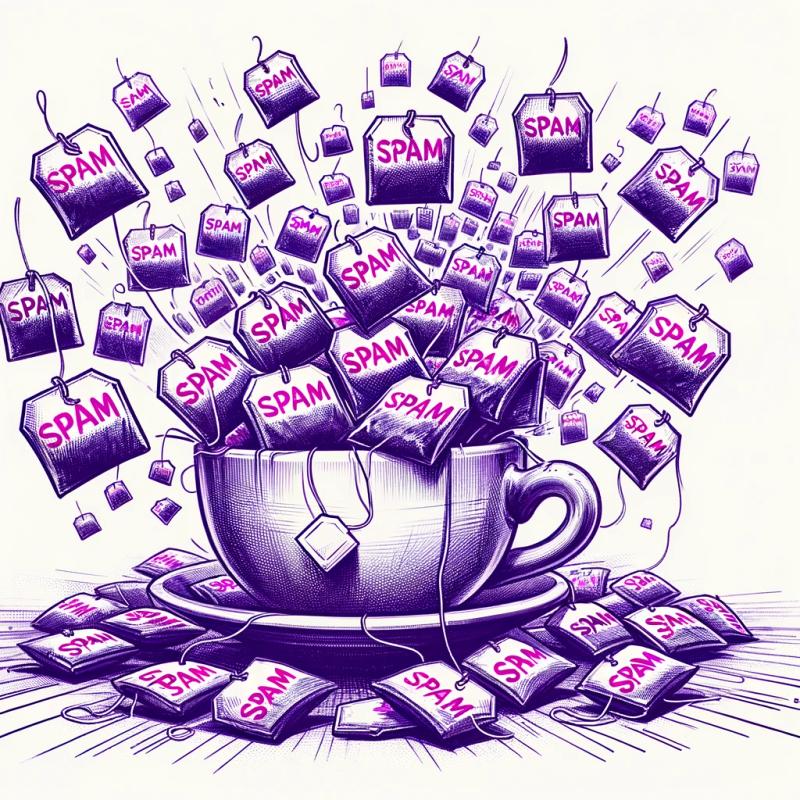
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-cool-draggable
Advanced tools
Drag-n-drop react library (component) for horizontal and vertical lists.
Readme
Drag-n-drop react library (component) for horizontal and vertical lists.
One common frontend task is to create draggable cards in various lists. To simplify this task, I wrote a library that can work with horizontal and vertical lists, as well as their combinations (for example, as done in Trello).
npm:
npm install react-cool-draggable
yarn:
yarn add react-cool-draggable
import {
DragDropContext,
Droppable,
Draggable,
reorder,
} from 'react-cool-draggable';
const handleDragEnd = options => {
const newItems = reorder({
...options,
items,
getDraggableID: x => x.ID,
getDroppableID: () => 'row',
});
setItems(newItems);
};
<DragDropContext onDragEnd={handleDragEnd}>
<Droppable
direction='vertical'
droppableID='row'
droppableGroupID='board'>
{({ snapshot, ...rest }) => (
<div className='droppable' {...rest}>
{items.map(x => (
<Draggable key={x.ID} draggableID={x.ID}>
{({ snapshot, rootProps, draggableProps }) => (
<div className='draggable' {...rootProps} {...draggableProps}>
{x.text}
</div>
)}
</Draggable>
))}
</div>
)}
</Droppable>
</DragDropContext>
You can also combine and nest droppable and draggable elements to create more complex components if you need to.
Some universal types here
type ID = string | number;
type Direction = 'vertical' | 'horizontal';
type DraggableElement = HTMLElement | SVGElement;
This library uses the react context as a way to communicate between children in the tree, so the context must be explicitly invoked at the root of the component.
import { DragDropContext } from 'react-cool-draggable';
<DragDropContext onDragEnd={handleDragEnd}>
Some children here...
</DragDropContext>
type DragDropContextProps = {
onDragStart?: (options: OnDragStartOptions) => void;
onDragOver?: (options: OnDragOverOptions) => void;
onDragEnd: (options: OnDragEndOptions) => void;
children: React.ReactElement;
};
All of these callback accept a number of options that may be useful to you later.
type OnDragStartOptions = {
draggableID: ID;
droppableID: ID;
droppableGroupID: ID;
targetNode: DraggableElement;
};
type OnDragOverOptions = {
draggableID: ID;
droppableID: ID;
droppableGroupID: ID;
targetNode: DraggableElement;
nearestNode: DraggableElement | null;
};
type OnDragEndOptions = {
draggableID: ID;
droppableID: ID;
droppableGroupID: ID;
sourceIdx: number;
destinationIdx: number;
isMoving: boolean;
targetNode: DraggableElement;
};
Think of this component as a surface on which elements move. It is simple logic where all the logic is hidden. At the same time, it is based on the concept of RenderProps and expects to receive a render function as children.
import { Droppable } from 'react-cool-draggable';
<Droppable
direction='vertical'
droppableID='row'
droppableGroupID='board'>
{({ snapshot, ...rest }) => <div {...rest}>Some children here...</div>}
</Droppable>
type DroppableProps = {
direction: Direction;
droppableID: ID;
droppableGroupID: ID;
transitionTimeout?: number;
transitionTimingFn?: string;
disabled?: boolean;
debounceTimeout?: number;
children: (options: DroppableChildrenOptions) => React.ReactElement;
};
The direction - the main property that specifies how the component should deal with the drag direction. You will also need to provide some identifiers so that you can distinguish one surface from another.
type DroppableChildrenOptions = {
snapshot: {
isDragging: boolean;
};
...some service options
};
The snapshot can be useful for you to understand and react to real-time dragging, such as changing the surface color to let the user know that the dragging mode has been activated.
You can think of this component as an element that is draggable by the user. And it is also just a component that implements logic without any external appearance. So he also needs a render function to tell it what it should look like.
import { Draggable } from 'react-cool-draggable';
<Draggable draggableID={x.ID}>
{({ snapshot, rootProps, draggableProps }) =>
<div {...rootProps} {...draggableProps}>Some children here...</div>}
</Draggable>
type DraggableProps = {
draggableID: ID;
children: (options: DraggableChildrenOptions) => React.ReactElement;
};
type DraggableChildrenOptions = {
snapshot: {
isDragging: boolean;
};
rootProps: SomeServiceOptions;
draggableProps: SomeServiceOptions;
};
In the render function declaration, you must pass rootProps and draggableProps to the element. At the same time, if you want to make the draggable activation not be carried out on the entire element, but only on its part (for example, a button that needs to be captured in order to drag), then you must pass draggableProps to this button and pass only rootProps to the element itself.
import { reorder, move } from 'react-cool-draggable';
These are the functions you need to apply inside the onDragEnd callback to sort the list of items in the component's state correctly. In this case, reorder does the usual sorting, and move - moving an element from one group to another, if you implement an exchange of elements between two or more droppable surfaces. This may sound complicated, but it isn't, so please see examples/trello for examples of how to use these functions.
const newItems = reorder({
...options,
items,
getDraggableID: x => x.ID,
getDroppableID: () => 'row',
});
const newItems = move({
...options,
items,
getDraggableID: x => x.ID,
getDroppableID: x => x.groupID,
setDroppableID: (x, droppableID) => {
x.groupID = droppableID;
},
});
MIT © Alex Plex
FAQs
Drag-n-drop react library (component) for horizontal and vertical lists.
The npm package react-cool-draggable receives a total of 1,130 weekly downloads. As such, react-cool-draggable popularity was classified as popular.
We found that react-cool-draggable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.