What is react-email?
The react-email package is designed to help developers create and manage email templates using React components. It allows for the creation of dynamic and reusable email templates with the power of React's component-based architecture.
What are react-email's main functionalities?
Creating Email Templates
This feature allows you to create email templates using React components. The example demonstrates a simple email template with a heading and a paragraph.
const EmailTemplate = () => (
<div>
<h1>Welcome to Our Service</h1>
<p>Thank you for signing up!</p>
</div>
);
Dynamic Content
This feature allows you to include dynamic content in your email templates. The example shows how to pass a user's name as a prop to personalize the email.
const EmailTemplate = ({ userName }) => (
<div>
<h1>Welcome, {userName}!</h1>
<p>We're glad to have you with us.</p>
</div>
);
Styling Emails
This feature allows you to style your email templates using inline styles. The example demonstrates how to apply styles to the email content.
const EmailTemplate = () => (
<div style={{ fontFamily: 'Arial, sans-serif', color: '#333' }}>
<h1 style={{ color: '#007BFF' }}>Welcome to Our Service</h1>
<p>Thank you for signing up!</p>
</div>
);
Other packages similar to react-email
mjml-react
mjml-react is a package that allows you to use MJML components in React to create responsive email templates. It provides a higher-level abstraction for creating emails, focusing on responsiveness and compatibility across email clients. Compared to react-email, mjml-react is more specialized in ensuring that emails look good on all devices and email clients.
react-html-email
react-html-email is a package for creating HTML emails using React components. It provides utilities for inline styles and media queries, making it easier to create responsive and visually appealing emails. Compared to react-email, react-html-email offers more built-in utilities for handling email-specific styling challenges.
email-templates
email-templates is a package that allows you to create, preview, and send email templates using various templating engines, including React. It provides a more comprehensive solution for managing email templates, including features for previewing and sending emails. Compared to react-email, email-templates offers a broader range of templating options and additional features for email management.
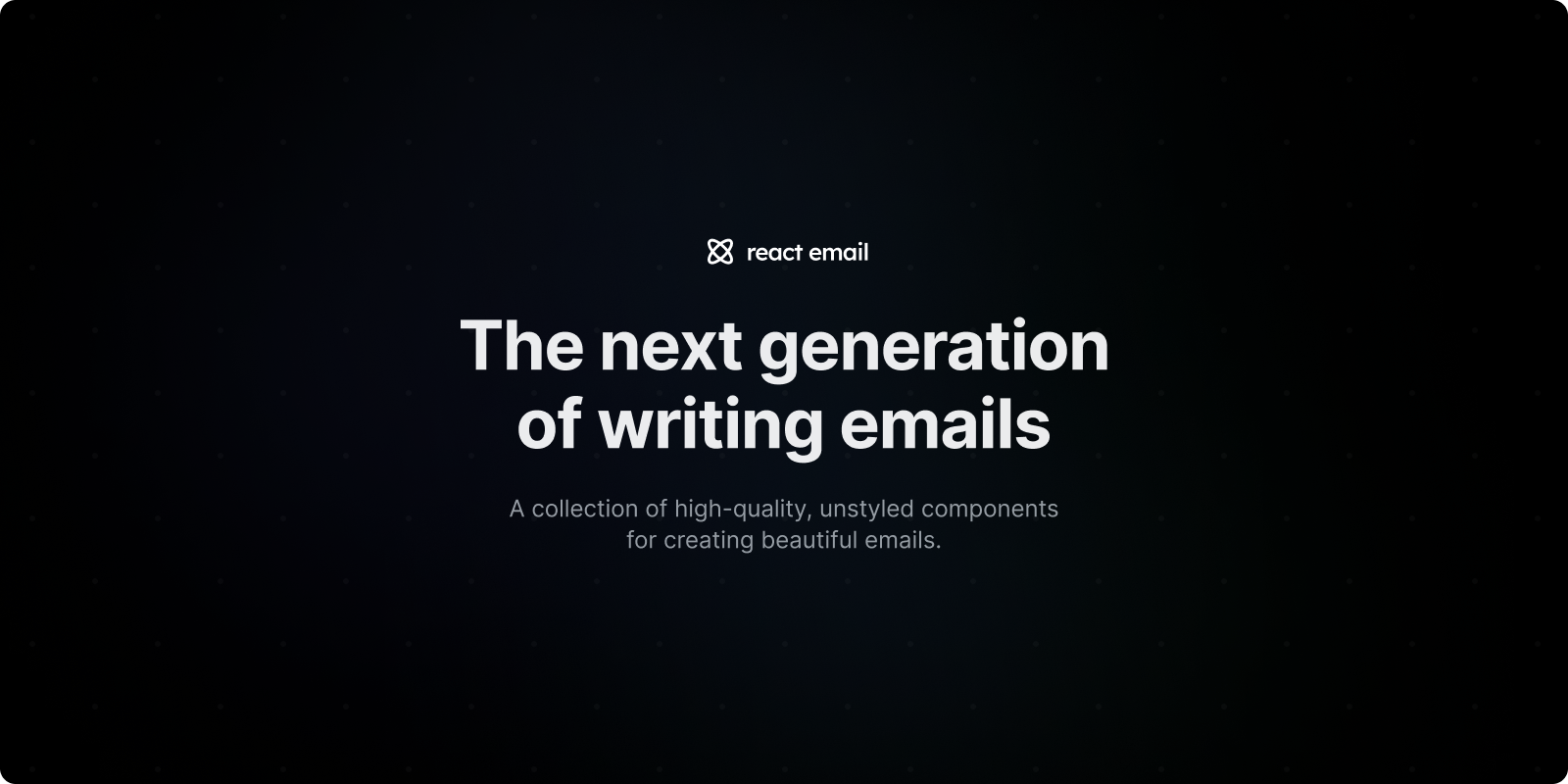
React Email
The next generation of writing emails.
High-quality, unstyled components for creating emails.
Getting started
To get started, open a new shell and run:
npx create-email
This will create a new folder called emails
with a few email templates.
Commands
email dev
Starts a local development server that will watch your files and automatically rebuild your email when you make changes.
npx react-email dev
email export
Generates the plain HTML files of your emails into a out
directory.
npx react-email export
License
MIT License