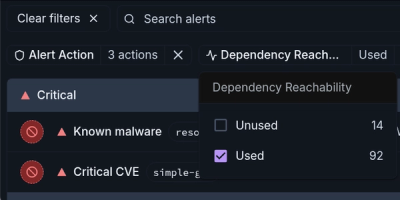
Product
Introducing Module Reachability: Focus on the Vulnerabilities That Matter
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.
react-event-tracker
Advanced tools
npm install --save react-event-tracker
App.js - root level component
import { withSiteTracking } from "react-event-tracker";
function App() {
// Your root level component
}
const trackingConfig = {
// `siteData` can be anything
siteData: {
site: "my site"
},
inject: {
// `trackEvent` will be injected as a prop.
// You can choose any prop name.
trackEvent: ({ siteData, pageData, eventData }) => {
// Do whatever you want with the data.
// For example, call your analytics API.
}
}
};
export default withSiteTracking(App, trackingConfig);
or, you can wrap your root level component with WithSiteTracking
:
import { WithSiteTracking } from "react-event-tracker";
function App() {
return (
<WithSiteTracking {...trackingConfig}>
{/* Your root level component */}
</WithSiteTracking>
);
}
ProductPage.js - page level component
import { withPageTracking } from "react-event-tracker";
function ProductPage() {
return (
...
<ProductPageContent />
...
);
}
// `pageData` can be anything
const pageData = {
page: "my_product"
};
export default withPageTracking(ProductPage, { pageData });
ProductPageContent.js - any component deep inside the tree
import { withEventTracking } from "react-event-tracker";
// The `trackEvent` prop is injected by `withEventTracking` according to the `trackingConfig` above.
function ProductPageContent({ trackEvent }) {
return (
...
<button
onClick={() => {
/*
Here is the core of what this library does.
You call `trackEvent` (provided by `react-event-tracker`) with `eventData` (can be anything).
In return, `react-event-tracker` will call your own `trackEvent` (that you defined in the `trackingConfig` above) with `siteData`, `pageData`, and `eventData`.
*/
trackEvent({ button: "Apply" });
}}
>
Apply
</button>
...
)
}
export default withEventTracking(ProductPageContent);
Add an onPageLoad
function to the trackingConfig
:
const trackingConfig = {
onPageLoad: ({ siteData, pageData, eventData }) => {
// Do whatever you want with the data.
// For example, call your analytics API.
}
}
};
react-event-tracker
will call the onPageLoad
function once your pages (i.e. components wrapped with withPageTracking
) finish rendering.
You could, for example, inspect cookies or localStorage
here, build your data layer, and then call your analytics API.
localStorage
Sometimes, when tracking a page view, you may want to track the traffic source.
For example, say you are tracking page views of the Application page. It could be very useful to know how users have arrived to the Aplication page. Did they click the "Apply" link in the header on the Home page? Maybe the "Apply" link in the footer? Or, maybe, they landed on the Application page after clicking "Apply" on your Product Page?
One way to track this, is to write to localStorage
when users click the "Apply" link. Then, read from localStorage
in the onPageLoad
function.
const trackingConfig = {
storeTrafficSource: ({ pageData, eventData }) => {
localStorage.setItem(
"traffic_source",
`${pageData.page}:${eventData.source}`
);
}
};
import { withEventTracking } from "react-event-tracker";
// The `storeTrafficSource` prop is injected by `withEventTracking` according to the `trackingConfig` above.
function ProductPageContent({ storeTrafficSource }) {
return (
...
{/*
You call `storeTrafficSource` (provided by `react-event-tracker`) with `eventData`.
In return, `react-event-tracker` will call your own `storeTrafficSource` (that you defined in the `trackingConfig` above) with `siteData`, `pageData`, and `eventData`.
*/}
<a
href="/apply"
onClick={() => {
// This will write "my_product:apply" to "traffic_source" in `localStorage`.
storeTrafficSource({ source: "apply" });
}}
>
Apply
</a>
...
)
}
export default withEventTracking(ProductPageContent);
When linking to external sites, you may want to add query string parameters based on siteData
, pageData
, and/or eventData
.
Add a getQueryString
function to inject
, e.g.:
const trackingConfig = {
inject: {
getQueryString: ({ siteData, pageData, eventData }) => {
const dataLayer = {
...siteData,
...pageData,
...eventData
};
return Object.keys(dataLayer)
.map(key => `${key}=${encodeURIComponent(dataLayer[key])}`)
.join("&");
}
}
};
Then, call getQueryString
that is injected as a prop:
import { withEventTracking } from "react-event-tracker";
// The `getQueryString` prop is injected by `withEventTracking` according to the `trackingConfig` above.
function ProductPageContent({ getQueryString }) {
return (
...
{/*
You call `getQueryString` (provided by `react-event-tracker`) with `eventData`.
In return, `react-event-tracker` will call your own `getQueryString` (that you defined in the `trackingConfig` above) with `siteData`, `pageData`, and `eventData`.
*/}
<a
href={`https://external-site.com?${getQueryString({
link: "apply"
})}`}
>
Apply on external site
</a>
...
)
}
export default withEventTracking(ProductPageContent);
FAQs
Easily track events in your React application
The npm package react-event-tracker receives a total of 62 weekly downloads. As such, react-event-tracker popularity was classified as not popular.
We found that react-event-tracker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.
Company News
Socket is bringing best-in-class reachability analysis into the platform — cutting false positives, accelerating triage, and cementing our place as the leader in software supply chain security.
Product
Socket is introducing a new way to organize repositories and apply repository-specific security policies.