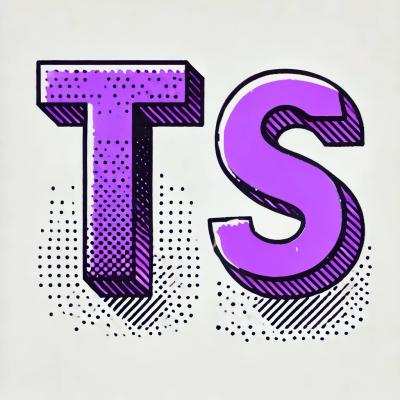
Security News
Node.js Moves Toward Stable TypeScript Support with Amaro 1.0
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
react-fast-hoc
Advanced tools
Lightweight and type-safe High-Order Components (HOCs) library for React, leveraging high-order types from "hotscript" and JavaScript Proxies for zero VDOM overhead and blazing-fast performance.
Supply Chain Security
Vulnerability
Quality
Maintenance
License
Lightweight and type-safe High-Order Components (HOCs) library for React, leveraging high-order types from "hotscript" and JavaScript Proxies for zero VDOM overhead and blazing-fast performance.
Install the react-fast-hoc
package:
# hotscript is a peer dependency
npm i react-fast-hoc && npm i -D hotscript
# or
yarn add react-fast-hoc && yarn add -D hotscript
# or
pnpm i react-fast-hoc && pnpm i -D hotscript
Or with ni:
ni react-fast-hoc && ni -D hotscript
Directly create a new component with transformed props.
import { transformProps } from "react-fast-hoc";
const EnhancedComponent = transformProps(
MyComponent,
(props) => {
// Transform props here
return { ...props, transformedProp: "Transformed Value" };
},
"WithTransformedProps"
);
Create a new HOC with a custom props transformer and optional display name prefix.
import { createHoc } from "react-fast-hoc";
const withCustomLogic = createHoc({
namePrefix: "WithCustomLogic",
propsTransformer: (props) => {
// Apply custom logic here
return { ...props, customProp: "Custom Value" };
},
resultTransformer: null,
nameRewrite: null,
});
const EnhancedComponent = withCustomLogic(MyComponent);
Create a new HOC that transforms the props passed to the wrapped component.
import { createTransformProps } from "react-fast-hoc";
const withTransformedProps = createTransformProps((props) => {
// Transform props here
return { ...props, transformedProp: "Transformed Value" };
}, "WithTransformedProps");
const EnhancedComponent = withTransformedProps(MyComponent);
You can use
wrapIntoProxy
to create more customizable hocs
Detailed API documentation can be found in the API.md file.
You can find example usage of react-fast-hoc
in the examples folder.
React Fast HOC is MIT licensed.
FAQs
Lightweight and type-safe High-Order Components (HOCs) library for React, leveraging high-order types from [`hotscript`](https://github.com/gvergnaud/hotscript) and JavaScript Proxies for zero VDOM overhead.
The npm package react-fast-hoc receives a total of 4,155 weekly downloads. As such, react-fast-hoc popularity was classified as popular.
We found that react-fast-hoc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.