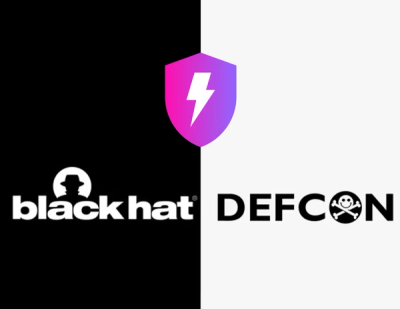
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
react-google-oauth2
Advanced tools
Easily add Google OAuth 2.0 Single Sign On to a React app & let your server handle your access & refresh tokens. This library will work directly with Flask JWT Router & provide Google OAuth 2.0 integration out of the box with minimal setup.
Docs: https://joegasewicz.github.io/react-google-oauth2.0/
npm install react-google-oauth2
import * as React from "react";
import * as ReactDOM from "react-dom";
import { GoogleButton, IAuthorizationOptions } from "../src";
function App(props: any) {
const options: IAuthorizationOptions = {
clientId: (process.env.CLIENT_ID as string),
redirectUri: "http://localhost:3000/react-google-Oauth2.0/dist/index.html",
scopes: ["openid", "profile", "email"],
includeGrantedScopes: true,
accessType: "offline",
};
return (
<>
<GoogleButton
placeholder="demo/search.png" // Optional
options={options}
apiUrl="http://localhost:5000/google_login"
defaultStyle={true} // Optional
/>
</>
);
}
ReactDOM.render(
</App>,
document.getElementById("main"),
);
The GoogleButton
component will make the following request to your api:
POST options = {body: { code: <code>, email: <email>, scope: <scope> }} URL = `apiUrl`
If you are using Flask as your REST api framework then this library is designed to work
directly with flask-jwt-router
. See Flask JWT Router
for more details.
FAQs
React frontend login with OAuth 2.0 & integrates a Rest API backend.
The npm package react-google-oauth2 receives a total of 81 weekly downloads. As such, react-google-oauth2 popularity was classified as not popular.
We found that react-google-oauth2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.