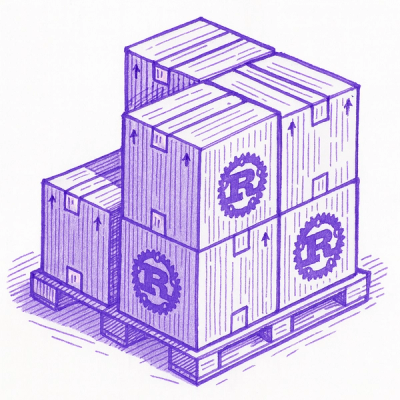
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
react-grid-layout
Advanced tools
A draggable and resizable grid layout with responsive breakpoints, for React.
react-grid-layout is a powerful library for creating dynamic and responsive grid layouts in React applications. It allows developers to create draggable, resizable, and responsive grid layouts with ease.
Draggable Grid Items
This feature allows you to create grid items that can be dragged around within the grid. The layout array defines the position and size of each grid item.
import React from 'react';
import GridLayout from 'react-grid-layout';
const layout = [
{i: 'a', x: 0, y: 0, w: 1, h: 2},
{i: 'b', x: 1, y: 0, w: 3, h: 2},
{i: 'c', x: 4, y: 0, w: 1, h: 2}
];
const MyFirstGrid = () => (
<GridLayout className="layout" layout={layout} cols={12} rowHeight={30} width={1200}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</GridLayout>
);
export default MyFirstGrid;
Resizable Grid Items
This feature allows you to create grid items that can be resized by dragging their edges. The isResizable property in the layout array enables resizing for each grid item.
import React from 'react';
import GridLayout from 'react-grid-layout';
const layout = [
{i: 'a', x: 0, y: 0, w: 1, h: 2, isResizable: true},
{i: 'b', x: 1, y: 0, w: 3, h: 2, isResizable: true},
{i: 'c', x: 4, y: 0, w: 1, h: 2, isResizable: true}
];
const MyFirstGrid = () => (
<GridLayout className="layout" layout={layout} cols={12} rowHeight={30} width={1200}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</GridLayout>
);
export default MyFirstGrid;
Responsive Grid Layout
This feature allows you to create grid layouts that adapt to different screen sizes. The layouts object defines the layout for different breakpoints, making the grid responsive.
import React from 'react';
import { Responsive, WidthProvider } from 'react-grid-layout';
const ResponsiveGridLayout = WidthProvider(Responsive);
const layouts = {
lg: [
{i: 'a', x: 0, y: 0, w: 1, h: 2},
{i: 'b', x: 1, y: 0, w: 3, h: 2},
{i: 'c', x: 4, y: 0, w: 1, h: 2}
],
md: [
{i: 'a', x: 0, y: 0, w: 1, h: 2},
{i: 'b', x: 1, y: 0, w: 2, h: 2},
{i: 'c', x: 3, y: 0, w: 1, h: 2}
]
};
const MyResponsiveGrid = () => (
<ResponsiveGridLayout className="layout" layouts={layouts} breakpoints={{lg: 1200, md: 996}} cols={{lg: 12, md: 10}}>
<div key="a">A</div>
<div key="b">B</div>
<div key="c">C</div>
</ResponsiveGridLayout>
);
export default MyResponsiveGrid;
react-grid-system provides a responsive grid layout system for React applications. It is simpler and more lightweight compared to react-grid-layout, focusing primarily on responsive design without built-in drag-and-drop or resizable functionalities.
react-masonry-component is a React wrapper for the Masonry layout library. It allows for creating dynamic grid layouts with a masonry-style arrangement. Unlike react-grid-layout, it does not support drag-and-drop or resizing but excels in creating Pinterest-like layouts.
react-flexbox-grid is a set of React components implementing flexboxgrid.css. It provides a grid system based on flexbox, which is highly responsive and easy to use. However, it lacks the drag-and-drop and resizable features found in react-grid-layout.
React-Grid-Layout is a grid layout system much like Packery or Gridster, for React.
RGL is not as full-featured as the above projects. However, it solves a major pain point I've found with those projects - it is responsive! Try resizing the window in the demo and see how the grid resizes without any fuss.
RGL works just fine with server-side rendering.
RGL is React-only and does not require jQuery.
More demos are coming soon. RGL supports adding and removing components without fuss.
If you have a feature request, please add it as an issue or make a pull request.
Use ReactGridLayout like any other component.
render: function() {
// layout is an array of objects, see the demo
var initialLayout = getOrGenerateLayout();
return (
<ReactGridLayout className="layout" initialLayout={initialLayout}
cols={12} rowHeight={30}>
<div key={1}>1</div>
<div key={2}>2</div>
<div key={3}>3</div>
</ReactGridLayout>
)
}
You can also set layout properties directly on the children:
render: function() {
// layout is an array of objects, see the demo
return (
<ReactGridLayout className="layout" cols={12} rowHeight={30}>
<div key={1} _grid={{x: 0, y: 0, w: 1: h: 2}}>1</div>
<div key={2} _grid={{x: 1, y: 0, w: 1: h: 2}}>2</div>
<div key={3} _grid={{x: 2, y: 0, w: 1: h: 2}}>3</div>
</ReactGridLayout>
)
}
RGL supports the following properties (see the source for the final word on this):
// If true, the container height swells and contracts to fit contents
autoSize: React.PropTypes.bool,
// {name: pxVal}, e.g. {lg: 1200, md: 996, sm: 768, xs: 480}
breakpoints: React.PropTypes.object,
// Can be specified as a single number or a {breakpoint: cols} map
cols: React.PropTypes.oneOfType([
React.PropTypes.object,
React.PropTypes.number
]),
// A selector for the draggable handler
handle: React.PropTypes.string,
// Layout is an array of object with the format:
// {x: Number, y: Number, w: Number, h: Number}
initialLayout: React.PropTypes.array,
// This allows setting this on the server side
initialWidth: React.PropTypes.number,
// margin between items [x, y] in px
margin: React.PropTypes.array,
// Rows have a static height, but you can change this based on breakpoints
// if you like
rowHeight: React.PropTypes.number,
// Flags.
isDraggable: React.PropTypes.bool,
isResizable: React.PropTypes.bool,
// Callback so you can save the layout
onLayoutChange: React.PropTypes.func
{
autoSize: true,
breakpoints: {lg: 1200, md: 996, sm: 768, xs: 480, xxs: 0},
cols: 10,
rowHeight: 150,
initialWidth: 1280,
margin: [10, 10],
isDraggable: true,
isResizable: true,
onLayoutChange: function(){}
}
_grid
key)FAQs
A draggable and resizable grid layout with responsive breakpoints, for React.
The npm package react-grid-layout receives a total of 714,432 weekly downloads. As such, react-grid-layout popularity was classified as popular.
We found that react-grid-layout demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.