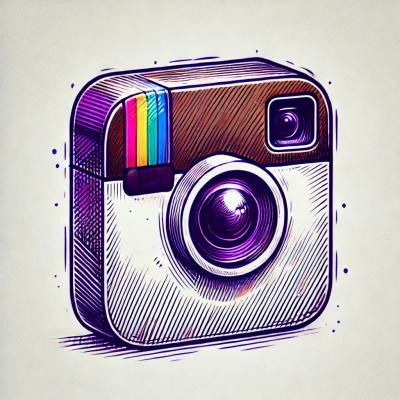
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
react-hook-form
Advanced tools
Performant, flexible and extensible forms library for React Hooks
The react-hook-form npm package is a flexible and performant library that simplifies the process of working with forms in React applications. It provides hooks and components to manage form state and validation with minimal re-renders.
Easy Form State Management
This feature allows you to easily manage form state, handle form submissions, and integrate form validation.
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
const { register, handleSubmit, watch, errors } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input name='example' defaultValue='test' ref={register} />
<input name='exampleRequired' ref={register({ required: true })} />
{errors.exampleRequired && <span>This field is required</span>}
<input type='submit' />
</form>
);
}
Form Validation
This feature provides built-in validation methods and allows you to create custom validation rules for your form inputs.
import React from 'react';
import { useForm } from 'react-hook-form';
function MyForm() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input name='example' ref={register({ required: 'This is required' })} />
{errors.example && <p>{errors.example.message}</p>}
<input type='submit' />
</form>
);
}
Integrating with UI Libraries
This feature allows you to easily integrate react-hook-form with other UI libraries like react-select, material-ui, and antd.
import React from 'react';
import { useForm, Controller } from 'react-hook-form';
import Select from 'react-select';
function MyForm() {
const { control, handleSubmit } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<Controller
as={Select}
options={[{ value: 'chocolate', label: 'Chocolate' }]}
name='ReactSelect'
isClearable
control={control}
rules={{ required: true }}
/>
<input type='submit' />
</form>
);
}
Formik is another popular form library for React. It is known for its simplicity and support for complex form logic. Compared to react-hook-form, Formik may have a slightly larger bundle size and potentially more re-renders, but it offers a more declarative API and built-in helpers for managing form state.
Redux-form integrates form state into the Redux store, providing deep integration with Redux. It is suitable for complex form handling in large-scale applications. However, it can be overkill for simple forms and may lead to more boilerplate compared to react-hook-form, which is more lightweight and requires less setup.
Final Form is a subscription-based form state management library that separates form state from the UI layer. It is framework-agnostic and can be used with React via react-final-form. It offers fine-grained control over form rendering, but react-hook-form tends to have better performance due to its use of uncontrolled components.
Get started | API | Form Builder | FAQs | Examples
npm install react-hook-form
import { useForm } from 'react-hook-form';
function App() {
const {
register,
handleSubmit,
formState: { errors },
} = useForm();
return (
<form onSubmit={handleSubmit((data) => console.log(data))}>
<input {...register('firstName')} />
<input {...register('lastName', { required: true })} />
{errors.lastName && <p>Last name is required.</p>}
<input {...register('age', { pattern: /\d+/ })} />
{errors.age && <p>Please enter number for age.</p>}
<input type="submit" />
</form>
);
}
We’re incredibly grateful to these kind and generous sponsors for their support!
Thank you to our previous sponsors for your generous support!
Thanks go to all our backers! [Become a backer].
Thanks go to these wonderful people! [Become a contributor].
Documentation website supported and backed by Vercel
FAQs
Performant, flexible and extensible forms library for React Hooks
The npm package react-hook-form receives a total of 10,953,478 weekly downloads. As such, react-hook-form popularity was classified as popular.
We found that react-hook-form demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.