What is redux-form?
redux-form is a library that allows you to manage form state in Redux. It provides a way to keep form state in a Redux store, making it easier to manage complex forms and their validation, submission, and other behaviors.
What are redux-form's main functionalities?
Form Creation
This code demonstrates how to create a simple form using redux-form. The `Field` component is used to define form fields, and the `reduxForm` higher-order component is used to connect the form to Redux.
import React from 'react';
import { Field, reduxForm } from 'redux-form';
let SimpleForm = props => {
const { handleSubmit } = props;
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" component="input" type="text" />
</div>
<button type="submit">Submit</button>
</form>
);
};
SimpleForm = reduxForm({
form: 'simple'
})(SimpleForm);
export default SimpleForm;
Form Validation
This code demonstrates how to add validation to a form using redux-form. The `validate` function checks for errors and returns an object with error messages, which are then used by redux-form to display validation errors.
import React from 'react';
import { Field, reduxForm } from 'redux-form';
const validate = values => {
const errors = {};
if (!values.firstName) {
errors.firstName = 'Required';
}
return errors;
};
let ValidatedForm = props => {
const { handleSubmit } = props;
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" component="input" type="text" />
</div>
<button type="submit">Submit</button>
</form>
);
};
ValidatedForm = reduxForm({
form: 'validated',
validate
})(ValidatedForm);
export default ValidatedForm;
Form Submission
This code demonstrates how to handle form submission using redux-form. The `handleSubmit` function is passed a `submit` function that processes the form data.
import React from 'react';
import { Field, reduxForm } from 'redux-form';
const submit = values => {
console.log('Form data:', values);
};
let SubmitForm = props => {
const { handleSubmit } = props;
return (
<form onSubmit={handleSubmit(submit)}>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" component="input" type="text" />
</div>
<button type="submit">Submit</button>
</form>
);
};
SubmitForm = reduxForm({
form: 'submit'
})(SubmitForm);
export default SubmitForm;
Other packages similar to redux-form
formik
Formik is a popular form library for React that provides a simpler API compared to redux-form. It focuses on reducing boilerplate and improving performance by not relying on Redux for form state management. Formik is often preferred for its ease of use and flexibility.
react-hook-form
React Hook Form is a library that uses React hooks to manage form state and validation. It is known for its minimal re-renders and high performance. Unlike redux-form, it does not require Redux and is often chosen for its simplicity and performance benefits.
final-form
Final Form is a framework-agnostic form state management library that can be used with React through the react-final-form package. It offers similar functionality to redux-form but is designed to be more lightweight and flexible, without the need for Redux.
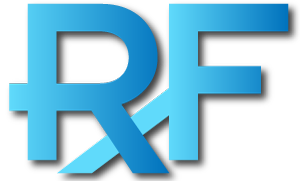
redux-form
You build great forms, but do you know HOW users use your forms? Find out with Form Nerd! Professional analytics from the creator of Redux Form.

redux-form
works with React Redux to
enable an html form in React to use
Redux to store all of its state.
💰Psst!! Do you know React and Redux? Sign up with Triplebyte to get offers from top tech companies! 💰
⚠️ ATTENTION ⚠️
If you're just getting started with your application and are looking for a form solution, the general consensus of the community is that you should not put your form state in Redux. The author of Redux Form took all of the lessons he learned about form use cases from maintaining Redux Form and built 🏁 React Final Form, which he recommends you use if you are just starting your project. It's also pretty easy to migrate to from Redux Form, because the <Field>
component APIs are so similar. Here is a blog post where he explains his reasoning, or there are two talks if you prefer video. Formik is also a nice solution.
The only good reason, in the author's view, to use Redux Form in your application is if you need really tight coupling of your form data with Redux, specifically if you need to subscribe to it and modify it from parts of your application far from your form component, e.g. on another route. If you don't have that requirement, use 🏁 React Final Form.
Installation
npm install --save redux-form
Documentation
🏖 Code Sandboxes 🏖
You can play around with redux-form
in these sandbox versions of the Examples.
Videos
 |
---|
A Practical Guide to Redux Form – React Alicante 2017 |
 |
---|
Abstracting Form State with Redux Form – JS Channel 2016 |
Contributors
This project exists thanks to all the people who contribute.

Backers
Thank you to all our backers! 🙏 [Become a backer]

Support this project by becoming a sponsor. Your logo will show up here with a link to your website. [Become a sponsor]

