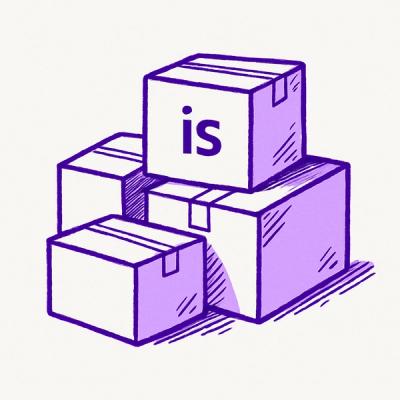
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
react-hot-toast
Advanced tools
Smoking hot React Notifications. Lightweight, customizable and beautiful by default.
react-hot-toast is a lightweight and customizable toast notification library for React applications. It provides a simple API to create and manage toast notifications with various customization options.
Basic Toast Notification
This feature allows you to display a basic toast notification with a simple message. The `Toaster` component is used to render the toast notifications.
import toast, { Toaster } from 'react-hot-toast';
function App() {
return (
<div>
<button onClick={() => toast('Hello, World!')}>Show Toast</button>
<Toaster />
</div>
);
}
Custom Toast Notification
This feature allows you to customize the toast notification with various options such as duration, position, and styles.
import toast, { Toaster } from 'react-hot-toast';
function App() {
return (
<div>
<button onClick={() => toast('Custom Toast', {
duration: 4000,
position: 'top-center',
style: {
background: '#333',
color: '#fff',
},
})}>Show Custom Toast</button>
<Toaster />
</div>
);
}
Promise-based Toast Notification
This feature allows you to display toast notifications based on the state of a promise. It shows different messages for loading, success, and error states.
import toast, { Toaster } from 'react-hot-toast';
function App() {
const handleClick = () => {
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => resolve('Success!'), 2000);
});
toast.promise(myPromise, {
loading: 'Loading...',
success: 'Success!',
error: 'Error!',
});
};
return (
<div>
<button onClick={handleClick}>Show Promise Toast</button>
<Toaster />
</div>
);
}
react-toastify is another popular toast notification library for React. It offers similar functionality to react-hot-toast, including customizable toasts and promise-based notifications. However, react-toastify has a larger bundle size compared to react-hot-toast.
notistack is a highly customizable notification library for React. It allows stacking of notifications and provides a flexible API for managing notifications. Compared to react-hot-toast, notistack offers more advanced features like stacking and dismissing notifications programmatically.
react-notifications-component is a library for creating customizable notifications in React. It provides a variety of notification types and customization options. While it offers similar functionality to react-hot-toast, it has a more complex API and larger bundle size.
useToaster()
pnpm add react-hot-toast
npm install react-hot-toast
Add the Toaster to your app first. It will take care of rendering all notifications emitted. Now you can trigger toast()
from anywhere!
import toast, { Toaster } from 'react-hot-toast';
const notify = () => toast('Here is your toast.');
const App = () => {
return (
<div>
<button onClick={notify}>Make me a toast</button>
<Toaster />
</div>
);
};
Find the full API reference on official documentation.
FAQs
Smoking hot React Notifications. Lightweight, customizable and beautiful by default.
The npm package react-hot-toast receives a total of 1,128,037 weekly downloads. As such, react-hot-toast popularity was classified as popular.
We found that react-hot-toast demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.