react-infinite-observer

Check live StoryBook demo
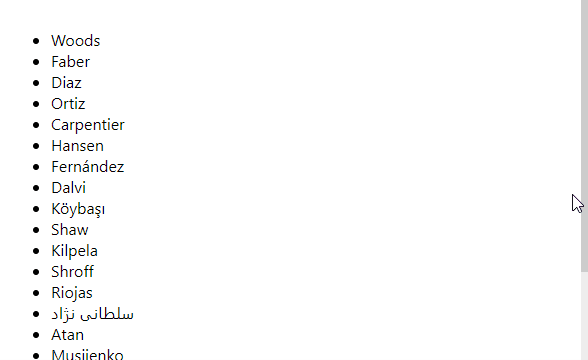
Overview
React Infinite Observer is a lightweight React hook for handling infinite scrolling in your web applications. It enables you to efficiently load and display large sets of data by triggering requests as the user scrolls down/up the page.
Features
- Infinite Scrolling: Load more data automatically as the user scrolls down/up the page.
- Easy Integration: Simple integration into your existing React components.
- Flexibility: Customize the trigger point for fetching more data and loading indicator appearance.
- Full Manual Control: The hook provides option to autofetch data without scolling until height of list > screen height
Getting Started
Installation
npm install react-infinite-observer
or
yarn add react-infinite-observer
Usage
Static Ref
import { FC, useState, useCallback, useEffect } from 'react';
import { useInfiniteObserver } from 'react-infinite-observer';
export const App: FC = () => {
const [items, setItems] = useState([]);
const [isLoading, setIsLoading] = useState(false);
const [page, setPage] = useState(1);
const onIntersection = useCallback(() => {
setPage((pageNo) => pageNo + 1);
}, []);
const [setRefElement] = useInfiniteObserver(onIntersection);
const fetchData = async () => {
setIsLoading(true);
setIsLoading(false);
};
useEffect(() => {
fetchData();
}, [page]);
return (
<div>
<ul>
{items.map((item) => (
<li key={`${item.id.name}-${item.id.value}-${item.login.uuid}`}>
{item.name.last}
</li>
))}
<div ref={setRefElement}></div>
</ul>
{isLoading && <p>Loading...</p>}
</div>
);
};
Dynamic Ref (keep fetching data until screen is filled)
import { FC, useState, useCallback, useEffect } from 'react';
import { useInfiniteObserver } from 'react-infinite-observer';
export const App: FC = () => {
const [items, setItems] = useState([]);
const [isLoading, setIsLoading] = useState(false);
const [page, setPage] = useState(1);
const onIntersection = useCallback(() => {
setPage((pageNo) => pageNo + 1);
}, []);
const [setRefElement] = useInfiniteObserver(onIntersection);
const fetchData = async () => {
setIsLoading(true);
setIsLoading(false);
};
useEffect(() => {
fetchData();
}, [page]);
return (
<div>
<ul>
{items.map((item, index) => (
<li
key={`${item.id.name}-${item.id.value}-${item.login.uuid}`}
ref={index === items.length - 1 ? setRefElement : undefined}
>
{item.name.last}
</li>
))}
</ul>
{isLoading && <p>Loading...</p>}
</div>
);
};
API
infiniteObserverOptions
Provide these as the options argument in the useInfiniteObserver
hook.
Name | Type | Default | Description |
---|
threshold | number | 1 | Number between 0 and 1 indicating the percentage that should be visible before triggering. |
onIntersection | () => void | undefined | Call this function whenever the Ref Element is in view for the first time. |
Example
Check out the stackblitz snippet for a simple implementation.
License
This project is licensed under the MIT License - see the LICENSE file for details.