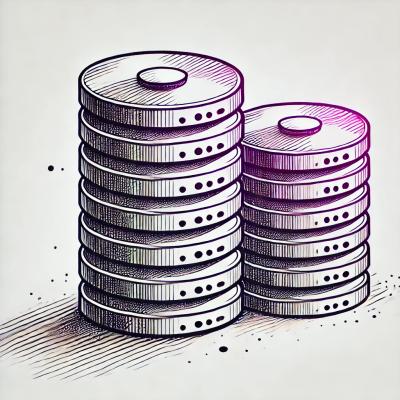
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
react-lineto
Advanced tools
Draw a line between two elements in React.
yarn install
yarn run demo
Browse to localhost:4567.
Draw line between two DOM elements.
import LineTo from 'react-lineto';
function render() {
return (
<div>
<div className="A">Element A</div>
<div className="B">Element B</div>
<LineTo from="A" to="B" />
</div>
);
}
If using multiple instances of <LineTo />
inside separate components, you must provide a unique key for each of the container divs.
Name | Type | Description | Example Values |
---|---|---|---|
borderColor | string | Border color | #f00 , red , etc. |
borderStyle | string | Border style | solid , dashed , etc. |
borderWidth | number | Border width (px) | |
className | string | Desired CSS className for the rendered element | |
delay | number or bool | Force render after delay (ms) | 0 , 1 , 100 , true |
fromAnchor | string | Anchor for starting point (Format: "x y") | top right , bottom center , left , 100% 0 |
from* | string | CSS class name of the first element | |
toAnchor | string | Anchor for ending point (Format: "x y") | top right , bottom center , left , 100% 0 |
to* | string | CSS class name of the second element | |
within | string | CSS class name of the desired container | |
zIndex | number | Z-index offset |
* Required
Draw stepped line between two DOM elements.
import { SteppedLineTo } from 'react-lineto';
function render() {
return (
<div>
<div className="A">Element A</div>
<div className="B">Element B</div>
<SteppedLineTo from="A" to="B" orientation="v" />
</div>
);
}
Name | Type | Description | Example Values |
---|---|---|---|
borderColor | string | Border color | #f00 , red , etc. |
borderStyle | string | Border style | solid , dashed , etc. |
borderWidth | number | Border width (px) | |
className | string | Desired CSS className for the rendered element | |
delay | number or bool | Force render after delay (ms) | 0 , 1 , 100 , true |
fromAnchor | string | Anchor for starting point (Format: "x y") | top right , bottom center , left , 100% 0 |
from* | string | CSS class name of the first element | |
orientation | enum | "h" for horizonal, "v" for vertical | h or v |
toAnchor | string | Anchor for ending point (Format: "x y") | top right , bottom center , left , 100% 0 |
to* | string | CSS class name of the second element | |
within | string | CSS class name of the desired container | |
zIndex | number | Z-index offset |
* Required
Draw line using pixel coordinates (relative to viewport).
import { Line } from 'react-lineto';
function render() {
return (
<Line x0={0} y0={0} x1={10} y1={10} />
);
}
Name | Type | Description | Example Values |
---|---|---|---|
borderColor | string | Border color | #f00 , red , etc. |
borderStyle | string | Border style | solid , dashed , etc. |
borderWidth | number | Border width (px) | |
className | string | Desired CSS className for the rendered element | |
within | string | CSS class name of the desired container | |
x0* | number | First X coordinate | |
x1* | number | Second X coordinate | |
y0* | number | First Y coordinate | |
y1* | number | Second Y coordinate | |
zIndex | number | Z-index offset |
* Required
package.json
CHANGELOG.md
yarn build
or ./scripts/update
master
branch and tags to origin3.3.0
FAQs
Draw a line between two elements in React.
The npm package react-lineto receives a total of 6,773 weekly downloads. As such, react-lineto popularity was classified as popular.
We found that react-lineto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.