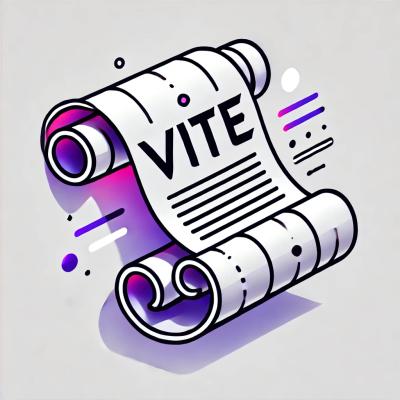
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
react-marksome
Advanced tools
Lightweight, flexible and readable labels in React using a subset of markdown
Parses some markdown, builds a tree of segments and renders them in React.
It was designed for adding basic support for styling and links to singleline strings.
See Rationale for more info.
Install:
npm i react-marksome
Import and render the Marksome component:
import { Marksome } from 'react-marksome';
function Demo() {
const text =
'The *quick* *brown* **fox** jumps over the *lazy* **dog**. [Wikipedia][1]';
const references: References = {
'1':
'https://en.wikipedia.org/wiki/The_quick_brown_fox_jumps_over_the_lazy_dog',
};
return <Marksome text={text} references={references} />;
}
renders the following line:
The quick brown fox jumps over the lazy dog. Wikipedia
For more examples, see the stories together with related fixtures.
React component that parses and renders a subset of markdown.
It expects the markdown text to be provided via a text
prop, which then is combined with reference links ([label][reference]
) defined under the references
prop.
type MarksomeProps = HTMLAttributes<HTMLSpanElement> & {
text: string;
references?: References;
};
type References = Record<string, string | ReferenceRenderFunction>;
type ReferenceRenderFunction = (children: ReactNode) => ReactElement;
See Quick start.
One can actually render custom components in the place of reference links by providing a render function instead of link url:
function CustomComponentsDemo() {
const text = 'The following is an actual button: [*Howdie*][greeting-button]';
const references: References = {
'greeting-button': (children) => (
<button
onClick={() => {
alert('Hello!');
}}
>
{children}
</button>
),
};
return <Marksome text={text} references={references} />;
}
The current subset of markdown that is supported is:
Emphasis (*Emphasis*) and strong emphasis (**strong emphasis**) parcing respects the related commonmark spec section.
Influenced by the related commonmark spec section, link references can be defined in a couple of ways:
There are certain quirks in marksome that are non-spec:
references
prop or notreferences
prop (ex: case-sensitive, no space-trimming, etc)If reference links are not being matched as you desire, disable unintended matches by escaping the related opening (\[) or closing (\]) brackets.
By restricting ourselves to support only some markdown we're able to:
Additionally, we build out a tree of segments instead of simply using string replacement mostly for extensibility and configuratility, like being able to render segments with custom React components.
All of the above means that users don't need to worry about escaping the text since:
The following browserslist is supported without the need of any polyfills:
caniuse-lite db date: 2nd Jan 2022
This project was bootstrapped with TSDX. Check its docs for more info on the commands.
npm run storybook
This loads the stories from ./stories
.
Jest tests are set up to run with npm test
.
Calculates the real cost of your library using size-limit with npm run size
and visualize it with npm run analyze
.
FAQs
Lightweight, flexible and readable labels in React using a subset of markdown
The npm package react-marksome receives a total of 2,677 weekly downloads. As such, react-marksome popularity was classified as popular.
We found that react-marksome demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.