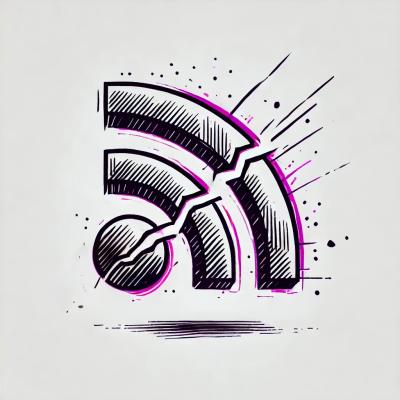
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
react-native-auto-size-text
Advanced tools
React Native component for Android and iOS that provides several ways to resize text within a certain dimension/parent.
React Native component for Android and iOS that provides several ways to resize text within a certain dimension/parent.
Port of auto_size_text
yarn react-native-auto-size-text
npm i react-native-auto-size-text
Import react-native-auto-size-text
and ResizeTextMode
import { AutoSizeText, ResizeTextMode } from 'react-native-auto-size-text';
Choose one of the modes below:
Required props: fontSize
, numberOfLines
and mode
.
<AutoSizeText
fontSize={32}
numberOfLines={2}
mode={ResizeTextMode.max_lines}>
This string will be automatically resized to fit on two lines.
</AutoSizeText>
Required props: minFontSize
, numberOfLines
and mode
.
<AutoSizeText
numberOfLines={3}
minFontSize={21}
mode={ResizeTextMode.min_font_size}>
This string's size will not be smaller than 21. It will be automatically
resized to fit on 3 lines.
</AutoSizeText>
Required props: mode
.
<AutoSizeText
mode={ResizeTextMode.group}>
This mode will fit the available space and sync their text size
</AutoSizeText>
Required props: fontSize
, numberOfLines
, granularity
and mode
.
<AutoSizeText
fontSize={48}
numberOfLines={4}
granularity={10}
mode={ResizeTextMode.step_granularity}>
This String changes its size with a stepGranularity of 10. It will be automatically
resized to fit on 4 lines.
</AutoSizeText>
Required props: fontSizePresets
, numberOfLines
and mode
.
<AutoSizeText
fontSizePresets={[64, 42, 24]}
numberOfLines={4}
mode={ResizeTextMode.preset_font_sizes}>
This String has only three allowed sizes: 64, 42 and 24.
It will be automatically resized to fit on 4 lines.
With this setting, you have most control
</AutoSizeText>
Required props: fontSize
, numberOfLines
, overFlowReplacement
and mode
.
<AutoSizeText
fontSize={32}
numberOfLines={3}
overFlowReplacement={'Text overflowing'}
mode={ResizeTextMode.overflow_replacement}>
This String's size will not be smaller than 32.
It will be automatically resized to fit on 3 lines.
Otherwise it will be replaced by a replacement string. Here's an example.
</AutoSizeText>
name | description | type | default |
---|---|---|---|
fontSize | Font size | number | 14 |
numberOfLines | Number of lines before rescaling | number | none |
mode | Resize text mode | ResizeTextMode | ResizeTextMode.max_lines |
minFontSize | Minimum font size | number | none |
granularity | Text resize granularity | number | none |
fontSizePresets | Font size presets | number[] | none |
Overflowreplacement | Replacement if the text overflows parent | string | '' |
style | Text style | function: () => {} | |
TextProps | All other <Text/> props | function: () => {} |
import React, {useState} from 'react';
import {ScrollView, StyleSheet, Text, TextInput, View} from 'react-native';
import {AutoSizeText, ResizeTextMode} from 'react-native-auto-size-text';
const App = () => {
const [text, setText] = useState<string>('');
return (
<ScrollView
style={styles.scrollViewContainer}
contentContainerStyle={styles.container}>
<TextInput
style={styles.input}
onChangeText={e => setText(e)}
value={text}
/>
<Text>MaxLines</Text>
<View style={styles.textWrapper}>
<AutoSizeText
fontSize={64}
numberOfLines={2}
mode={ResizeTextMode.max_lines}>
{text}
</AutoSizeText>
</View>
<Text>MinFontSize</Text>
<View style={styles.textWrapper}>
<AutoSizeText
numberOfLines={3}
minFontSize={18}
mode={ResizeTextMode.min_font_size}>
{text}
</AutoSizeText>
</View>
<Text>PresetFontSizes</Text>
<View style={styles.textWrapper}>
<AutoSizeText
fontSizePresets={[50, 30, 10]}
numberOfLines={3}
mode={ResizeTextMode.preset_font_sizes}>
{text}
</AutoSizeText>
</View>
<Text>OverflowReplacement</Text>
<View style={styles.textWrapper}>
<AutoSizeText
fontSize={12}
numberOfLines={1}
mode={ResizeTextMode.overflow_replacement}
overFlowReplacement={'Text overflowing'}>
{text}
</AutoSizeText>
</View>
<Text>Group</Text>
<View style={styles.textWrapper}>
<AutoSizeText mode={ResizeTextMode.group} fontSize={2048}>
{text}
</AutoSizeText>
</View>
<Text>StepGranularity</Text>
<View style={styles.textWrapper}>
<AutoSizeText
mode={ResizeTextMode.step_granularity}
fontSize={64}
numberOfLines={2}
granularity={10}>
{text}
</AutoSizeText>
</View>
</ScrollView>
);
};
const styles = StyleSheet.create({
container: {
justifyContent: 'center',
alignItems: 'center',
},
textWrapper: {
borderColor: '#bcbcbc',
borderRadius: 10,
width: '80%',
margin: 16,
height: 200,
borderWidth: 2,
},
scrollViewContainer: {
flex: 1,
},
input: {
height: 80,
width: '100%',
margin: 12,
borderWidth: 1,
},
});
export default App;
Contributions are what make the open source community such an amazing place to be learn, inspire, and create. Any contributions you make are greatly appreciated.
git checkout -b feature/AmazingFeature
)git commit -m 'Add some AmazingFeature'
)git push origin feature/AmazingFeature
)FAQs
React Native component for Android and iOS that provides several ways to resize text within a certain dimension/parent.
The npm package react-native-auto-size-text receives a total of 771 weekly downloads. As such, react-native-auto-size-text popularity was classified as not popular.
We found that react-native-auto-size-text demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.