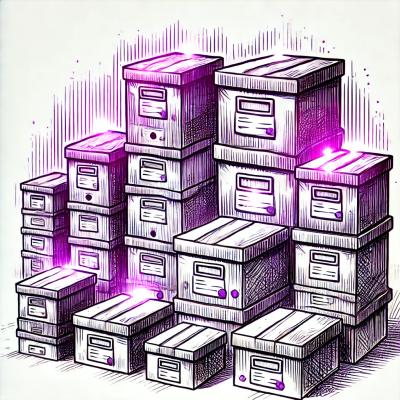
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
react-native-code-push
Advanced tools
This plugin provides client-side integration for the CodePush service, allowing you to easily add a dynamic code update experience to your React Native apps.
The CodePush React Native API provides two primary mechanisms for discovering updates and dynamically applying them within your apps:
When getting started using CodePush, we would recommended using the sync mode until you discover that it doesn't suit your needs. That said, if you have a user scenario that isn't currently covered well by sync, please let us know since that would be valuable feedback.
NOTE: We don't currently have support for an "automatic mode" which provides a "code-free" experience to adding in dynamic update discovery and acquisition. If that would be valuable to you, please let us know.
A React Native application's assets (JavaScript code and other resources) are traditionally bundled up as a .jsbundle
file which is loaded from the application installation location on the target device during runtime. After you submit an update to the store, the user downloads the update, and those assets will be replaced with the new assets.
CodePush is here to simplify this process by allowing you to instantly update your application's assets without having to submit a new update to the store. We do this by allowing you to upload and manage your React Native app bundles on our CodePush server. In the application, we check for the presence of updated bundles on the server. If they are available, we will install and persist them to the internal storage of the device. If a new bundle is installed, the application will reload from the updated package location.
Acquire the React Native CodePush plugin by running the following command within your app's root directory:
npm install --save react-native-code-push
Once you've acquired the CodePush plugin, you need to integrate it into the Xcode project of your React Native app. To do this, take the following steps:
Open your app's Xcode project
Find the CodePush.xcodeproj
file witin the node_modules/react-native-code-push
directory, and drag it into the Libraries
node in Xcode
Select the project node in Xcode and select the "Build Phases" tab of your project configuration.
Drag libCodePush.a
from Libraries/CodePush.xcodeproj/Products
into the "Link Binary With Libraries" secton of your project's "Build Phases" configuration.
Under the "Build Settings" tab of your project configuration, find the "Header Search Paths" section and edit the value.
Add a new value, $(SRCROOT)/../node_modules/react-native-code-push
and select "recursive" in the dropdown.
Once your Xcode project has been setup to build/link the CodePush plugin, you need to configure your app to consult CodePush for the location of your JS bundle, since it will "take control" of managing the current and all future versions. To do this, perform the following steps:
Open up the AppDelegate.m
file, and add an import statement for the CodePush headers:
#import "CodePush.h"
Find the following line of code, which loads your JS Bundle from the packager's dev server:
jsCodeLocation = [NSURL URLWithString:@"http://localhost:8081/index.ios.bundle?platform=ios&dev=true"];
Replace it with this line:
jsCodeLocation = [CodePush getBundleUrl];
This change configures your app to always load the most recent version of your app's JS bundle. On the initial launch, this will correspond to the file that was compiled with the app. However, after an update has been pushed via CodePush, this will return the location of the most recently applied update.
To let the CodePush runtime know which deployment it should query for updates against, perform the following steps:
Info.plist
and add a new CodePushDeploymentKey
entry, whose value is the key of the deployment you want to configure this app against (e.g. the Staging deployment for FooBar app)Info.plist
make sure your CFBundleShortVersionString
value is a valid semver version (e.g. 1.0.0 not 1.0)With the CodePush plugin downloaded and linked, and your app asking CodePush where to get the right JS bundle from, the only thing left is to add the neccessary code to your app to control the following:
The simplest way to do this is to perform the following in your app's root component:
Import the JavaScript module for CodePush:
var CodePush = require("react-native-code-push")
Call the sync
method from within the componentDidMount
lifecycle event, to initiate a background update on each app start:
CodePush.sync();
If an update is available, a dialog will be displayed to the user asking them if they would like to install it. If the update was marked as mandatory, then the dialog will
omit the option to decline installation. The sync
method takes a handful of options to customize this experience, so refer to its API reference if you'd like to tweak its default behavior.
Once your app has been configured and distributed to your users, and you've made some JS changes, it's time to release it to them instantly! To do this, run the following steps:
react-native bundle
in order to generate the JS bundle for your app.code-push release <appName> <deploymentName> ./ios/main.jsbundle <appVersion>
in order to publish the generated JS bundle to the server (assuming your CWD is the root directory of your React Native app).And that's it! For more information regarding the CodePush API, including the various options you can pass to the sync
method, refer to the reference section below.
When you require the react-native-code-push
module, that object provides the following methods directly on it:
RemotePackage
that can be subsequently downloaded.codePush.checkForUpdate(): Promise<RemotePackage>;
Queries the CodePush service for an update against the configured deployment. This method returns a promise which resolves to a RemotePackage
that can be subsequently downloaded.
checkForUpdate
returns a Promise that resolves to one of two values:
null
if there is no update availableRemotePackage
instance that represents an available update that can be downloadedExample Usage:
codePush.checkForUpdate().then((update) => {
if (!update) {
console.log("The app is up to date!");
} else {
console.log("An update is available! Should we download it?");
}
});
codePush.getCurrentPackage(): Promise<LocalPackage>;
Gets information about the currently applied package (e.g. description, installation time).
This method returns a Promise that resolves with the LocalPackage
instance that represents the running update. This API is only useful for advanced scenarios, and so many devs won't need to concern themselves with it.
codePush.notifyApplicationReady(): Promise<void>;
Notifies the CodePush runtime that an update is considered successful, and therefore, a rollback isn't neccessary. Calling this function is required whenever the rollbackTimeout
parameter is specified when calling either LocalPackage.apply
or sync
. If you specify a rollbackTimeout
, and don't call notifyApplicationReady
, the CodePush runtime will assume that the applied update has failed and roll back to the previous version.
If the rollbackTimeout
parameter was not specified, the CodePush runtime will not enforce any automatic rollback behavior, and therefore, calling this function is not required and will result in a no-op.
codePush.sync(options: Object): Promise<Number>;
Provides a simple option for checking for an update, displaying a notification to the user, downloading it and then applying it, all while also respecting the policy that your release was published with. This method effectively composes together the "advanced mode" APIs for you, so that you don't need to handle any of the following scenarios yourself:
If you want to pivot whether you check and/or download an available update based on the end-user's device battery level, network conditions, etc. then simply wrap the call to sync
in a condition that ensures you only call it when desired.
The method accepts an options object that allows you to customize numerous aspects of the default behavior, all of which provide sensible values by default:
false
." Description: "
true
."Continue"
."An update is available that must be installed."
."Ignore"
."Install"
."An update is available. Would you like to install it?"
.0
, which disabled rollback protection."Update available"
.The method returns a Promise
that is resolved to a SyncStatus
integer code, which indicates why the sync
call succeeded. This code can be one of the following values:
If the update check and/or the subseqeuent download fails for any reason, the Promise
object returned by sync
will be rejected with the reason.
Example Usage:
codePush.sync()
.then((status) => {
if (status == codePush.SyncStatus.UPDATE_APPLIED) {
// Do any neccessary work here before the app
// is restarted in order to apply the update
}
})
.catch((reason) => {
// Do something with the failure
});
The sync
method can be called anywhere you'd like to check for an update. That could be in the componentWillMount
lifecycle event of your root component, the onPress handler of a <TouchableHighlight>
component, in the callback of a periodic timer, or whatever else makes sense for your needs. Just like the checkForUpdate
method, it will perform the network request to check for an update in the background, so it won't impact your UI thread and/or JavaScript thread's responsiveness.
The checkForUpdate
and getCurrentPackage
methods return promises, that when resolved, provide acces to "package" objects. The package represents your code update as well as any extra metadata (e.g. description, mandatory). The CodePush API has the distinction between the following types of packages:
Contains details about an update package that has been downloaded locally or already applied (currently installed package). You can get a reference to an instance of this object either by calling the module-level getCurrentPackage
method, or as the value of the promise returned by the download
method of a RemotePackage.
Info.plst
file.sync
method will automatically ignore updates which have previously failed, so you only need to worry about this property if using checkForUpdate
.notifyApplicationReady
for the given number of milliseconds.
If notifyApplicationReady
is called before the time period specified by rollbackTimeout, the apply operation is considered a success.
Otherwise, the apply operation will be marked as failed, and the application is reverted to its previous version.Contains details about an update package that is available for download. You get a reference to an instance this object by calling the checkForUpdate
method when an update is available. If you are using the sync
API, you don't need to worry about the RemotePackage
, since it will handle the download and application process automatically for you.
The RemotePackage
inherits all of the same properties as the LocalPackage
, but includes one additional one:
download
method will automatically handle the acquisition of updates for you.LocalPackage
.npm install
cd
into Examples/CodePushDemoApp
npm install
Info.plist
and fill in the value for CodePushDeploymentKeynpm start
to launch the packagerCodePushDemoApp.xcodeproj
in XcodeCodePushDemoApp.xcodeproj
in XcodeFAQs
React Native plugin for the CodePush service
The npm package react-native-code-push receives a total of 77,626 weekly downloads. As such, react-native-code-push popularity was classified as popular.
We found that react-native-code-push demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.