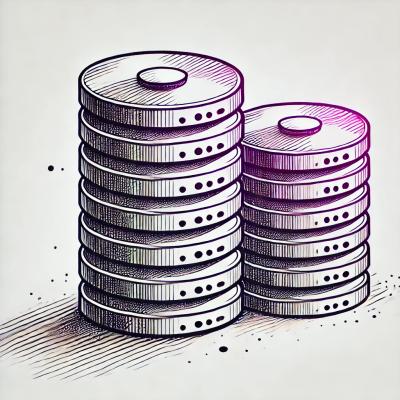
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
react-native-ffmpeg
Advanced tools
FFmpeg for React Native
Based on MobileFFmpeg
Supports
Both Android and IOS
FFmpeg v4.1
and v4.2-dev-x
(master) releases
arm-v7a
, arm-v7a-neon
, arm64-v8a
, x86
and x86_64
architectures on Android
armv7
, armv7s
, arm64
, arm64e
, i386
and x86_64
architectures on IOS
24 external libraries
fontconfig
, freetype
, fribidi
, gmp
, gnutls
, kvazaar
, lame
, libaom
, libass
, libiconv
, libilbc
, libtheora
, libvorbis
, libvpx
, libwebp
, libxml2
, opencore-amr
, opus
, shine
, snappy
, soxr
, speex
, twolame
, wavpack
4 external libraries with GPL license
vid.stab
, x264
, x265
, xvidcore
zlib
and MediaCodec
Android system libraries
bzip2
, zlib
IOS system libraries and AudioToolbox
, CoreImage
, VideoToolbox
, AVFoundation
IOS system frameworks
Licensed under LGPL 3.0, can be customized to support GPL v3.0
Includes eight different packages with different external libraries enabled in FFmpeg
min | min-gpl | https | https-gpl | audio | video | full | full-gpl | |
---|---|---|---|---|---|---|---|---|
external libraries | - | vid.stab x264 x265 xvidcore | gmp gnutls | gmp gnutls vid.stab x264 x265 xvidcore | lame libilbc libvorbis opencore-amr opus shine soxr speex twolame wavpack | fontconfig freetype fribidi kvazaar libaom libass libiconv libtheora libvpx libwebp snappy | fontconfig freetype fribidi gmp gnutls kvazaar lame libaom libass libiconv libilbc libtheora libvorbis libvpx libwebp libxml2 opencore-amr opus shine snappy soxr speex twolame wavpack | fontconfig freetype fribidi gmp gnutls kvazaar lame libaom libass libiconv libilbc libtheora libvorbis libvpx libwebp libxml2 opencore-amr opus shine snappy soxr speex twolame vid.stab wavpack x264 x265 xvidcore |
android system libraries | zlib MediaCodec | |||||||
ios system libraries | zlib AudioToolbox AVFoundation CoreImage VideoToolbox bzip2 |
$ yarn add react-native-ffmpeg
$ react-native link react-native-ffmpeg
Add react-native-ffmpeg
pod to your Podfile
and run pod install
pod 'react-native-ffmpeg', :podspec => '../node_modules/react-native-ffmpeg/ios/react-native-ffmpeg.podspec'
DO NOT USE react-native link
on IOS. react-native link
breaks Cocoapods dependencies.
Installation of react-native-ffmpeg
using instructions in 2.1
and 2.2
enables the default package, which is based
on https
package. It is possible to enable other installed packages using the following steps.
android/settings.gradle
file and modify projectDir
for react-native-ffmpeg
by appending package name at
the end of the path.
project(':react-native-ffmpeg').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-ffmpeg/android/<package name>')
ios/Podfile
file and modify podspec
path for react-native-ffmpeg
by appending package name at the end of
the name. After that run pod install
again.
pod 'react-native-ffmpeg-<package name>', :podspec => '../node_modules/react-native-ffmpeg/ios/react-native-ffmpeg-<package name>.podspec'
Execute commands.
import { LogLevel, RNFFmpeg } from 'react-native-ffmpeg';
RNFFmpeg.execute('-i file1.mp4 -c:v mpeg4 file2.mp4', ' ').then(result => console.log("FFmpeg process exited with rc " + result.rc));
import { LogLevel, RNFFmpeg } from 'react-native-ffmpeg';
RNFFmpeg.executeWithArguments(["-i", "file1.mp4", "-c:v", "mpeg4", "file2.mp4"]).then(result => console.log("FFmpeg process exited with rc " + result.rc));
Check execution output. Zero represents successful execution, non-zero values represent failure.
RNFFmpeg.getLastReturnCode().then(result => {
console.log("Last return code: " + result.lastRc);
});
RNFFmpeg.getLastCommandOutput().then(result => {
console.log("Last command output: " + result.lastCommandOutput);
});
Stop an ongoing operation. Note that this function does not wait for termination to complete and returns immediately.
RNFFmpeg.cancel();
Get media information for a file.
RNFFmpeg.getMediaInformation('<file path or uri>').then(info => {
console.log('Result: ' + JSON.stringify(info));
});
RNFFmpeg.getMediaInformation('<file path or uri>').then(info => {
console.log('Result: ' + JSON.stringify(info));
console.log('Media Information');
console.log('Path: ' + info.path);
console.log('Format: ' + info.format);
console.log('Duration: ' + info.duration);
console.log('Start time: ' + info.startTime);
console.log('Bitrate: ' + info.bitrate);
if (info.streams) {
for (var i = 0; i < info.streams.length; i++) {
console.log('Stream id: ' + info.streams[i].index);
console.log('Stream type: ' + info.streams[i].type);
console.log('Stream codec: ' + info.streams[i].codec);
console.log('Stream full codec: ' + info.streams[i].fullCodec);
console.log('Stream format: ' + info.streams[i].format);
console.log('Stream full format: ' + info.streams[i].fullFormat);
console.log('Stream width: ' + info.streams[i].width);
console.log('Stream height: ' + info.streams[i].height);
console.log('Stream bitrate: ' + info.streams[i].bitrate);
console.log('Stream sample rate: ' + info.streams[i].sampleRate);
console.log('Stream sample format: ' + info.streams[i].sampleFormat);
console.log('Stream channel layout: ' + info.streams[i].channelLayout);
console.log('Stream sar: ' + info.streams[i].sampleAspectRatio);
console.log('Stream dar: ' + info.streams[i].displayAspectRatio);
console.log('Stream average frame rate: ' + info.streams[i].averageFrameRate);
console.log('Stream real frame rate: ' + info.streams[i].realFrameRate);
console.log('Stream time base: ' + info.streams[i].timeBase);
console.log('Stream codec time base: ' + info.streams[i].codecTimeBase);
}
}
});
Enable log callback and redirect all FFmpeg
logs to a console/file/widget.
logCallback = (logData) => {
console.log(logData.log);
};
...
RNFFmpeg.enableLogCallback(this.logCallback);
Enable statistics callback and follow the progress of an ongoing operation.
statisticsCallback = (statisticsData) => {
console.log('Statistics; frame: ' + statisticsData.videoFrameNumber.toFixed(1) + ', fps: ' + statisticsData.videoFps.toFixed(1) + ', quality: ' + statisticsData.videoQuality.toFixed(1) +
', size: ' + statisticsData.size + ', time: ' + statisticsData.time);
};
...
RNFFmpeg.enableStatisticsCallback(this.statisticsCallback);
Poll statistics without implementing statistics callback.
RNFFmpeg.getLastReceivedStatistics().then(stats => console.log('Stats: ' + JSON.stringify(stats)));
Reset statistics before starting a new operation.
RNFFmpeg.resetStatistics();
Set log level.
RNFFmpeg.setLogLevel(LogLevel.AV_LOG_WARNING);
Register your own fonts by specifying a custom fonts directory, so they are available to use in FFmpeg
filters. Please note that this function can not work on relative paths, you need to provide full file system path.
RNFFmpeg.setFontDirectory('<folder with fonts>', null);
RNFFmpeg.setFontDirectory('<folder with fonts>', { my_easy_font_name: "my complex font name" });
Use your own fontconfig
configuration.
RNFFmpeg.setFontconfigConfigurationPath('<fontconfig configuration directory>');
Disable log functionality of the library. Logs will not be printed to console and log callback will be disabled.
RNFFmpeg.disableLogs();
Disable statistics functionality of the library. Statistics callback will be disabled but the last received statistics data will be still available.
RNFFmpeg.disableStatistics();
List enabled external libraries.
RNFFmpeg.getExternalLibraries().then(externalLibraries => {
console.log(externalLibraries);
});
0.1.x
releases are based on FFmpeg v4.0.2
and MobileFFmpeg v2.x
0.2.x
releases are based on FFmpeg v4.1-dev
and MobileFFmpeg v3.x
0.3.0
and 0.3.1
releases are based on FFmpeg v4.2-dev
and MobileFFmpeg v4.2
master
includes the latest released version v0.3.1
development
branch includes new features and unreleased fixesreact-native-ffmpeg
uses MobileFFmpeg in its core.
Starting from v4.2
, MobileFFmpeg
binaries are published in two different variants: Main Release
and LTS Release
.
Main releases include complete functionality of the library and support the latest SDK/API features
LTS releases are customized to support a wide range of devices. They are built using older API/SDK versions, so some features are not available for them
By default, react-native-ffmpeg
releases depend on LTS
releases, to be backward compatible with its earlier versions. But you can change MobileFFmpeg
variant and/or version used to support a specific feature/architecture.
To do that on Android, set a different value to mobileFFmpegVersion
variable inside build.gradle
file imported, under ../node_modules/react-native-ffmpeg/android
path.
On IOS, specify a different version for mobile-ffmpeg
dependency inside imported .podspec
file under ../node_modules/react-native-ffmpeg/ios
path.
Main Release | LTS Release | |
---|---|---|
Android API Level | 24 | 21 |
Android Camera Access | x | - |
Android Architectures | arm-v7a-neon arm64-v8a x86 x86-64 | arm-v7a arm-v7a-neon arm64-v8a x86 x86-64 |
IOS SDK | 12.1 | 9.3 |
Xcode Support | 10.1 | 7.3.1 |
IOS Architectures | arm64 arm64e x86-64 | armv7 arm64 i386 x86-64 |
Apply provided solutions if you encounter one of the following issues.
You should not use double quotes (") to define your complex filters or map definitions.
-filter_complex [0:v]scale=1280:-1[v] -map [v]
If your commands include unnecessary quotes or space characters your command will fail with No such filter: ' '
errors. Please check your command and remove them.
Sometimes react-native run-ios
fails with weird build errors. Execute the following commands and try again.
rm -rf ios/Pods ios/build ios/Podfile.lock
cd ios
pod install
Add "postinstall": "sed -i '' 's\/#import <RCTAnimation\\/RCTValueAnimatedNode.h>\/#import \"RCTValueAnimatedNode.h\"\/' ./node_modules/react-native/Libraries/NativeAnimation/RCTNativeAnimatedNodesManager.h"
line to the scripts section of your package.json as recommended in react-native issue # 13198,
if your build receives the following error for IOS.
../node_modules/react-native/Libraries/NativeAnimation/RCTNativeAnimatedNodesManager.h:10:9: fatal error: 'RCTAnimation/RCTValueAnimatedNode.h' file not found
#import <RCTAnimation/RCTValueAnimatedNode.h>
^~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
1 error generated.
When pod install
is not successful with the following message, delete Podfile.lock
file and run pod install
again.
[!] Unable to find a specification for 'react-native-ffmpeg'.
If react-native link
is used for IOS linking, building may fail with this error. Running pod install
again
fixes this issue.
../node_modules/react-native-ffmpeg/ios/Pods/Target Support Files/Pods-ReactNativeFFmpeg/Pods-ReactNativeFFmpeg.debug.xcconfig: unable to open file (in target "ReactNativeFFmpeg" in project "ReactNativeFFmpeg") (in target 'ReactNativeFFmpeg')
Using cocoapods
for IOS dependency management may produce duplicate symbols for libReact.a
and libyoga.a
.
Add the following block to your Podfile
and run pod install
again.
post_install do |installer|
installer.pods_project.targets.each do |target|
targets_to_ignore = %w(React yoga)
if targets_to_ignore.include? target.name
target.remove_from_project
end
end
end
You can see how React Native FFmpeg
is used inside an application by running test applications provided under react-native-ffmpeg-test repository. All applications provide the same functionality; performs command execution and video encoding operations. The difference between them is IOS dependency management mechanism applied.
Refer to Changelog for updates.
This project is licensed under the LGPL v3.0. However, if installation is customized to use a package with -gpl
postfix (min-gpl, https-gpl, full-gpl) then React Native FFmpeg
is subject to the GPL v3.0 license.
Digital assets used in test applications are published in the public domain.
Feel free to submit issues or pull requests.
0.3.1 - 2019-01-31
FAQs
FFmpeg for React Native
The npm package react-native-ffmpeg receives a total of 295 weekly downloads. As such, react-native-ffmpeg popularity was classified as not popular.
We found that react-native-ffmpeg demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.