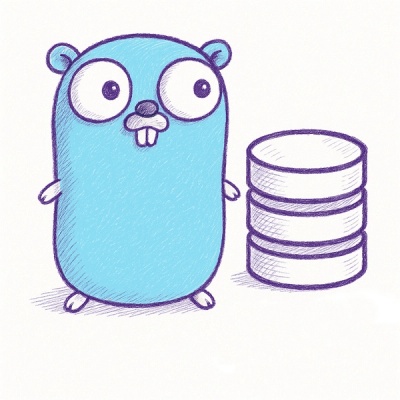
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
react-native-flipper-databases
Advanced tools
Flipper Databases plugin for React Native
This React Native plugin allows browsing popular React Native databases using Flipper built-in Databases plugin.
yarn add -D react-native-flipper react-native-flipper-databases
No particular setup is required on iOS.
Since Android already provide a built-in Databases plugin (see official docs here for more details) in order to avoid conflicts with react-native-flipper-databases
it must be disabled.
Edit ReactNativeFlipper.java
file under android/app/src/debug/java/<your-app-package>
like this
...
import com.facebook.flipper.plugins.crashreporter.CrashReporterPlugin;
// import com.facebook.flipper.plugins.databases.DatabasesFlipperPlugin; // <- Exclude to avoid plugin conflicts
import com.facebook.flipper.plugins.fresco.FrescoFlipperPlugin;
import com.facebook.flipper.plugins.inspector.DescriptorMapping;
import com.facebook.flipper.plugins.inspector.InspectorFlipperPlugin;
import com.facebook.flipper.plugins.network.FlipperOkhttpInterceptor;
import com.facebook.flipper.plugins.network.NetworkFlipperPlugin;
import com.facebook.flipper.plugins.react.ReactFlipperPlugin;
import com.facebook.flipper.plugins.sharedpreferences.SharedPreferencesFlipperPlugin;
...
public static void initializeFlipper(Context context, ReactInstanceManager reactInstanceManager) {
if (FlipperUtils.shouldEnableFlipper(context)) {
final FlipperClient client = AndroidFlipperClient.getInstance(context);
client.addPlugin(new InspectorFlipperPlugin(context, DescriptorMapping.withDefaults()));
client.addPlugin(new ReactFlipperPlugin());
// client.addPlugin(new DatabasesFlipperPlugin(context)); // <- Exclude to avoid plugin conflicts
client.addPlugin(new SharedPreferencesFlipperPlugin(context));
client.addPlugin(CrashReporterPlugin.getInstance());
...
See facebook/flipper#1628 for more details.
When sticking to a managed Expo project, it's impossible to make the necessary modifications to the ReactNativeFlipper.java
file.
@liamdawson wrote a basic plugin to automate those changes, which will ensure Expo prebuild and builds via EAS will disable the integrated Databases plugin on Android.
See @liamdawson/disable-react-native-flipper-databases-expo-plugin for more info.
WatermelonDB Version | Use Version |
---|---|
>=0.24.0 | 2.x |
<0.24.0 | 1.x |
Attach a WatermelonDB database:
// ...
/// ReactNativeFlipperDatabases - START
if (__DEV__) {
// Import connectDatabases function and required DBDrivers
const { connectDatabases, WatermelonDB } = require('react-native-flipper-databases');
connectDatabases([
new WatermelonDB(database), // Pass in database definition
]);
}
/// ReactNativeFlipperDatabases - END
// ...
Attach an open Realm:
// ...
const realm = await Realm.open(config);
/// FlipperDatabasesPlugin - START
if (__DEV__) {
// Import connectDatabases function and required DBDrivers
const { connectDatabases, RealmDB } = require('react-native-flipper-databases');
connectDatabases([
new RealmDB('Realm', realm), // Pass in a realm name and an open realm reference
]);
}
/// FlipperDatabasesPlugin - END
// ...
Attach an open PouchDB database:
// ...
const db = new PouchDB('db', {
adapter: 'react-native-sqlite',
});
/// ReactNativeFlipperDatabases - START
if (__DEV__) {
// Import connectDatabases function and required DBDrivers
const {
connectDatabases,
PouchDB: PouchDBDriver,
} = require('react-native-flipper-databases');
connectDatabases([
new PouchDBDriver([db]), // Pass in database definitions
]);
}
/// ReactNativeFlipperDatabases - END
// ...
Attach an open Vasern database:
// ...
export const VasernDB = new Vasern({
// Vasern config
});
/// ReactNativeFlipperDatabases - START
if (__DEV__) {
// Import connectDatabases function and required DBDrivers
const {
connectDatabases,
VasernDB: VasernDBDriver,
} = require('react-native-flipper-databases');
connectDatabases([
new VasernDBDriver(VasernDB), // Pass in database definitions
]);
}
/// ReactNativeFlipperDatabases - END
// ...
Attach an open SQLite database (with Promise support enabled)
// ...
SQLite.enablePromise(true);
async function openDatabase() {
const db = await SQLite.openDatabase({ name: 'data.db' });
// Create tables
/// ReactNativeFlipperDatabases - START
if (__DEV__) {
// Import connectDatabases function and required DBDrivers
const { connectDatabases, SQLiteStorage } = require('react-native-flipper-databases');
connectDatabases([
// Pass in database definitions
new SQLiteStorage([
{
name: 'data.db',
database: db,
},
]),
]);
}
/// ReactNativeFlipperDatabases - END
return db;
}
// ...
import { openDatabase } from 'react-native-quick-sqlite'
// ...
/// ReactNativeFlipperDatabases - START
if (__DEV__) {
// Import connectDatabases function and required DBDrivers
const {
connectDatabases,
QuickSQLiteStorage,
} = require('react-native-flipper-databases');
openDatabase(
{ name: 'data.db' },
(db) => {
connectDatabases([
new QuickSQLiteStorage([
{
name: 'data.db',
database: db,
},
]),
])
},
() => {},
)
}
/// ReactNativeFlipperDatabases - END
// ...
To see how to implement this plugin and test how it works some examples are provided.
To run the examples:
git clone https://codeberg.org/panz3r/react-native-flipper-databases.git
yarn bootstrap
launch one of the following scripts from the root folder
example:watermelon
to launch the WatermelonDB
example appexample:realm
to launch the MongoDB Realm
example appexample:pouch
to launch the PouchDB
example appexample:vasern
to launch the Vasern
example appexample:sqlitestorage
to launch the SQLite Storage
example appThe plugin integrations are located inside the src/infrastructure/database
folder of each example app.
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
Made with :sparkles: & :heart: by Mattia Panzeri and contributors
FAQs
Flipper Databases plugin for React Native
The npm package react-native-flipper-databases receives a total of 36 weekly downloads. As such, react-native-flipper-databases popularity was classified as not popular.
We found that react-native-flipper-databases demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.