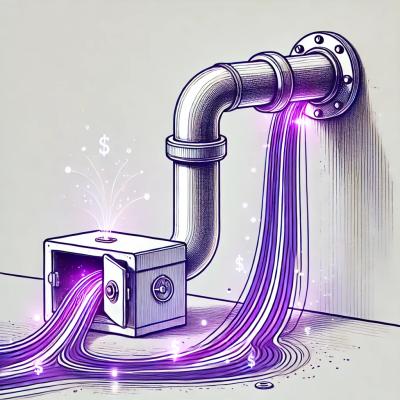
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
react-native-google-places-x
Advanced tools
iOS/Android Google Places Widgets (Autocomplete, Place Picker) and API Services for React Native Apps
iOS/Android Google Places Widgets (Autocomplete, Place Picker) and API Services for React Native Apps
npm i react-native-google-places --save
react-native link react-native-google-places
OR
yarn add react-native-google-places
react-native link react-native-google-places
react-native link react-native-google-places
. Or you can run the command now if you have not already.Libraries ➜ Add Files to [your project's name]
.node_modules
➜ react-native-google-places
and add RNGooglePlaces.xcodeproj
.libRNGooglePlaces.a
to your project's Build Phases
➜ Link Binary With Libraries
.gem install cocoapods
to set it up the first time. (Hint: Go grab a cup of coffee!)cd ios && pod init
at the root directory of your project.pod 'GooglePlaces'
, pod 'GooglePlacePicker'
and pod 'GoogleMaps'
to your Podfile. Otherwise just edit your Podfile to include:source 'https://github.com/CocoaPods/Specs.git'
target 'YOUR_APP_TARGET_NAME' do
pod 'GooglePlaces'
pod 'GoogleMaps'
pod 'GooglePlacePicker'
end
@import GooglePlaces;
and @import GoogleMaps;
on top of the file.didFinishLaunchingWithOptions
method, instantiate the library as follows:[GMSPlacesClient provideAPIKey:@"YOUR_IOS_API_KEY_HERE"];
[GMSServices provideAPIKey:@"YOUR_IOS_API_KEY_HERE"];
pod install
from your ios
directory..xcworkspace
file to open the project. Or just use the react-native run-ios
command as usual to run your app in the simulator.<application>
tag as follows:<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<application
android:name=".MainApplication"
...>
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_ANDROID_API_KEY_HERE"/>
...
</application>
react-native link react-native-google-places
. Otherwise, do the following or just ensure they are in place;android/settings.gradle
file:include ':react-native-google-places'
project(':react-native-google-places').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-google-places/android')
android/app/build.grade
file:dependencies {
...
compile project(':react-native-google-places')
}
...MainApplication.java
file:import com.arttitude360.reactnative.rngoogleplaces.RNGooglePlacesPackage;
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
...
new RNGooglePlacesPackage() //<-- Add line
);
}
react-native run-android
to get started.import RNGooglePlaces from 'react-native-google-places';
class GPlacesDemo extends Component {
openSearchModal() {
RNGooglePlaces.openAutocompleteModal()
.then((place) => {
console.log(place);
// place represents user's selection from the
// suggestions and it is a simplified Google Place object.
})
.catch(error => console.log(error.message)); // error is a Javascript Error object
}
render() {
return (
<View style={styles.container}>
<TouchableOpacity
style={styles.button}
onPress={() => this.openSearchModal()}
>
<Text>Pick a Place</Text>
</TouchableOpacity>
</View>
);
}
}
To filter autocomplete results as listed for Android and iOS in the official docs, you can pass an options
object as a parameter to the openAutocompleteModal()
method as follows:
RNGooglePlaces.openAutocompleteModal({
type: 'establishment',
country: 'CA',
latitude: 53.544389,
longitude: -113.490927,
radius: 10
})
.then((place) => {
console.log(place);
})
.catch(error => console.log(error.message));
type
(String) - The type of results to return. Can be one of (geocode
, address
, establishment
, regions
, and cities
). (optional)country
(String) - Limit results to a specific country using a ISO 3166-1 Alpha-2 country code (case insensitive). If this is not set, no country filtering will take place. (optional)latitude
(Number) - Latitude of the point around which you wish to retrieve place information (required if longitude
is given)longitude
(Number) - Longitude of the point around which you wish to retrieve place information (required if latitude
is given)radius
(Number) - Radius (in kilo-meters) within which to retrieve place information. Only works if latitude
and longitude
are also given. Note that setting a radius biases results to the indicated area, but may not fully restrict results to the specified area. Defaults to 0.1
.useOverlay
(Boolean) [Android Only] - If true, the autocomplete modal will open as an overlay. Defaults to false
.class GPlacesDemo extends Component {
openSearchModal() {
RNGooglePlaces.openPlacePickerModal()
.then((place) => {
console.log(place);
// place represents user's selection from the
// suggestions and it is a simplified Google Place object.
})
.catch(error => console.log(error.message)); // error is a Javascript Error object
}
render() {
return (
<View style={styles.container}>
<TouchableOpacity
style={styles.button}
onPress={() => this.openSearchModal()}
>
<Text>Open Place Picker</Text>
</TouchableOpacity>
</View>
);
}
}
To set the initial viewport that the place picker map should show when the picker is launched, you can pass a latLngBounds
object as a parameter
to the openPlacePickerModal()
method as follows. The latLngBounds
object takes the following optional keys:
latitude
(Number) - Latitude of the point which you want the map centered on (required if longitude
is given)longitude
(Number) - Longitude of the point which you want the map centered on (required if latitude
is given)radius
(Number) - Radius (in kilo-meters) from the center of the map view to the edge. Use this to set the default "zoom" of the map view when it is first opened. Only works if latitude
and longitude
are also given. Defaults to 0.1
.If no initial viewport is set (no argument is passed to the openPlacePickerModal()
method), the viewport will be centered on the device's location, with the zoom at city-block level.
RNGooglePlaces.openPlacePickerModal({
latitude: 53.544389,
longitude: -113.490927,
radius: 0.01 // 10 meters
})
.then((place) => {
console.log(place);
})
.catch(error => console.log(error.message));
{
placeID: "ChIJZa6ezJa8j4AR1p1nTSaRtuQ",
website: "https://www.facebook.com/",
phoneNumber: "+1 650-543-4800",
address: "1 Hacker Way, Menlo Park, CA 94025, USA",
name: "Facebook HQ",
types: [ 'street_address', 'geocode' ],
latitude: 37.4843428,
longitude: -122.14839939999999
}
Promise
from calling RNGooglePlaces.openAutocompleteModal()
are dependent on the selected place - as phoneNumber, website, north, south, east, west, priceLevel, rating
are not set on all Google Place
objects.This method returns to you the place where the device is currently located. That is, the place at the device's currently-reported location. For each place, the result includes an indication of the likelihood that the place is the right one. A higher value for likelihood
means a greater probability that the place is the best match.
RNGooglePlaces.getCurrentPlace()
.then((results) => console.log(results))
.catch((error) => console.log(error.message));
[{ name: 'Facebook HQ',
website: 'https://www.facebook.com/',
longitude: -122.14835169999999,
address: '1 Hacker Way, Menlo Park, CA 94025, USA',
latitude: 37.48485,
placeID: 'ChIJZa6ezJa8j4AR1p1nTSaRtuQ',
types: [ 'street_address', 'geocode' ],
phoneNumber: '+1 650-543-4800',
likelihood: 0.9663974,
...
},{
...
}]
This method returns photo and save into your storage and return that url located.
RNGooglePlaces.lookUpPhotosForPlaceID(placeID)
.then((results) => console.log(results))
.catch((error) => console.log(error.message));
[{
url: 'user/sfa/sdf/a/sfd/a.png',
size: {
width: 300,
height: 200
}
...
},{
...
}]
The sum of the likelihoods in a given result set is always less than or equal to 1.0. Note that the sum isn't necessarily 1.0.
If you have specific branding needs or you would rather build out your own custom search input and suggestions list (think Uber
), you may profit from calling the API methods below which would get you autocomplete predictions programmatically using the underlying iOS and Android SDKs
.
RNGooglePlaces.getAutocompletePredictions('facebook')
.then((results) => this.setState({ predictions: results }))
.catch((error) => console.log(error.message));
To filter autocomplete results as listed for Android and iOS in the official docs, you can pass an options
object as a second parameter to the getAutocompletePredictions()
method as follows:
RNGooglePlaces.getAutocompletePredictions('Lagos', {
type: 'cities',
country: 'NG'
})
.then((place) => {
console.log(place);
})
.catch(error => console.log(error.message));
OR
RNGooglePlaces.getAutocompletePredictions('pizza', {
type: 'establishments',
latitude: 53.544389,
longitude: -113.490927,
radius: 10
})
.then((place) => {
console.log(place);
})
.catch(error => console.log(error.message));
type
(String) - The type of results to return. Can be one of (geocode
, address
, establishment
, regions
, and cities
). (optional)country
(String) - Limit results to a specific country using a ISO 3166-1 Alpha-2 country code (case insensitive). If this is not set, no country filtering will take place. (optional)latitude
(Number) - Latitude of the point around which you wish to retrieve place information (required if longitude
is given)longitude
(Number) - Longitude of the point around which you wish to retrieve place information (required if latitude
is given)radius
(Number) - Radius (in kilo-meters) within which to retrieve place information. Only works if latitude
and longitude
are also given. Note that setting a radius biases results to the indicated area, but may not fully restrict results to the specified area. Defaults to 0.1
.[ { primaryText: 'Facebook HQ',
placeID: 'ChIJZa6ezJa8j4AR1p1nTSaRtuQ',
secondaryText: 'Hacker Way, Menlo Park, CA, United States',
fullText: 'Facebook HQ, Hacker Way, Menlo Park, CA, United States' },
types: [ 'street_address', 'geocode' ],
{ primaryText: 'Facebook Way',
placeID: 'EitGYWNlYm9vayBXYXksIE1lbmxvIFBhcmssIENBLCBVbml0ZWQgU3RhdGVz',
secondaryText: 'Menlo Park, CA, United States',
fullText: 'Facebook Way, Menlo Park, CA, United States' },
types: [ 'street_address', 'geocode' ],
...
]
RNGooglePlaces.lookUpPlaceByID('ChIJZa6ezJa8j4AR1p1nTSaRtuQ')
.then((results) => console.log(results))
.catch((error) => console.log(error.message));
{ name: 'Facebook HQ',
website: 'https://www.facebook.com/',
longitude: -122.14835169999999,
address: '1 Hacker Way, Menlo Park, CA 94025, USA',
latitude: 37.48485,
placeID: 'ChIJZa6ezJa8j4AR1p1nTSaRtuQ',
types: [ 'street_address', 'geocode' ],
phoneNumber: '+1 650-543-4800',
}
The typical use flow would be to call getAutocompletePredictions()
when the value of your search input changes to populate your suggestion listview and call lookUpPlaceByID()
to retrieve the place details when a place on your listview is selected.
getAutocompletePredictions()
method is subject to tiered query limits. See the documentation on Android & iOS Usage Limits.You have to link dependencies and re-run the build:
react-native link
Manual Linking With Your Project
steps above.react-native run-ios
Extras / Google Play services
Extras / Google Repository
Android (API 23+) / Google APIs Intel x86 Atom System Image Rev. 13
Check manual installation steps
Ensure your API key has permissions for Google Place
and Google Android Maps
If you have a different version of play serivces than the one included in this library (which is currently at 10.2.4), use the following instead (switch 10.2.0 for the desired version) in your android/app/build.grade
file:
...
dependencies {
...
compile(project(':react-native-google-places')){
exclude group: 'com.google.android.gms', module: 'play-services-base'
exclude group: 'com.google.android.gms', module: 'play-services-places'
exclude group: 'com.google.android.gms', module: 'play-services-location'
}
compile 'com.google.android.gms:play-services-base:10.2.0'
compile 'com.google.android.gms:play-services-places:10.2.0'
compile 'com.google.android.gms:play-services-location:10.2.0'
}
The MIT License.
FAQs
iOS/Android Google Places Widgets (Autocomplete, Place Picker) and API Services for React Native Apps
The npm package react-native-google-places-x receives a total of 4 weekly downloads. As such, react-native-google-places-x popularity was classified as not popular.
We found that react-native-google-places-x demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.