react-native-keyboard-spacer
Advanced tools
Comparing version 0.2.0 to 0.3.0
/** | ||
* Created by andrewhurst on 10/5/15. | ||
*/ | ||
var React = require('react'); | ||
var ReactNative = require('react-native'); | ||
import React, { Component, PropTypes } from 'react'; | ||
import { | ||
Keyboard, | ||
LayoutAnimation, | ||
View, | ||
Platform, | ||
StyleSheet | ||
} from 'react-native'; | ||
var { | ||
DeviceEventEmitter, | ||
LayoutAnimation, | ||
View, | ||
Platform | ||
} = ReactNative; | ||
const styles = StyleSheet.create({ | ||
container: { | ||
left: 0, | ||
right: 0, | ||
bottom: 0, | ||
}, | ||
}); | ||
// From: https://medium.com/man-moon/writing-modern-react-native-ui-e317ff956f02 | ||
const defaultAnimation = { | ||
duration: 500, | ||
create: { | ||
export default class KeyboardSpacer extends Component { | ||
static propTypes = { | ||
topSpacing: PropTypes.number, | ||
onToggle: PropTypes.func, | ||
style: View.propTypes.style, | ||
animationConfig: PropTypes.object, | ||
}; | ||
static defaultProps = { | ||
topSpacing: 0, | ||
// From: https://medium.com/man-moon/writing-modern-react-native-ui-e317ff956f02 | ||
animationConfig: { | ||
duration: 500, | ||
create: { | ||
duration: 300, | ||
type: LayoutAnimation.Types.easeInEaseOut, | ||
property: LayoutAnimation.Properties.opacity | ||
}, | ||
update: { | ||
}, | ||
update: { | ||
type: LayoutAnimation.Types.spring, | ||
springDamping: 200 | ||
} | ||
}; | ||
} | ||
}, | ||
onToggle: () => null, | ||
}; | ||
class KeyboardSpacer extends React.Component { | ||
constructor(props, context) { | ||
super(props, context); | ||
this.state = { | ||
keyboardSpace: 0, | ||
isKeyboardOpened: false | ||
}; | ||
constructor(props, context) { | ||
super(props, context); | ||
this.state = { | ||
keyboardSpace: 0, | ||
isKeyboardOpened: false | ||
}; | ||
this._listeners = null; | ||
this.updateKeyboardSpace = this.updateKeyboardSpace.bind(this); | ||
this.resetKeyboardSpace = this.resetKeyboardSpace.bind(this); | ||
} | ||
this.updateKeyboardSpace = this.updateKeyboardSpace.bind(this); | ||
this.resetKeyboardSpace = this.resetKeyboardSpace.bind(this); | ||
} | ||
componentDidMount() { | ||
const updateListener = Platform.OS === 'android' ? 'keyboardDidShow' : 'keyboardWillShow'; | ||
const resetListener = Platform.OS === 'android' ? 'keyboardDidHide' : 'keyboardWillHide'; | ||
this._listeners = [ | ||
Keyboard.addListener(updateListener, this.updateKeyboardSpace), | ||
Keyboard.addListener(resetListener, this.resetKeyboardSpace) | ||
]; | ||
} | ||
componentWillUpdate(props, state) { | ||
if (state.isKeyboardOpened !== this.state.isKeyboardOpened) { | ||
LayoutAnimation.configureNext(props.animationConfig || defaultAnimation); | ||
} | ||
componentWillUpdate(props, state) { | ||
if (state.isKeyboardOpened !== this.state.isKeyboardOpened) { | ||
LayoutAnimation.configureNext(props.animationConfig); | ||
} | ||
} | ||
updateKeyboardSpace(frames) { | ||
if (!frames.endCoordinates) | ||
return; | ||
componentWillUnmount() { | ||
this._listeners.forEach(listener => listener.remove()); | ||
} | ||
var keyboardSpace = frames.endCoordinates.height + ('topSpacing' in this.props ? this.props.topSpacing : 0); | ||
this.setState({ | ||
keyboardSpace: keyboardSpace, | ||
isKeyboardOpened: true | ||
}, () => ('onToggle' in this.props ? this.props.onToggle(true, keyboardSpace) : null)); | ||
updateKeyboardSpace(frames) { | ||
if (!frames.endCoordinates) { | ||
return; | ||
} | ||
const keyboardSpace = frames.endCoordinates.height + this.props.topSpacing; | ||
this.setState({ | ||
keyboardSpace, | ||
isKeyboardOpened: true | ||
}, this.props.onToggle(true, keyboardSpace)); | ||
} | ||
resetKeyboardSpace() { | ||
this.setState({ | ||
keyboardSpace: 0, | ||
isKeyboardOpened: false | ||
}, () => ('onToggle' in this.props ? this.props.onToggle(false, 0) : null)); | ||
} | ||
resetKeyboardSpace() { | ||
this.setState({ | ||
keyboardSpace: 0, | ||
isKeyboardOpened: false | ||
}, this.props.onToggle(false, 0)); | ||
} | ||
componentDidMount() { | ||
if (Platform.OS == "android") { | ||
this._listeners = [ | ||
DeviceEventEmitter.addListener('keyboardDidShow', this.updateKeyboardSpace), | ||
DeviceEventEmitter.addListener('keyboardDidHide', this.resetKeyboardSpace) | ||
]; | ||
} else { | ||
this._listeners = [ | ||
DeviceEventEmitter.addListener('keyboardWillShow', this.updateKeyboardSpace), | ||
DeviceEventEmitter.addListener('keyboardWillHide', this.resetKeyboardSpace) | ||
]; | ||
} | ||
} | ||
componentWillUnmount() { | ||
this._listeners.forEach(function(/** EmitterSubscription */listener) { | ||
listener.remove(); | ||
}); | ||
} | ||
render() { | ||
return (<View style={[{height: this.state.keyboardSpace, left: 0, right: 0, bottom: 0}, this.props.style]}/>); | ||
} | ||
render() { | ||
return ( | ||
<View style={[styles.container, { height: this.state.keyboardSpace }, this.props.style]} />); | ||
} | ||
} | ||
module.exports = KeyboardSpacer; |
{ | ||
"name": "react-native-keyboard-spacer", | ||
"version": "0.2.0", | ||
"version": "0.3.0", | ||
"description": "Plug and play react-Native keyboard spacer view.", | ||
@@ -5,0 +5,0 @@ "main": "KeyboardSpacer.js", |
@@ -7,3 +7,3 @@ [](https://nodei.co/npm/react-native-keyboard-spacer/) | ||
 | ||
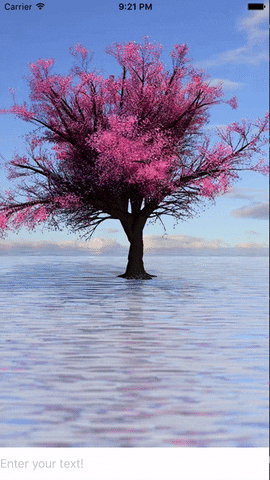 | ||
## Quick Start | ||
@@ -18,5 +18,5 @@ | ||
```javascript | ||
var React = require('react-native'); | ||
var KeyboardSpacer = require('react-native-keyboard-spacer'); | ||
var { | ||
import KeyboardSpacer from 'react-native-keyboard-spacer'; | ||
import React, { Component } from 'react'; | ||
import { | ||
AppRegistry, | ||
@@ -27,6 +27,6 @@ StyleSheet, | ||
TextInput | ||
} = React; | ||
} from 'react-native'; | ||
var DemoApp = React.createClass({ | ||
render: function() { | ||
class DemoApp extends Component { | ||
render() { | ||
return ( | ||
@@ -36,7 +36,7 @@ <View style={[{flex: 1}]}> | ||
<Image source={{uri: 'http://img11.deviantart.net/072b/i/2011/206/7/0/the_ocean_cherry_tree_by_tomcadogan-d41nzsz.png', static: true}} | ||
style={{flex: 1}}/> | ||
style={{flex: 1}}/> | ||
{/* The text input to put on top of the keyboard */} | ||
<TextInput style={{left: 0, right: 0, height: 45}} | ||
placeholder={'Enter your text!'}/> | ||
placeholder={'Enter your text!'}/> | ||
@@ -48,3 +48,3 @@ {/* The view that will animate to match the keyboards height */} | ||
} | ||
}); | ||
} | ||
@@ -51,0 +51,0 @@ AppRegistry.registerComponent('DemoApp', () => DemoApp); |
89
6563