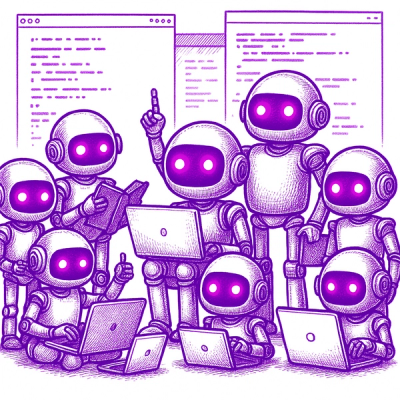
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
react-native-keychain
Advanced tools
The react-native-keychain package provides a secure way to store and retrieve sensitive information such as passwords, tokens, and other credentials in a React Native application. It leverages the native keychain services on iOS and the Keystore system on Android to ensure data is stored securely.
Storing Credentials
This feature allows you to securely store a username and password in the device's keychain or keystore.
import * as Keychain from 'react-native-keychain';
async function saveCredentials(username, password) {
await Keychain.setGenericPassword(username, password);
}
Retrieving Credentials
This feature allows you to retrieve stored credentials from the device's keychain or keystore.
import * as Keychain from 'react-native-keychain';
async function getCredentials() {
const credentials = await Keychain.getGenericPassword();
if (credentials) {
console.log('Credentials successfully loaded for user ' + credentials.username);
} else {
console.log('No credentials stored');
}
}
Resetting Credentials
This feature allows you to reset or delete the stored credentials from the device's keychain or keystore.
import * as Keychain from 'react-native-keychain';
async function resetCredentials() {
await Keychain.resetGenericPassword();
}
Storing Internet Credentials
This feature allows you to securely store internet credentials (e.g., server, username, password) in the device's keychain or keystore.
import * as Keychain from 'react-native-keychain';
async function saveInternetCredentials(server, username, password) {
await Keychain.setInternetCredentials(server, username, password);
}
Retrieving Internet Credentials
This feature allows you to retrieve stored internet credentials from the device's keychain or keystore.
import * as Keychain from 'react-native-keychain';
async function getInternetCredentials(server) {
const credentials = await Keychain.getInternetCredentials(server);
if (credentials) {
console.log('Internet credentials successfully loaded for server ' + server);
} else {
console.log('No internet credentials stored for server ' + server);
}
}
react-native-sensitive-info is another package that provides secure storage for sensitive information in React Native applications. It supports storing data in the Android Keystore and iOS Keychain, similar to react-native-keychain. However, it also offers additional features like encryption and the ability to store data in shared preferences or user defaults.
react-native-secure-storage is a package that provides secure storage for sensitive data in React Native applications. It uses the Android Keystore and iOS Keychain for secure storage, similar to react-native-keychain. It also supports encryption and offers a simple API for storing and retrieving data.
react-native-encrypted-storage is a package that provides secure and encrypted storage for sensitive data in React Native applications. It uses the Android Keystore and iOS Keychain for secure storage, similar to react-native-keychain. It also offers encryption and decryption of data, ensuring that sensitive information is stored securely.
This library provides access to the Keychain (iOS) and Keystore (Android) for securely storing credentials like passwords, tokens, or other sensitive information in React Native apps.
yarn add react-native-keychain
pod install
in ios/
directory to install iOS dependencies.NSFaceIDUsageDescription
entry in your Info.plist
.Please refer to the documentation website on https://oblador.github.io/react-native-keychain
Check the GitHub Releases page.
![]() Joel Arvidsson Author |
![]() Dorian Mazur Maintainer |
![]() Vojtech Novak Maintainer |
![]() Pelle Stenild Coltau Maintainer |
![]() Oleksandr Kucherenko Contributor |
This library is used by several projects, including:
MIT © Joel Arvidsson 2016-2020
FAQs
Keychain Access for React Native
The npm package react-native-keychain receives a total of 175,041 weekly downloads. As such, react-native-keychain popularity was classified as popular.
We found that react-native-keychain demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.