What is react-native-localize?
The react-native-localize package provides localization utilities for React Native applications. It helps in handling various localization tasks such as determining the user's locale, timezone, and country, as well as formatting numbers, dates, and times according to the user's locale.
What are react-native-localize's main functionalities?
Get User's Locale
This feature allows you to get the list of locales preferred by the user. The code sample retrieves the user's preferred locales and logs them to the console.
const { getLocales } = require('react-native-localize');
const locales = getLocales();
console.log(locales);
Get User's Timezone
This feature allows you to get the user's current timezone. The code sample retrieves the user's timezone and logs it to the console.
const { getTimeZone } = require('react-native-localize');
const timeZone = getTimeZone();
console.log(timeZone);
Get User's Country
This feature allows you to get the user's current country. The code sample retrieves the user's country and logs it to the console.
const { getCountry } = require('react-native-localize');
const country = getCountry();
console.log(country);
Format Number
This feature allows you to get the number formatting settings for the user's locale. The code sample retrieves the decimal and grouping separators and logs them to the console.
const { getNumberFormatSettings } = require('react-native-localize');
const { decimalSeparator, groupingSeparator } = getNumberFormatSettings();
console.log(`Decimal Separator: ${decimalSeparator}, Grouping Separator: ${groupingSeparator}`);
Handle Localization Changes
This feature allows you to handle changes in localization settings. The code sample adds an event listener that logs a message when localization settings change.
const { addEventListener, removeEventListener } = require('react-native-localize');
const handleLocalizationChange = () => {
console.log('Localization settings changed');
};
addEventListener('change', handleLocalizationChange);
// To remove the listener
// removeEventListener('change', handleLocalizationChange);
Other packages similar to react-native-localize
i18next
i18next is a popular internationalization framework for JavaScript that provides a complete solution for localizing your app. It supports multiple languages, pluralization, and interpolation. Compared to react-native-localize, i18next offers more comprehensive internationalization features but requires more setup.
react-intl
react-intl is a library for internationalizing React applications. It provides components and an API to format dates, numbers, and strings, and to handle pluralization. While react-intl focuses on formatting and message handling, react-native-localize provides more utilities for determining the user's locale and timezone.
expo-localization
expo-localization is a package from the Expo ecosystem that provides localization utilities similar to react-native-localize. It can determine the user's locale, timezone, and country. However, expo-localization is designed to work seamlessly with Expo projects, whereas react-native-localize can be used in any React Native project.
🌍 react-native-localize
A toolbox for your React Native app localization.

⚠️ Breaking change
This project was known as react-native-languages and has been renamed to reflect new APIs possibilities.
Find more informations about this change here.
Support
react-native-localize | 1.0.0+ | 0.56.0+ |
react-native-languages | 2.0.1 | 0.48.0 - 0.55.4 |
Setup
$ npm install --save react-native-localize
$ yarn add react-native-localize
Linking
Using react-native-cli (recommended)
$ react-native link react-native-localize
Manual (iOS)
- In the XCode's "Project navigator", right click on your project's Libraries folder ➜
Add Files to <...>
- Go to
node_modules
➜ react-native-localize
➜ ios
➜ select RNLocalize.xcodeproj
- Add
RNLocalize.a
to Build Phases -> Link Binary With Libraries
Manual (Android)
- Add the following lines to
android/settings.gradle
:
include ':react-native-localize'
project(':react-native-localize').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-localize/android')
- Add the compile line to the dependencies in
android/app/build.gradle
:
dependencies {
// ...
implementation project(':react-native-localize')
}
- Add the import and link the package in
MainApplication.java
:
import com.reactcommunity.rnlocalize.RNLocalizePackage;
public class MainApplication extends Application implements ReactApplication {
@Override
protected List<ReactPackage> getPackages() {
return Arrays.<ReactPackage>asList(
new MainReactPackage(),
new RNLocalizePackage()
);
}
}
Basic usage example
import * as RNLocalize from "react-native-localize";
console.log(RNLocalize.getLocales());
console.log(RNLocalize.getCurrencies());
RNLocalize.addEventListener("change", () => {
});
API
getLocales()
Returns the user preferred locales, in order.
Method type
type getLocales = () => Array<{
languageCode: string;
scriptCode?: string;
countryCode: string;
languageTag: string;
isRTL: boolean;
}>;
Usage example
console.log(RNLocalize.getLocales());
getNumberFormatSettings()
Returns number formatting settings.
Method type
type getNumberFormatSettings = () => {
decimalSeparator: string;
groupingSeparator: string;
};
Usage example
console.log(RNLocalize.getNumberFormatSettings());
getCurrencies()
Returns the user preferred currency codes, in order.
Method type
type getCurrencies = () => Array<string>;
Usage example
console.log(RNLocalize.getCurrencies());
getCountry()
Returns the user current country code (based on its device locale, not on its position).
Method type
type getCountry = () => string;
Usage example
console.log(RNLocalize.getCountry());
getCalendar()
Returns the user preferred calendar format.
Method type
type getCalendar = () => "gregorian" | "japanese" | "buddhist";
Usage example
console.log(RNLocalize.getCalendar());
getTemperatureUnit()
Returns the user preferred temperature unit.
Method type
type getTemperatureUnit = () => "celsius" | "fahrenheit";
Usage example
console.log(RNLocalize.getTemperatureUnit());
getTimeZone()
Returns the user preferred timezone (based on its device settings, not on its position).
Method type
type getTimeZone = () => string;
Usage example
console.log(RNLocalize.getTimeZone());
uses24HourClock()
Returns true
if the user prefers 24h clock format, false
if he prefers 12h clock format.
Method type
type uses24HourClock = () => boolean;
Usage example
console.log(RNLocalize.uses24HourClock());
usesMetricSystem()
Returns true
if the user prefers metric measure system, false
if he prefers imperial.
Method type
type usesMetricSystem = () => boolean;
Usage example
console.log(RNLocalize.usesMetricSystem());
addEventListener() / removeEventListener()
Allows you to listen for any localization change.
Methods type
type addEventListener = (type: "change", handler: Function) => void;
type removeEventListener = (type: "change", handler: Function) => void;
Usage example
function handleLocalizationChange() {
console.log(RNLocalize.getLocales());
}
RNLocalize.addEventListener("change", handleLocalizationChange);
RNLocalize.removeEventListener("change", handleLocalizationChange);
findBestAvailableLanguage()
Returns the best language tag possible and its direction (if it can find one). Useful to choose the best translation available.
Method type
type findBestAvailableLanguage = (
languageTags: Array<string>,
) => { languageTag: string; isRTL: boolean } | void;
Usage example
console.log(RNLocalize.findBestAvailableLanguage(["en-US", "en", "fr"]));
Add project's supported localizations (iOS)
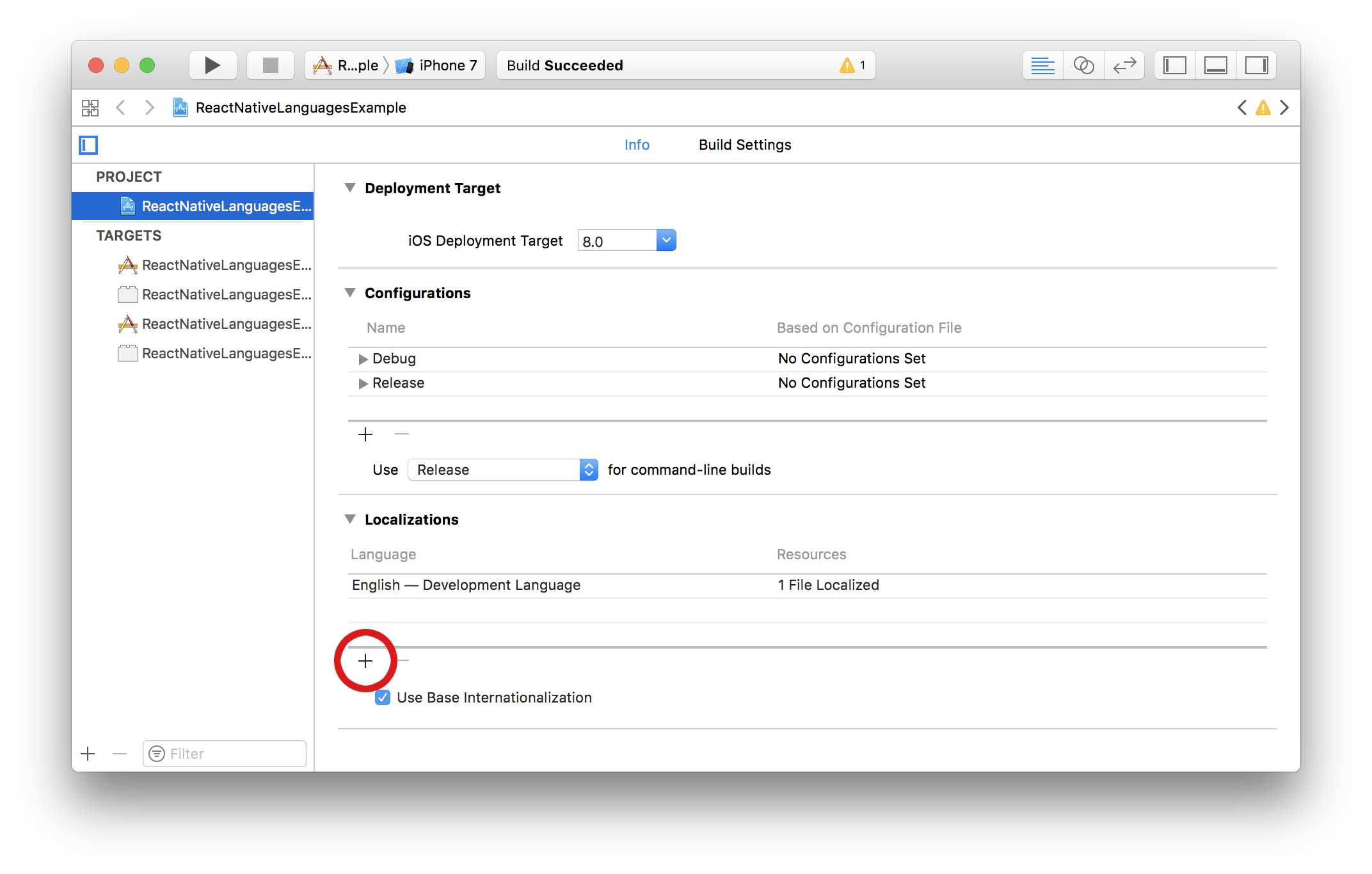
Browse the files in the /example directory.