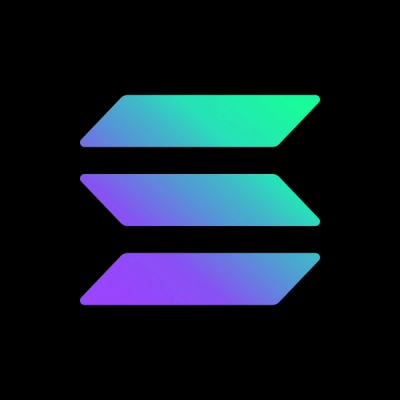
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
react-native-nfc-manager-dibeling
Advanced tools
Bring NFC feature to React Native. Inspired by phonegap-nfc and react-native-ble-manager
Contributions are welcome!
Made with ❤️ by whitedogg13 and revteltech
npm i --save react-native-nfc-manager
This library use native-modules, so you will need to do pod install
for iOS:
cd ios && pod install && cd ..
For Android, it should be properly auto-linked, so you don't need to do anything.
Please see here
To support Android 12, you will need to update compileSdkVersion
in your android/build.gradle
like this:
buildscript {
ext {
...
compileSdkVersion = 31
...
}
...
}
The reason for this is because Android puts new limitation on PendingIntent:
Starting with Build.VERSION_CODES.S, it will be required to explicitly specify the mutability of PendingIntents
The original issue is here
We have a full featured NFC utility app available for download.
It also open sourced in this repo: React Native NFC ReWriter App
If all you want to do is to read NDEF
data, you can use this example:
import NfcManager, {NfcEvents} from 'react-native-nfc-manager';
// Pre-step, call this before any NFC operations
async function initNfc() {
await NfcManager.start();
}
function readNdef() {
const cleanUp = () => {
NfcManager.setEventListener(NfcEvents.DiscoverTag, null);
NfcManager.setEventListener(NfcEvents.SessionClosed, null);
};
return new Promise((resolve) => {
let tagFound = null;
NfcManager.setEventListener(NfcEvents.DiscoverTag, (tag) => {
tagFound = tag;
resolve(tagFound);
NfcManager.setAlertMessageIOS('NDEF tag found');
NfcManager.unregisterTagEvent().catch(() => 0);
});
NfcManager.setEventListener(NfcEvents.SessionClosed, () => {
cleanUp();
if (!tagFound) {
resolve();
}
});
NfcManager.registerTagEvent();
});
}
Anything else, ex: write NDEF, send custom command, please read next section.
In high level, there're 3 steps to perform advanced NFC operations:
NfcManager
object, including:
For example, here's an example to write NDEF:
import NfcManager, {NfcTech, Ndef} from 'react-native-nfc-manager';
// Pre-step, call this before any NFC operations
async function initNfc() {
await NfcManager.start();
}
async function writeNdef({type, value}) {
let result = false;
try {
// Step 1
await NfcManager.requestTechnology(NfcTech.Ndef, {
alertMessage: 'Ready to write some NDEF',
});
const bytes = Ndef.encodeMessage([Ndef.textRecord('Hello NFC')]);
if (bytes) {
await NfcManager.ndefHandler // Step2
.writeNdefMessage(bytes); // Step3
if (Platform.OS === 'ios') {
await NfcManager.setAlertMessageIOS('Successfully write NDEF');
}
}
result = true;
} catch (ex) {
console.warn(ex);
}
// Step 4
NfcManager.cancelTechnologyRequest().catch(() => 0);
return result;
}
Here's an example to read a Mifare Ultralight tag:
import NfcManager, {NfcTech} from 'react-native-nfc-manager';
// Pre-step, call this before any NFC operations
async function initNfc() {
await NfcManager.start();
}
async function readMifare() {
try {
// 0. Request Mifare technology
let reqMifare = await NfcManager.requestTechnology(
NfcTech.MifareUltralight,
);
if (reqMifare !== 'MifareUltralight') {
throw new Error(
'[NFC Read] [ERR] Mifare technology could not be requested',
);
}
// 1. Get NFC Tag information
const nfcTag = await NfcManager.getTag();
console.log('[NFC Read] [INFO] Tag: ', nfcTag);
// 2. Read pages
const readLength = 60;
let mifarePages = [];
const mifarePagesRead = await Promise.all(
[...Array(readLength).keys()].map(async (_, i) => {
const pageOffset = i * 4; // 4 Pages are read at once, so offset should be in steps with length 4
let pages = await NfcManager.mifareUltralightHandlerAndroid.mifareUltralightReadPages(
pageOffset,
);
mifarePages.push(pages);
console.log(`[NFC Read] [INFO] Mifare Page: ${pageOffset}`, pages);
//await wait(500); // If Mifare Chip is to slow
}),
);
// 3. Success
console.log('[NFC Read] [INFO] Success reading Mifare');
// 4. Cleanup
_cleanup();
} catch (ex) {
console.warn('[NFC Read] [ERR] Failed Reading Mifare: ', ex);
_cleanup();
}
}
function _cleanup() {
NfcManager.cancelTechnologyRequest().catch(() => 0);
}
To see more examples, please see React Native NFC ReWriter App
Please see here
Please see here
This package cannot be used in the "Expo Go" app because it requires custom native code.
After installing this npm package, add the config plugin to the plugins
array of your app.json
or app.config.js
:
{
"expo": {
"plugins": ["react-native-nfc-manager"]
}
}
Next, rebuild your app as described in the "Adding custom native code" guide.
The plugin provides props for extra customization. Every time you change the props or plugins, you'll need to rebuild (and prebuild
) the native app. If no extra properties are added, defaults will be used.
nfcPermission
(string | false): Sets the iOS NFCReaderUsageDescription
permission message to the Info.plist
. Setting false
will skip adding the permission. Defaults to Allow $(PRODUCT_NAME) to interact with nearby NFC devices
(Info.plist).selectIdentifiers
(string[]): Sets the iOS com.apple.developer.nfc.readersession.iso7816.select-identifiers
to a list of supported application IDs (Info.plist).systemCodes
(string[]): Sets the iOS com.apple.developer.nfc.readersession.felica.systemcodes
to a user provided list of FeliCa™ system codes that the app supports (Info.plist). Each system code must be a discrete value. The wild card value (0xFF
) isn't allowed.{
"expo": {
"plugins": [
[
"react-native-nfc-manager",
{
"nfcPermission": "Custom permission message",
"selectIdentifiers": ["A0000002471001"],
"systemCodes": ["8008"]
}
]
]
}
}
FAQs
A NFC module for react native.
The npm package react-native-nfc-manager-dibeling receives a total of 1 weekly downloads. As such, react-native-nfc-manager-dibeling popularity was classified as not popular.
We found that react-native-nfc-manager-dibeling demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.