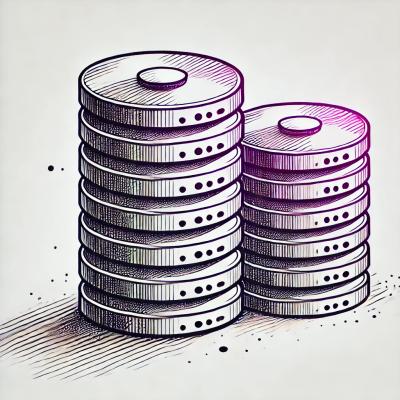
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
react-native-photo-manipulator
Advanced tools
React Native Image Processing API to edit photo programmatically for Android and iOS.
React Native Image Processing API to edit photo programmatically for Android and iOS.
Framework | Android | iOS |
---|---|---|
React Native | ✔️ | ✔️ |
Expo with development build | ✔️ | ✔️ |
Expo Go | ❌ | ❌ |
$ yarn add react-native-photo-manipulator
(or)
$ npm install react-native-photo-manipulator
Please read Get Started Guide
$ yarn expo install react-native-photo-manipulator
(or)
$ npx expo install react-native-photo-manipulator
Import library with
import RNPhotoManipulator from 'react-native-photo-manipulator';
Crop image with cropRegion
and resize to targetSize
if specified
static crop(image: ImageSource, cropRegion: Rect, targetSize?: Size) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The image |
cropRegion | Rect | Yes | The region of image to be cropped |
targetSize | Size | No | The target size of result image |
mimeType | 'image/jpeg', 'image/png' | No | Output file format |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const cropRegion = { x: 5, y: 30, height: 400, width: 250 };
const targetSize = { height: 200, width: 150 };
RNPhotoManipulator.crop(image, cropRegion, targetSize).then(path => {
console.log(`Result image path: ${path}`);
});
Save result image
with specified quality
between 0 - 100
in jpeg format
static optimize(image: ImageSource, quality: number) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The image |
quality | number | Yes | The quality of result image between 0 - 100 |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const quality = 90;
RNPhotoManipulator.optimize(image, 90).then(path => {
console.log(`Result image path: ${path}`);
});
Flip image horizontally, vertically or both
static flipImage(image: ImageSource, mode: FlipMode) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The background image |
mode | FlipMode | Yes | Flip mode Horizontal, Vertical or Both |
mimeType | 'image/jpeg', 'image/png' | No | Output file format |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const mode = FlipMode.Vertical;
RNPhotoManipulator.flipImage(image, mode).then(path => {
console.log(`Result image path: ${path}`);
});
Rotate image 90° (90° Clockwise), 180° (Half Rotation) or 270° (90° Counterclockwise)
static rotateImage(image: ImageSource, mode: RotationMode) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The background image |
mode | RotationMode | Yes | Rotation mode 90° (90° Clockwise), 180° (Half Rotation) or 270° (90° Counterclockwise) |
mimeType | 'image/jpeg', 'image/png' | No | Output file format |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const mode = RotationMode.R90;
RNPhotoManipulator.rotateImage(image, mode).then(path => {
console.log(`Result image path: ${path}`);
});
Overlay image on top of background image
static overlayImage(image: ImageSource, overlay: ImageSource, position: Point) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The background image |
overlay | ImageSource | Yes | The overlay image |
position | Point | Yes | The position of overlay image in background image |
mimeType | 'image/jpeg', 'image/png' | No | Output file format |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const overlay = "https://www.iconfinder.com/icons/1174949/download/png/128";
const position = { x: 5, y: 20 };
RNPhotoManipulator.overlayImage(image, overlay, position).then(path => {
console.log(`Result image path: ${path}`);
});
Print texts into image
static printText(image: ImageSource, texts: TextOptions[]) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The image |
texts | TextOptions[] | Yes | The list of text operations |
mimeType | 'image/jpeg', 'image/png' | No | Output file format |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const texts = [
{ position: { x: 50, y: 30 }, text: "Text 1", textSize: 30, color: "#000000" },
{ position: { x: 50, y: 30 }, text: "Text 1", textSize: 30, color: "#FFFFFF", thickness: 3 }
];
RNPhotoManipulator.printText(image, texts).then(path => {
console.log(`Result image path: ${path}`);
});
Crop, resize and do operations (overlay and printText) on image
static batch(image: ImageSource, operations: PhotoBatchOperations[], cropRegion: Rect, targetSize?: Size, quality?: number) => Promise<string>
Parameter | Type | Required | Description |
---|---|---|---|
image | ImageSource | Yes | The image |
operations | PhotoBatchOperations[] | Yes | The list of operations |
cropRegion | Rect | Yes | The region of image to be cropped |
targetSize | Size | No | The target size of result image |
quality | number | No | The quality of result image between 0 - 100 |
mimeType | 'image/jpeg', 'image/png' | No | Output file format |
Promise with image path in cache directory
const image = "https://unsplash.com/photos/qw6qQQyYQpo/download?force=true";
const cropRegion = { x: 5, y: 30, height: 400, width: 250 };
const targetSize = { height: 200, width: 150 };
const operations = [
{ operation: "text", options: { position: { x: 50, y: 30 }, text: "Text 1", textSize: 30, color: "#000000" } },
{ operation: "overlay", overlay: "https://www.iconfinder.com/icons/1174949/download/png/128", position: { x: 5, y: 20 } },
];
const quality = 90;
RNPhotoManipulator.batch(image, operations, cropRegion, targetSize, quality).then(path => {
console.log(`Result image path: ${path}`);
});
Image resource can be url or require()
Type | Description |
---|---|
number | Image from require('path/to/image') |
string | Image from url supports (file://, http://, https:// and ftp://) or base64 encoded (data:image/png;base64,iVBORw...) |
Represent overlay image, print text or flip operation
Overlay image batch operation
Property | Type | Description |
---|---|---|
operation | "overlay" | |
overlay | ImageSource | The overlay image |
position | Point | he position of overlay image in background image |
Print text batch operation
Property | Type | Description |
---|---|---|
operation | "text" | |
options | TextOptions | The text attributes |
Flip image batch operation
Property | Type | Description |
---|---|---|
operation | "flip" | |
mode | FlipMode | Flip mode Vertical, Horizontal or Both |
Rotate image batch operation
Property | Type | Description |
---|---|---|
operation | "rotate" | |
mode | RotationMode | Rotation mode 90° (90° Clockwise), 180° (Half Rotation) or 270° (90° Counterclockwise) |
Enum represent flip Mode
Enum | Description |
---|---|
Horizontal | Flip horizontal (y-axis) |
Vertical | Flip vertical (x-asis) |
Both | Flip vertical and horizontal |
Enum represent rotation Mode
Enum | Description |
---|---|
R90 | Rotate 90° (90° Clockwise) |
R180 | Rotate 180° (Half Rotation) |
R270 | Rotate 270° (90° Counterclockwise) |
Represent position (x, y) from top-left of image
Property | Type | Description |
---|---|---|
x | number | The x-axis coordinate from top-left |
y | number | The y-axis coordinate from top-left |
Represent area of region
Property | Type | Description |
---|---|---|
x | number | The x-axis coordinate from top-left |
y | number | The y-axis coordinate from top-left |
width | number | The width of the region |
height | number | The height of the region |
Represent size (width, height) of region or image
Property | Type | Description |
---|---|---|
width | number | The width of the region |
height | number | The height of the region |
Enum represent text align
Enum | Description |
---|---|
START | Align text to the start of the line (e.g., left-aligned text in LTR, right-aligned text in RTL) |
CENTER | Align text to the center of the line |
END | Align text to the end of the line (e.g., right-aligned text in LTR, left-aligned text in RTL) |
Enum represent text direction, this will affect coordinate and alignment
Enum | Description |
---|---|
LTR | Left-to-Right text direction (e.g., English, Spanish) [Top-Left, Left] |
RTL | Right-to-Left text direction (e.g., Arabic, Hebrew) [Top-Right, Right] |
Represent attributes of text such as text, color, size, and etc.
Property | Type | Required | Description |
---|---|---|---|
position | Point | Yes | The position of the text in background image |
text | string | Yes | The value of the text |
textSize | number | Yes | The size of the text |
fontName | string | No | The font name that can resolve by React Native iOS: Use "PostScript name" Android: Use filename |
color | string | No | The color of the text |
thickness | number | No | The thickness (border width) of the region |
rotation | number | No | The rotation of text in degrees |
shadowRadius | number | No | The shadow radius |
shadowOffset | Point | No | The shadow offset |
shadowColor | string | No | The color of the shadow |
direction | TextDirection | No | The direction of the text, default to TextDirection.LTR |
align | TextAlign | No | The direction of the text, default to TextAlign.START |
FAQs
React Native Image Processing API to edit photo programmatically for Android and iOS.
The npm package react-native-photo-manipulator receives a total of 5,162 weekly downloads. As such, react-native-photo-manipulator popularity was classified as popular.
We found that react-native-photo-manipulator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.