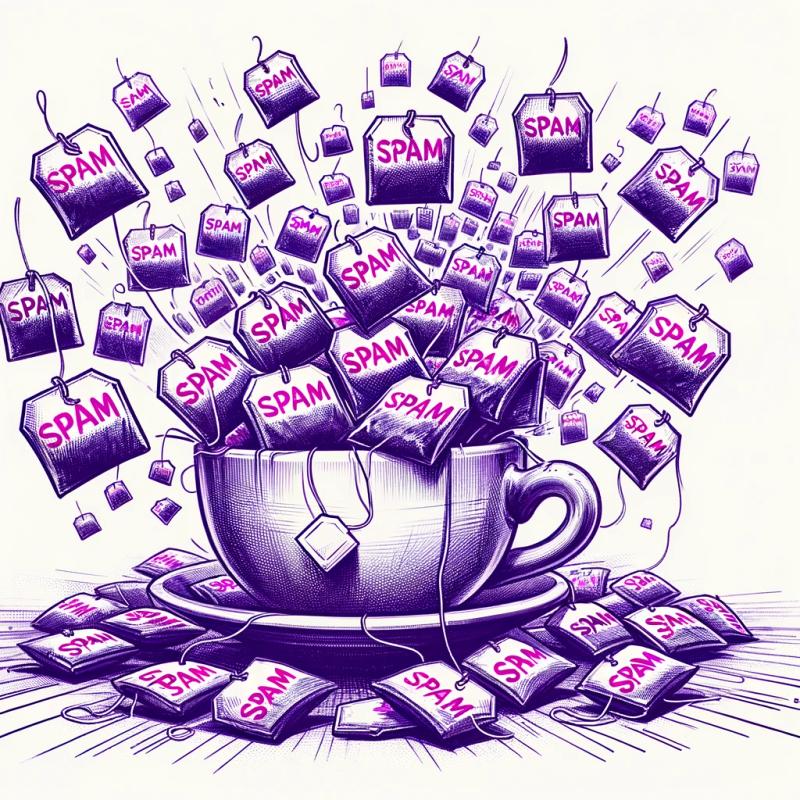
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-native-sqlite-orm
Advanced tools
Readme
It is a simple ORM utility to use with React Native sqlite storage
Warn: it works only on iOS and Android. Web is not supported (SEE)
yarn add react-native-sqlite-orm
You need to provide 3 things:
database
: Instance of expo SQLite or promise with that instancetableName
: The name of the tablecolumnMapping
: The columns for the model and their types
type
, primary_key
, not_null
, unique
, default
import SQLite from 'react-native-sqlite-storage'
import { BaseModel, types } from 'expo-sqlite-orm'
export default class Animal extends BaseModel {
constructor(obj) {
super(obj)
}
static get database() {
return async () => SQLite.openDatabase({ name: 'database.db' })
}
static get tableName() {
return 'animals'
}
static get columnMapping() {
return {
id: { type: types.INTEGER, primary_key: true }, // For while only supports id as primary key
name: { type: types.TEXT, not_null: true },
color: { type: types.TEXT },
age: { type: types.NUMERIC },
another_uid: { type: types.INTEGER, unique: true },
timestamp: { type: types.INTEGER, default: () => Date.now() }
}
}
}
Animal.dropTable()
Create a new table if it doesn't exist
Animal.createTable()
const props = {
name: 'Bob',
color: 'Brown',
age: 2
}
const animal = new Animal(props)
animal.save()
or
const props = {
name: 'Bob',
color: 'Brown',
age: 2
}
Animal.create(new Animal(props))
const id = 1
Animal.find(id).then(animal => animal.name)
or
Animal.findBy({ age_eq: 12345, color_cont: '%Brown%' }).then(animal => animal.name)
const id = 1
const animal = await Animal.find(id)
animal.age = 3
animal.save()
or
const props = {
id: 1 // required
age: 3
}
Animal.update(props)
const id = 1
Animal.destroy(id)
or
const id = 1
const animal = await Animal.find(id)
animal.destroy()
Animal.destroyAll()
const options = {
columns: 'id, name',
where: {
age_gt: 2
},
page: 2,
limit: 30,
order: 'name ASC'
}
Animal.query(options)
Where operations
=
,<>
,<
,<=
,>
,>=
,LIKE
import { BaseModel } from 'expo-sqlite-orm'
export default class Example extends BaseModel {
//...another model methods...
static myCustomMethod() {
const sql = 'SELECT * FROM table_name WHERE status = ?'
const params = ['active']
return this.repository.databaseLayer.executeSql(sql, params).then(({ rows }) => rows)
}
}
or
import SQLite from 'react-native-sqlite-storage'
import DatabaseLayer from 'expo-sqlite-orm/src/DatabaseLayer'
const databaseLayer = new DatabaseLayer(async () => SQLite.openDatabase({ name: 'database_name' }))
databaseLayer.executeSql('SELECT * from table_name;').then(response => {
console.log(response)
})
import SQLite from 'react-native-sqlite-storage'
import DatabaseLayer from 'expo-sqlite-orm/src/DatabaseLayer'
const databaseLayer = new DatabaseLayer(async () => SQLite.openDatabase('database_name'), 'table_name')
const itens = [{id: 1, color: 'green'}, {id: 2, color: 'red'}]
databaseLayer.bulkInsertOrReplace(itens).then(response => {
console.log(response)
})
docker-compose run --rm bump # patch
docker-compose run --rm bump --minor # minor
git push
git push --tags
docker-compose run --rm app install
docker-compose run --rm app test
TBA
Inspired by
Upgraded by
This project is licensed under MIT License
FAQs
Rails-style sqlite ORM for React Native
The npm package react-native-sqlite-orm receives a total of 6 weekly downloads. As such, react-native-sqlite-orm popularity was classified as not popular.
We found that react-native-sqlite-orm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.