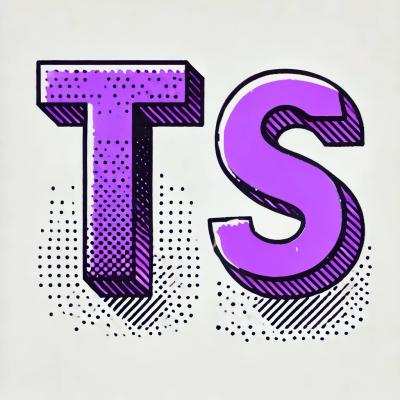
Security News
Node.js Moves Toward Stable TypeScript Support with Amaro 1.0
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
react-native-tcp-socket
Advanced tools
React Native TCP socket API for Android & iOS with SSL/TLS support
React Native TCP socket API for Android, iOS & macOS with SSL/TLS support. It allows you to create TCP client and server sockets, imitating Node's net and Node's tls API functionalities (check the available API for more information).
Install the library using either Yarn:
yarn add react-native-tcp-socket
or npm:
npm install --save react-native-tcp-socket
net
Since react-native-tcp-socket
offers the same API as Node's net, in case you want to import this module as net
or use require('net')
in your JavaScript, you must add the following lines to your package.json
file.
{
"react-native": {
"net": "react-native-tcp-socket"
}
}
In addition, in order to obtain the TS types (or autocompletion) provided by this module, you must also add the following to your custom declarations file.
...
declare module 'net' {
import TcpSockets from 'react-native-tcp-socket';
export = TcpSockets;
}
If you want to avoid duplicated net
types, make sure not to use the default node_modules/@types
in your tsconfig.json
"typeRoots"
property.
Check the example app provided for a working example.
tls
The same applies to tls
module. However, you should be aware of the following:
Server
class exported by default is non-TLS. In order to use the TLS server, you must use the TLSServer
class. You may override the default Server
class (tls.Server = tls.TLSServer
). The same goes with the createServer()
and connect()
. In order to use the TLS methods, you must use the createTLSServer()
and connectTLS()
methods respectively. You may override the default methods (tls.createServer = tls.createTLSServer
and tls.connect = tls.connectTLS
).tls
module requires the keys and certificates to be provided as a string. However, the react-native-tcp-socket
module requires them to be imported with require()
.In addition, in order to obtain the TS types (or autocompletion) provided by this module, you must also add the following to your custom declarations file.
...
declare module 'tls' {
import TcpSockets from 'react-native-tcp-socket';
export const Server = TcpSockets.TLSServer;
export const TLSSocket = TcpSockets.TLSSocket;
export const connect = TcpSockets.connectTLS;
export const createServer = TcpSockets.createTLSServer;
}
Check the example app provided for a working example.
Linking the package manually is not required anymore with Autolinking.
iOS Platform:
$ cd ios && pod install && cd ..
# CocoaPods on iOS needs this extra step
Android Platform:
Modify your android/build.gradle
configuration to match minSdkVersion = 21
:
buildscript {
ext {
...
minSdkVersion = 21
...
}
In order to generate the required files (keys and certificates) for self-signed SSL, you can use the following command:
openssl genrsa -out server-key.pem 4096
openssl req -new -key server-key.pem -out server-csr.pem
openssl x509 -req -in server-csr.pem -signkey server-key.pem -out server-cert.pem
openssl pkcs12 -export -out server-keystore.p12 -inkey server-key.pem -in server-cert.pem
Note: The server-keystore.p12
must not have a password.
You will need a metro.config.js file in order to use a self-signed SSL certificate. You should already have this file in your root project directory, but if you don't, create it.
Inside a module.exports
object, create a key called resolver
with another object called assetExts
. The value of assetExts
should be an array of the resource file extensions you want to support.
If you want to be able to use .pem
and .p12
files (plus all the already supported files), your metro.config.js
should look like this:
const {getDefaultConfig} = require('metro-config');
const defaultConfig = getDefaultConfig.getDefaultValues(__dirname);
module.exports = {
resolver: {
assetExts: [...defaultConfig.resolver.assetExts, 'pem', 'p12'],
},
// ...
};
You then need to link the native parts of the library for the platforms you are using. The easiest way to link the library is using the CLI tool by running this command from the root of your project:
$ react-native link react-native-tcp-socket
If you can't or don't want to use the CLI tool, you can also manually link the library using the instructions below (click on the arrow to show them):
Libraries
➜ Add Files to [your project's name]
node_modules
➜ react-native-tcp-socket
and add TcpSockets.xcodeproj
libTcpSockets.a
to your project's Build Phases
➜ Link Binary With Libraries
Cmd+R
)<android/app/src/main/java/[...]/MainApplication.java
import com.asterinet.react.tcpsocket.TcpSocketPackage;
to the imports at the top of the filenew TcpSocketPackage()
to the list returned by the getPackages()
methodandroid/settings.gradle
:
include ':react-native-tcp-socket'
project(':react-native-tcp-socket').projectDir = new File(rootProject.projectDir, '../node_modules/react-native-tcp-socket/android')
android/app/build.gradle
:
implementation project(':react-native-tcp-socket')
To use this library you need to ensure you are using the correct version of React Native. If you are using a version of React Native that is lower than 0.60
you will need to upgrade before attempting to use the latest version.
react-native-tcp-socket version | Required React Native Version |
---|---|
6.X.X , 5.X.X , 4.X.X , 3.X.X | >= 0.60.0 |
1.4.0 | >= Unknown |
Import the library:
import TcpSocket from 'react-native-tcp-socket';
// const net = require('react-native-tcp-socket');
// const tls = require('react-native-tcp-socket');
const options = {
port: port,
host: '127.0.0.1',
localAddress: '127.0.0.1',
reuseAddress: true,
// localPort: 20000,
// interface: "wifi",
};
// Create socket
const client = TcpSocket.createConnection(options, () => {
// Write on the socket
client.write('Hello server!');
// Close socket
client.destroy();
});
client.on('data', function(data) {
console.log('message was received', data);
});
client.on('error', function(error) {
console.log(error);
});
client.on('close', function(){
console.log('Connection closed!');
});
const server = TcpSocket.createServer(function(socket) {
socket.on('data', (data) => {
socket.write('Echo server ' + data);
});
socket.on('error', (error) => {
console.log('An error ocurred with client socket ', error);
});
socket.on('close', (error) => {
console.log('Closed connection with ', socket.address());
});
}).listen({ port: 12345, host: '0.0.0.0' });
server.on('error', (error) => {
console.log('An error ocurred with the server', error);
});
server.on('close', () => {
console.log('Server closed connection');
});
const options = {
port: port,
host: '127.0.0.1',
localAddress: '127.0.0.1',
reuseAddress: true,
// localPort: 20000,
// interface: "wifi",
ca: require('server-cert.pem'),
};
// Create socket
const client = TcpSocket.connectTLS(options, () => {
// Write on the socket
client.write('Hello server!');
// Close socket
client.destroy();
});
client.on('data', function(data) {
console.log('message was received', data);
});
client.on('error', function(error) {
console.log(error);
});
client.on('close', function(){
console.log('Connection closed!');
});
const options = {
keystore: require('server-keystore.p12'),
};
const server = TcpSocket.createTLSServer(options, function(socket) {
socket.on('data', (data) => {
socket.write('Echo server ' + data);
});
socket.on('error', (error) => {
console.log('An error ocurred with SSL client socket ', error);
});
socket.on('close', (error) => {
console.log('SSL closed connection with ', socket.address());
});
}).listen({ port: 12345, host: '0.0.0.0' });
server.on('error', (error) => {
console.log('An error ocurred with the server', error);
});
server.on('close', () => {
console.log('Server closed connection');
});
Note: In order to use self-signed certificates make sure to update your metro.config.js configuration.
Here are listed all methods implemented in react-native-tcp-socket
that imitate Node's net API, their functionalities are equivalent to those provided by Node's net (more info on #41). However, the methods whose interface differs from Node are marked in bold.
net.connect(options[, callback])
net.createConnection(options[, callback])
net.createServer([options][, connectionListener])
net.isIP(input)
net.isIPv4(input)
net.isIPv6(input)
address()
destroy([error])
end([data][, encoding][, callback])
setEncoding([encoding])
setKeepAlive([enable][, initialDelay])
- initialDelay
is ignoredsetNoDelay([noDelay])
setTimeout(timeout[, callback])
write(data[, encoding][, callback])
pause()
ref()
- Will not have any effectresume()
unref()
- Will not have any effectnet.createConnection()
net.createConnection(options[, callback])
creates a TCP connection using the given options
. The options
parameter must be an object
with the following properties:
Property | Type | iOS/macOS | Android | Description |
---|---|---|---|---|
port | <number> | ✅ | ✅ | Required. Port the socket should connect to. |
host | <string> | ✅ | ✅ | Host the socket should connect to. IP address in IPv4 format or 'localhost' . Default: 'localhost' . |
localAddress | <string> | ✅ | ✅ | Local address the socket should connect from. If not specified, the OS will decide. It is highly recommended to specify a localAddress to prevent overload errors and improve performance. |
localPort | <number> | ✅ | ✅ | Local port the socket should connect from. If not specified, the OS will decide. |
interface | <string> | ❌ | ✅ | Interface the socket should connect from. If not specified, it will use the current active connection. The options are: 'wifi', 'ethernet', 'cellular' . |
reuseAddress | <boolean> | ❌ | ✅ | Enable/disable the reuseAddress socket option. Default: true . |
Note: The platforms marked as ❌ use the default value.
Server.listen()
Server.listen(options[, callback])
creates a TCP server socket using the given options
. The options
parameter must be an object
with the following properties:
Property | Type | iOS/macOS | Android | Description |
---|---|---|---|---|
port | <number> | ✅ | ✅ | Required. Port the socket should listen to. |
host | <string> | ✅ | ✅ | Host the socket should listen to. IP address in IPv4 format or 'localhost' . Default: '0.0.0.0' . |
reuseAddress | <boolean> | ❌ | ✅ | Enable/disable the reuseAddress socket option. Default: true . |
Note: The platforms marked as ❌ use the default value.
Here are listed all methods implemented in react-native-tcp-socket
that imitate Node's tls API, their functionalities are equivalent to those provided by Node's tls. However, the methods whose interface differs from Node are marked in bold.
Socket
getCertificate()
getPeerCertificate()
Socket
Socket
'secureConnect'
tls.connectTLS()
tls.connectTLS(options[, callback])
creates a TLS socket connection using the given options
. The options
parameter must be an object
with the following properties:
Property | Type | iOS/macOS | Android | Description |
---|---|---|---|---|
ca | <import> | ✅ | ✅ | CA file (.pem format) to trust. If null , it will use the device's default SSL trusted list. Useful for self-signed certificates. Check the documentation for generating such file. Default: null . |
key | <import> | <string> | ✅ | ✅ | Private key file (.pem format). Check the documentation for generating such file. |
cert | <import> | <string> | ✅ | ✅ | Public certificate file (.pem format). Check the documentation for generating such file. |
androidKeyStore | <string> | ❌ | ✅ | Android KeyStore alias. |
certAlias | <string> | ✅ | ✅ | Android KeyStore certificate alias. |
keyAlias | <string> | ✅ | ✅ | Android KeyStore private key alias. |
... | <any> | ✅ | ✅ | Any other socket.connect() options not already listed. |
Note: The TLS server is named Server
in Node's tls, but it is named TLSServer
in react-native-tcp-socket
in order to avoid confusion with the Server
class.
Server
setSecureContext(options)
Server
Server
'secureConnection'
tls.createTLSServer()
tls.createTLSServer([options][, secureConnectionListener])
creates a new tls.TLSServer
. The secureConnectionListener
, if provided, is automatically set as a listener for the 'secureConnection'
event. The options
parameter must be an object
with the following properties:
Property | Type | iOS/macOS | Android | Description |
---|---|---|---|---|
keystore | <import> | ✅ | ✅ | Required. Key store in PKCS#12 format with the server certificate and private key. Check the documentation for generating such file. |
The library is released under the MIT license. For more information see LICENSE
.
FAQs
React Native TCP socket API for Android & iOS with SSL/TLS support
The npm package react-native-tcp-socket receives a total of 15,232 weekly downloads. As such, react-native-tcp-socket popularity was classified as popular.
We found that react-native-tcp-socket demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.