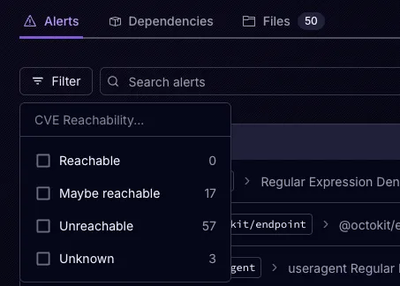
Product
Announcing Precomputed Reachability Analysis in Socket
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
react-next-carousel
Advanced tools
Typescript Supported Powerful, lightweight and fully customizable carousel component for React.js and Next.js apps.
I don't have any time available to keep maintaining this package. If you have any request, try to sort it within the community. I'm able to merge pull requests that look safe from time to time but no commitment on timelines here. Feel free to fork it and publish under other name if you are in a hurry or to use another component.
http://leandrowd.github.io/react-responsive-carousel/
Check it out these cool demos created using storybook.
yarn add react-next-carousel
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import "react-next-carousel/lib/styles/carousel.min.css"; // requires a loader
import { Carousel } from 'react-next-carousel';
class DemoCarousel extends Component {
const slides = [
"https://fastly.picsum.photos/id/11/2500/1667.jpg?hmac=xxjFJtAPgshYkysU_aqx2sZir-kIOjNR9vx0te7GycQ",
"https://fastly.picsum.photos/id/13/2500/1667.jpg?hmac=SoX9UoHhN8HyklRA4A3vcCWJMVtiBXUg0W4ljWTor7s",
"https://fastly.picsum.photos/id/12/2500/1667.jpg?hmac=Pe3284luVre9ZqNzv1jMFpLihFI6lwq7TPgMSsNXw2w",
"https://fastly.picsum.photos/id/27/3264/1836.jpg?hmac=p3BVIgKKQpHhfGRRCbsi2MCAzw8mWBCayBsKxxtWO8g",
"https://fastly.picsum.photos/id/28/4928/3264.jpg?hmac=GnYF-RnBUg44PFfU5pcw_Qs0ReOyStdnZ8MtQWJqTfA",
"https://fastly.picsum.photos/id/29/4000/2670.jpg?hmac=rCbRAl24FzrSzwlR5tL-Aqzyu5tX_PA95VJtnUXegGU",
]
render() {
return (
<Carousel slides={slides}/>
);
}
});
ReactDOM.render(<DemoCarousel />, document.querySelector('.demo-carousel'));
// Don't forget to include the css in your page
// Using webpack or parcel with a style loader
// import styles from 'react-responsive-carousel/lib/styles/carousel.min.css';
// Using html tag:
// <link rel="stylesheet" href="<NODE_MODULES_FOLDER>/react-responsive-carousel/lib/styles/carousel.min.css"/>
Name | Value | Description |
---|---|---|
autoPlay | boolean | Change the slide automatically based on interval prop. |
interval | number | Interval in milliseconds to automatically go to the next item when autoPlay is true, defaults to 2000 . |
showCarouselArrow | boolean | Enable previous and next arrow, defaults to true . |
showIndicators | boolean | Enable indicators to select items, defaults to true . |
showThumbs | boolean | Enable thumbs, defaults to true . |
thumbWidth | number | Width of the thumb, defaults to 80 . |
width | number or string | The width of the carousel, defaults to 100% . |
FAQs
react.js and next.js carousel with typescript
The npm package react-next-carousel receives a total of 0 weekly downloads. As such, react-next-carousel popularity was classified as not popular.
We found that react-next-carousel demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.