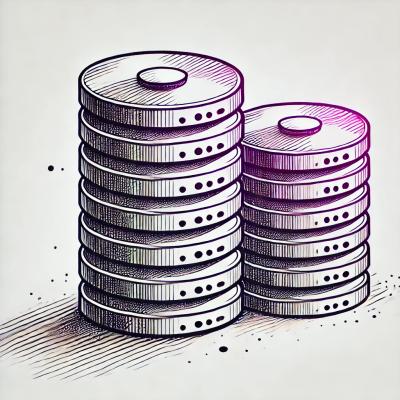
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
react-querybuilder
Advanced tools
React Query Builder component for constructing queries and filters, with utilities for executing them in various database and evaluation contexts
The Query Builder component for React
React Query Builder is a fully customizable query builder component for React, along with a collection of utility functions for importing from, and exporting to, various query languages like SQL, MongoDB, and more.
npm i react-querybuilder
# OR yarn add / pnpm add / bun add
import { useState } from 'react';
import { Field, QueryBuilder, RuleGroupType } from 'react-querybuilder';
import 'react-querybuilder/dist/query-builder.css';
const fields: Field[] = [
{ name: 'firstName', label: 'First Name' },
{ name: 'lastName', label: 'Last Name' },
{ name: 'age', label: 'Age', inputType: 'number' },
{ name: 'address', label: 'Address' },
{ name: 'phone', label: 'Phone' },
{ name: 'email', label: 'Email', validator: ({ value }) => /^[^@]+@[^@]+/.test(value) },
{ name: 'twitter', label: 'Twitter' },
{ name: 'isDev', label: 'Is a Developer?', valueEditorType: 'checkbox', defaultValue: false },
];
const initialQuery: RuleGroupType = {
combinator: 'and',
rules: [],
};
export const App = () => {
const [query, setQuery] = useState(initialQuery);
return <QueryBuilder fields={fields} defaultQuery={query} onQueryChange={setQuery} />;
};
Customizations are not limited to the following libraries, but these have first-class support through their respective compatibility packages:
[!TIP]
To enable drag-and-drop, use
@react-querybuilder/dnd
.For enhanced date/time support, use
@react-querybuilder/datetime
.
To export queries as a SQL WHERE
clause, MongoDB query object, or one of several other formats, use formatQuery
.
const query = {
combinator: 'and',
rules: [
{
field: 'first_name',
operator: 'beginsWith',
value: 'Stev',
},
{
field: 'last_name',
operator: 'in',
value: 'Vai, Vaughan',
},
],
};
formatQuery(query, 'sql');
/*
"(first_name like 'Stev%' and last_name in ('Vai', 'Vaughan'))"
*/
To import queries use parseSQL
, parseMongoDB
, parseJsonLogic
, parseJSONata
, parseCEL
, or parseSpEL
depending on the source.
// Tip: `parseSQL` will accept either a full `SELECT` statement or a `WHERE` clause by itself.
// Everything but the `WHERE` expressions will be ignored.
const query = parseSQL(
`SELECT * FROM my_table WHERE first_name LIKE 'Stev%' AND last_name in ('Vai', 'Vaughan')`
);
console.log(query);
/*
{
"combinator": "and",
"rules": [
{
"field": "first_name",
"operator": "beginsWith",
"value": "Stev",
},
{
"field": "last_name",
"operator": "in",
"value": "Vai, Vaughan",
},
],
}
*/
formatQuery
, transformQuery
, and the parse*
functions can be used without importing from react
(on the server, for example) like this:
import { formatQuery } from 'react-querybuilder/formatQuery';
import { parseCEL } from 'react-querybuilder/parseCEL';
import { parseJSONata } from 'react-querybuilder/parseJSONata';
import { parseJsonLogic } from 'react-querybuilder/parseJsonLogic';
import { parseMongoDB } from 'react-querybuilder/parseMongoDB';
import { parseSpEL } from 'react-querybuilder/parseSpEL';
import { parseSQL } from 'react-querybuilder/parseSQL';
import { transformQuery } from 'react-querybuilder/transformQuery';
(As of version 7, the parse*
functions are only available through these extended exports.)
FAQs
React Query Builder component for constructing queries and filters, with utilities for executing them in various database and evaluation contexts
The npm package react-querybuilder receives a total of 0 weekly downloads. As such, react-querybuilder popularity was classified as not popular.
We found that react-querybuilder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.