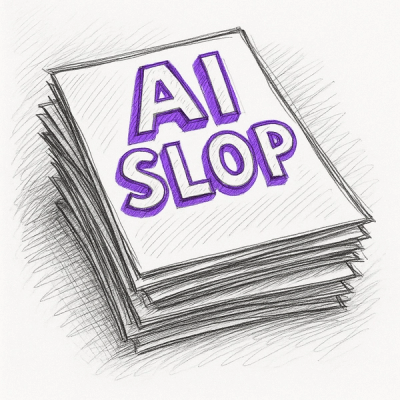
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
react-quill
Advanced tools
react-quill is a React component for Quill, a powerful rich text editor. It provides a customizable and extensible interface for creating and editing content with a variety of formatting options.
Basic Usage
This code demonstrates the basic usage of react-quill. It imports the necessary modules, sets up a state to hold the editor's content, and renders the ReactQuill component.
import React, { useState } from 'react';
import ReactQuill from 'react-quill';
import 'react-quill/dist/quill.snow.css';
function MyEditor() {
const [value, setValue] = useState('');
return (
<ReactQuill value={value} onChange={setValue} />
);
}
export default MyEditor;
Custom Toolbar
This code demonstrates how to create a custom toolbar for the react-quill editor. It defines a CustomToolbar component and uses it in the MyEditor component, specifying the custom toolbar in the modules prop.
import React, { useState } from 'react';
import ReactQuill, { Quill } from 'react-quill';
import 'react-quill/dist/quill.snow.css';
const CustomToolbar = () => (
<div id="toolbar">
<button className="ql-bold">Bold</button>
<button className="ql-italic">Italic</button>
<select className="ql-color">
<option value="red"></option>
<option value="green"></option>
<option value="blue"></option>
</select>
</div>
);
function MyEditor() {
const [value, setValue] = useState('');
return (
<div>
<CustomToolbar />
<ReactQuill value={value} onChange={setValue} modules={{ toolbar: '#toolbar' }} />
</div>
);
}
export default MyEditor;
Formats and Modules
This code demonstrates how to specify custom formats and modules for the react-quill editor. It defines the formats and modules arrays and passes them as props to the ReactQuill component.
import React, { useState } from 'react';
import ReactQuill from 'react-quill';
import 'react-quill/dist/quill.snow.css';
const formats = [
'header', 'bold', 'italic', 'underline', 'strike', 'blockquote',
'list', 'bullet', 'indent',
'link', 'image'
];
const modules = {
toolbar: [
[{ 'header': '1' }, { 'header': '2' }, { 'font': [] }],
[{ 'list': 'ordered'}, { 'list': 'bullet' }],
['bold', 'italic', 'underline', 'strike', 'blockquote'],
['link', 'image'],
[{ 'align': [] }]
]
};
function MyEditor() {
const [value, setValue] = useState('');
return (
<ReactQuill value={value} onChange={setValue} modules={modules} formats={formats} />
);
}
export default MyEditor;
draft-js is a framework for building rich text editors in React, developed by Facebook. It provides a more programmatic approach to managing editor state and content, allowing for extensive customization and control over the editor's behavior. Compared to react-quill, draft-js requires more setup and configuration but offers greater flexibility.
slate is another highly customizable framework for building rich text editors in React. It provides a more modern and flexible approach to handling editor state and content, with a focus on immutability and functional programming. Slate is more complex to set up compared to react-quill but offers extensive customization options and control over the editor's behavior.
ckeditor4-react is a React integration for CKEditor 4, a popular WYSIWYG editor. It offers a wide range of features and plugins for content editing, similar to react-quill. CKEditor 4 provides a more traditional WYSIWYG editing experience and is highly configurable, making it a good alternative for users looking for a feature-rich editor.
v0.0.4
QuillToolbar
QuillComponent
only accepting a single child.FAQs
The Quill rich-text editor as a React component.
The npm package react-quill receives a total of 535,991 weekly downloads. As such, react-quill popularity was classified as popular.
We found that react-quill demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.