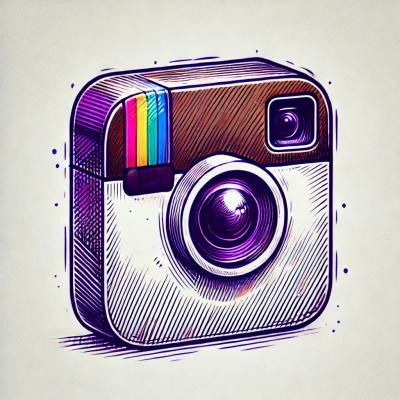
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
react-ranger
Advanced tools
Hooks for building range and multi-range sliders in React
Enjoy this library? Try them all! React Table, React Query, React Form, React Charts
This library is being built and maintained by me, @tannerlinsley and I am always in need of more support to keep projects like this afloat. If you would like to get premium support, add your logo or name on this README, or simply just contribute to my open source Sponsorship goal, visit my Github Sponsors page!
Get Your Logo Here! |
Get Your Logo Here! |
Get Your Logo Here! |
![]() |
$ npm i --save react-ranger
# or
$ yarn add react-ranger
The following is a very basic example of a single range input that looks similar to Chrome's default appearance.
import ReactRanger from 'react-ranger'
function App() {
const [values, setValues] = React.useState([10])
const { getTrackProps, handles } = useRanger({
values,
onChange: setValues,
min: 0,
max: 100,
stepSize: 5,
})
return (
<>
<div
{...getTrackProps({
style: {
height: '4px',
background: '#ddd',
boxShadow: 'inset 0 1px 2px rgba(0,0,0,.6)',
borderRadius: '2px',
},
})}
>
{handles.map(({ getHandleProps }) => (
<div
{...getHandleProps({
style: {
width: '12px',
height: '12px',
borderRadius: '100%',
background: 'linear-gradient(to bottom, #eee 45%, #ddd 55%)',
border: 'solid 1px #888',
},
})}
/>
))}
</div>
</>
)
}
value: Array<number>
- The current value (or values) for the range
min: number
- The minimum limit for the range
max: number
- The maximum limit for the range
stepSize: number
- The distance between selectable steps
steps: arrayOf(number)
- An array of custom steps to use. This will override stepSize
,tickSize: number
ticks: arrayOf(number): Default: 10
- An array of custom ticks to use. This will override tickSize
,onChange: Function(newValue)
- A function that is called when the handle is releasedonDrag: Function(newValue)
- A function that is called when a handled is draggedinterpolator: { getPercentageForValue: Function(value) => decimal, getValueForClientX: Function(x) => value}
useRanger
returns an object
with the following properties:
getTrackProps(userProps): func
- A function that takes optional props and returns the combined necessary props for the track component.ticks: array
- Ticks to be rendered. Each tick
has the following props:
value: number
- The tick number to be displayedgetTickProps(userProps): func
- A function that take optional props and returns the combined necessary props for the tick component.segments: array
- Segments to be rendered. Each segment
has the following props:
value: number
- The segments ending valuegetSegmentProps(userProps): func
- A function that take optional props and returns the combined necessary props for the segment component.handles: array
- Handles to be rendered. Each handle
has the following props:
value: number
- The current value for the handleactive: boolean
- Denotes if the handle is currently being dragged.getHandleProps(userProps): func
- A function that take optional props and returns the combined necessary props for the handle component.activeHandleIndex: oneOfType([null, number])
- The zero-based index of the handle that is currently being dragged, or null
if no handle is being dragged.By default, react-ranger
uses linear interpolation between data points, but allows you to easily customize it to use your own interpolation functions by passing an object that implements the following interface:
const interpolator = {
// Takes the value & range and returns a percentage [0, 100] where the value sits from left to right
getPercentageForValue: (val: number, min: number, max: number): number
// Takes the clientX (offset from the left edge of the ranger) along with the dimensions
// and range settings and transforms a pixel coordinate back into a value
getValueForClientX: (clientX: number, trackDims: object, min: number, max: number): number
}
Here is an exmaple of building and using a logarithmic interpolator!
import { useRanger } from 'react-ranger'
const logInterpolator = {
getPercentageForValue: (val, min, max) => {
const minSign = Math.sign(min)
const maxSign = Math.sign(max)
if (minSign !== maxSign) {
throw new Error(
'Error: logarithmic interpolation does not support ranges that cross 0.'
)
}
let percent =
(100 / (Math.log10(Math.abs(max)) - Math.log10(Math.abs(min)))) *
(Math.log10(Math.abs(val)) - Math.log10(Math.abs(min)))
if (minSign < 0) {
// negative range, means we need to invert our percent because of the Math.abs above
return 100 - percent
}
return percent
},
getValueForClientX: (clientX, trackDims, min, max) => {
const { left, width } = trackDims
let value = clientX - left
value *= Math.log10(max) - Math.log10(min)
value /= width
value = Math.pow(10, Math.log10(min) + value)
return value
},
}
useRanger({
interpolator: logInterpolator,
})
Thanks goes to these wonderful people (emoji key):
Tanner Linsley 💻 🤔 💡 🚧 👀 | Evert Timberg 💻 🤔 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Hooks for building range and multi-range sliders in React
The npm package react-ranger receives a total of 6,702 weekly downloads. As such, react-ranger popularity was classified as popular.
We found that react-ranger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.