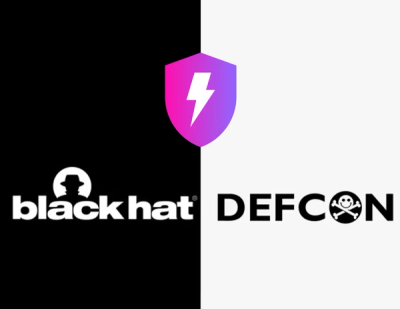
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
react-redux-local-form
Advanced tools
Manage component state with a local redux store. Includes react components for building composable forms.
React Redux Local Form is a set of minimal React components to help with building forms. State is managed with a Redux store that is local to your component. This promotes keeping your ui state separate from your global state while still being able to leverage the redux ecosystem. You can also mix and match redux reducers/actions between local and global state. If these ideas appeal to you read on... if not, check out some of these great alternatives:
React 15.3.0 and Redux 3.0.0 or later are peer dependencies.
npm install --save react-redux-local-form
import React from 'react'
import { withForm, FormProvider, Field } from 'react-redux-local-form'
function BasicForm({ form, onSubmit }) {
return (
<FormProvider store={form} onSubmit={onSubmit}>
<form onSubmit={preventDefault(form.submit)}>
<Field path="user.firstName">
{ ({ value = '', setValue }) =>
<div>
<label>First Name</label>
<input type="text" value={value} onChange={(e) => setValue(e.target.value)} />
</div>
}
</Field>
<button type="submit">Save</button>
</form>
</FormProvider>
)
}
const preventDefault = (next) => (e) => {
e.preventDefault()
next()
}
export default withForm()(BasicForm)
Check out the basic form example for the entire source.
Setting initial form state is done by passing it into withForm
...
export default withForm({
user: {
firstName: 'john'
}
})(BasicForm)
// or a function of props
export default withForm((props) => {
user: {
firstName: props.user.firstName
}
})(BasicForm)
This lib currently doesn't provide any validation functions out of the box, only an API to provide your own. Validation functions are simply functions that accept the current value and return a promise. Pass in a single validation function or an array to the <Field>
component. The form won't submit until all validation functions are resolved.
import React from 'react'
import { withForm, FormProvider, Field } from 'react-redux-local-form'
import { isEmail } from 'validator'
const required = (value) => new Promise((resolve, reject) => {
if (value) { resolve() }
else { reject(new Error('Invalid Email')) }
})
const email = (value) => new Promise((resolve, reject) => {
if (isEmail(value)) { resolve() }
else { reject(new Error('Invalid Email')) }
})
function BasicForm({ form, onSubmit }) {
return (
<FormProvider store={form} onSubmit={onSubmit}>
<form onSubmit={preventDefault(form.submit)}>
<Field path="user.firstName" validate={required}>
{ ({ value = '', setValue, error }) =>
<div>
<label>First Name</label>
<input type="text" value={value} onChange={(e) => setValue(e.target.value)} />
{ error && error.message }
</div>
}
</Field>
<Field path="user.firstName" validate={[ required, email ]}>
...
</Field>
<button type="submit">Save</button>
</form>
</FormProvider>
)
}
export default withForm()(BasicForm)
Check out the basic form example for the entire source.
Use the connectForm
function to map form state to props. This function has the exact same API as react-redux's connect
function.
import React from 'react'
import { withForm, connectForm, FormProvider, Field } from 'react-redux-local-form'
function mapFormStateToProps(formState) {
return {
userFormState: formState.user,
allErrors: formState.errors
}
}
function BasicForm({ userFormState, allErrors, form, onSubmit }) {
...
})
export default withForm()(
connectForm(mapFormStateToProps)(withForm)
)
FAQs
React components for building forms with a local Redux store for managing state.
The npm package react-redux-local-form receives a total of 1 weekly downloads. As such, react-redux-local-form popularity was classified as not popular.
We found that react-redux-local-form demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.