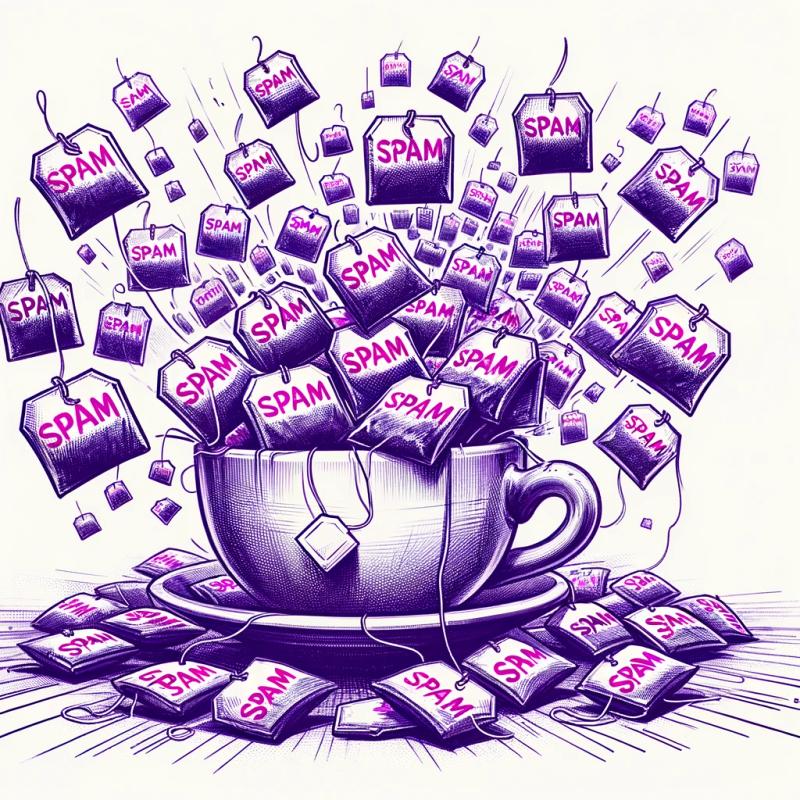
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
react-reorder-list
Advanced tools
Readme
A simple react component that facilitates the reordering of JSX/HTML elements through drag-and-drop functionality, allowing for easy position changes.
To install react-reorder-list
# with npm:
npm install react-reorder-list --save
# with yarn:
yarn add react-reorder-list
# with pnpm:
pnpm add react-reorder-list
# with bun:
bun add react-reorder-list
react-reorder-list
default exports <ReorderList>
component which encapsulates all the list items as its children.
Items in this list can be reordered by simply dragging an item and dropping it in place of another item.
Each <div>
component inside <ReorderList>
can now be drag-and-dropped to another <div>
to reorder them.
import React from "react";
import ReorderList from "react-reorder-list";
export default function App() {
return (
<ReorderList>
{[0, 1, 2, 3, 4].map((i) => {
return <div key={i}>Item {i}</div>; // Having a unique key is important
})}
</ReorderList>
);
}
The dragging behavior can be changed using the useOnlyIconToDrag
prop of <ReorderList>
component.
If set to false
, an item can be dragged by clicking anywhere inside the item.
If set to true
, an item can be dragged only using the <ReorderIcon>
present inside the item.
import React from 'react'
import ReorderList, { ReorderIcon } from 'react-reorder-list'
export default function App() {
return <ReorderList useOnlyIconToDrag={true}>
{[0, 1, 2, 3, 4].map(i => {
return <div key={i}>
<ReorderIcon /> {/* Default icon */}
<ReorderIcon>
{/* Custom icon/component */}
</Reordericon>
<span>{i}</span>
</div>
})}
</ReorderList>
}
<ReorderList>
can listen to updates to it's children components using the watchChildrenUpdates
prop as shown below.
If set to false
, any updates made in children component except reordering by user won't reflect.
If set to true
, updates to children like state changes, additions/omissions of children components will reflect in real time.
Further if preserveOrder
is set to false, the order in which new children appear will be maintained.
Whereas if preserveOrder
is set to true, the order of existing items will be preserved as before the update occured and new items will be placed at the end irrespective of their order in children. Also, if an item is being dragged and an update occurs at that moment, that item will be placed at respective location and onPositionChange
will be called to prevent any inconsistency.
NOTE: The props watchChildrenUpdates
and preserveOrder
should be used carefully to avoid any unexpected behaviour
import React, { useState } from "react";
import ReorderList from "react-reorder-list";
export default function App() {
const [array, setArray] = useState([0, 1, 2, 3, 4]);
function setNewArray() {
setArray((prev) => {
const array = [];
prev.forEach((_) => {
do {
var item = Math.floor(Math.random() * 9);
} while (array.includes(item));
array.push(item);
});
return array;
});
}
return (
<div>
<ReorderList watchChildrenUpdates={true} animationDuration={200}>
{array.map((i) => (
<div key={i}>Item {i}</div>
))}
</ReorderList>
<button onClick={setNewArray}>Click me</button>
</div>
);
}
import React from "react";
import ReorderList from "react-reorder-list";
export default function App() {
return (
<ReorderList>
<div key="div" data-disable-reorder={true}>
This div cannot be reordered
</div>
{[0, 1, 2, 3, 4].map((i) => {
return <div key={i}>Item {i}</div>; // Having a unique key is important
})}
<p key="p" data-disable-reorder={true}>
This p cannot be reordered either
</p>
</ReorderList>
);
}
import React from "react";
import ReorderList, { ReorderIcon } from "react-reorder-list";
export default function App() {
return (
<ReorderList>
{[0, 1, 2].map((i) => {
return (
<div key={i}>
<ReorderIcon />
<span>{"Parent" + i}</span>
<ReorderList useOnlyIconToDrag={true}>
{[0, 1, 2].map((j) => {
return (
<div key={j} style={{ paddingLeft: "16px" }}>
<ReorderIcon />
<span>{"Child" + i + j}</span>
</div>
);
})}
</ReorderList>
</div>
);
})}
</ReorderList>
);
}
Here is the full API for the <ReorderList>
component, these properties can be set on an instance of ReorderList:
Parameter | Type | Required | Default | Description |
---|---|---|---|---|
useOnlyIconToDrag | boolean | No | false | See usage with ReorderIcon |
selectedItemOpacity | number (0 to 1) | No | 0.5 | This determines the opacity of the item being dragged, until released. |
animationDuration | number | No | 400 | The duration (in ms) of swapping animation between items. If set to 0, animation will be disabled. |
watchChildrenUpdates | boolean | No | false | Enable this to listen to any updates in children of <ReorderList> and update the state accordingly. See listen to children updates |
preserveOrder | boolean | No | false | Works along woth watchChildrenUpdates to determine whether to preserve existing order or not. See listen to children updates |
onPositionChange | PositionChangeHandler | No | - | Function to be executed on item position change. |
disabled | boolean | No | false | When set to true, <ReorderList> will work as a plain div with no functionality. |
props | React.DetailedHTMLProps | No | - | Props to customize the <ReorderList> component. |
import { ReactNode } from "react";
type RevertHandler = () => void;
type PositionChangeParams = {
start?: number; // Index of the item being dragged
end?: number; // Index of the item being displaced by the starting item
oldItems?: ReactNode[]; // Array of children before reordering
newItems?: ReactNode[]; // Array of children after reordering
revert: RevertHandler; // A fallback handler to revert the reordering
};
type PositionChangeHandler = (params?: PositionChangeParams) => void;
FAQs
A simple react component that facilitates the reordering of JSX/HTML elements through drag-and-drop functionality, allowing for easy position changes.
The npm package react-reorder-list receives a total of 11 weekly downloads. As such, react-reorder-list popularity was classified as not popular.
We found that react-reorder-list demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.