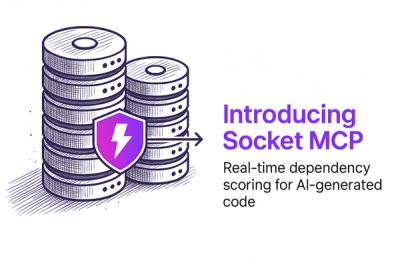
Product
Secure Your AI-Generated Code with Socket MCP
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
react-rest-cache
Advanced tools
This library allows to query a REST API using React hooks, and cache the results in a global cache. All objects in the API should have:
__typename
string field (e.g. User
, Post
, etc…)id
string field (e.g. id1
, id2
, etc…)The library will synchronize all objects and trigger re-renders when the cache is updated.
It aims to provide a similar experience to Apollo client but with REST APIs.
import { Provider, RestCache } from "react-rest-cache";
const restCache = RestCache({
baseUrl: "https://api.example.com",
});
const App = () => {
return (
<Provider restCache={restCache}>
<MyComponent />
</Provider>
);
};
import { useQuery, useMutation } from "react-rest-cache";
type Post = {
id: string;
title: string;
};
type User = {
id: string;
name: string;
posts: Post[];
};
const MyComponent = () => {
const { data, error, loading } = useQuery<User[]>("/users");
// The /users endpoint will return something like:
// [
// {
// __typename: "User",
// id: "id1",
// name: "John",
// posts: [
// {
// __typename: "Post",
// id: "id2",
// title: "Hello world",
// },
// ],
// },
// {
// __typename: "User",
// id: "id2",
// name: "Jane",
// posts: []
// },
// ]
if (loading) {
return <div>Loading…</div>;
}
if (error) {
return <div>Error: {error.message}</div>;
}
return (
<div>
{data.map((user) => (
<div key={user.id}>{user.name}</div>
))}
</div>
);
};
const MyButton = () => {
const [mutate, { data, error, loading }] = useMutation<User>(`/users/id2`, {
method: "PUT",
});
return (
<div>
{error ? <div>Error: {error.message}</div> : null}
{loading ? <div>Loading…</div> : null}
<button onClick={() => mutate({ body: { name: "John" } })}>Update</button>
</div>
);
};
This project is currently a big WIP. I don’t recommand you use it yet.
Features will be added progressively, while staying as simple as possible. This library won’t offer as much control or features as Apollo provides. If you need more control, you should use Apollo.
What I plan to add:
FAQs
React library to fetch a REST API with cache and sync capabilities
The npm package react-rest-cache receives a total of 9 weekly downloads. As such, react-rest-cache popularity was classified as not popular.
We found that react-rest-cache demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket MCP brings real-time security checks to AI-generated code, helping developers catch risky dependencies before they enter the codebase.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.