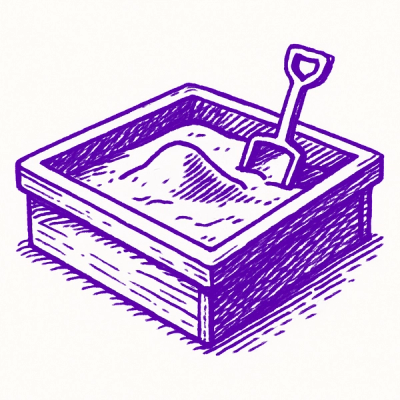
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
react-swipeable-list
Advanced tools
Demo • Installation • Usage
You like it? Buy me a coffee :) or a 🍺
A react component to render list with swipeable items. Items can have one or more actions on left (leading) and right (trailing) swipe and different behavior depending on props. See examples
This repository contains new version of sandstreamdev/react-swipeable-list/. Whole component was reimplemented to support buttons in revealed content and different swipe behaviors. More information can be found in this issue: Clarify relationship with @sandstreamdev/react-swipeable-list
Check working example page
npm install react-swipeable-list
# or via yarn
yarn add react-swipeable-list
import {
LeadingActions,
SwipeableList,
SwipeableListItem,
SwipeAction,
TrailingActions,
} from 'react-swipeable-list';
import 'react-swipeable-list/dist/styles.css';
const leadingActions = () => (
<LeadingActions>
<SwipeAction onClick={() => console.info('swipe action triggered')}>
Action name
</SwipeAction>
</LeadingActions>
);
const trailingActions = () => (
<TrailingActions>
<SwipeAction
destructive={true}
onClick={() => console.info('swipe action triggered')}
>
Delete
</SwipeAction>
</TrailingActions>
);
<SwipeableList>
<SwipeableListItem
leadingActions={leadingActions()}
trailingActions={trailingActions()}
>
Item content
</SwipeableListItem>
</SwipeableList>;
Type: milliseconds
(optional, default: 0
)
Time in milliseconds after which swipe action and animation should be called after trigggering swipe action.
It can be set for the whole list or for every item. See actionDelay
for SwipeableListItem
. Value from the SwipeableListItem
takes precedence.
Type: boolean
(optional, default: false
)
Changes behavior of IOS
list type.
When true
and swipe is done beyond threshold
and released the action is triggered.
When set to false
actions are only opened and they need to be clicked to trigger action.
Type: milliseconds
(optional, default: 1000
)
Time in milliseconds after which swipe action should be called for destructive
swipe action (item deletion).
It can be set for the whole list or for every item. See destructiveCallbackDelay
for SwipeableListItem
. Value from the SwipeableListItem
takes precedence.
Type: object
(optional, default: undefined
)
Additional styles for list tag.
Type: ListType (ANDROID | IOS | MS)
(optional, default: ANDROID
)
Changes behavior of swipeable items.
ANDROID
IOS
MS
Type: string
(optional, default: div
)
HTML tag that is used to create this component.
Type: number
(optional, default: 10
)
How far in pixels scroll needs to be done to block swiping. After scrolling is started and goes beyond the threshold, swiping is blocked.
It can be set for the whole list or for every item. See scrollStartThreshold
for SwipeableListItem
. Value from the SwipeableListItem
takes precedence.
Type: number
(optional, default: 10
)
How far in pixels swipe needs to be done to start swiping on list item. After a swipe is started and goes beyond the threshold, scrolling is blocked.
It can be set for the whole list or for every item. See swipeStartThreshold
for SwipeableListItem
. Value from the SwipeableListItem
takes precedence.
Type: number
(optional, default: 0.5
)
How far swipe needs to be done to trigger attached action. 0.5
means that item needs to be swiped to half of its width, 0.25
- one-quarter of width.
It can be set for the whole list or for every item. See threshold
for SwipeableListItem
. Value from the SwipeableListItem
takes precedence.
Type: milliseconds
(optional, default: 0
)
Time in milliseconds after which swipe action and animation should be called after trigggering swipe action.
It can be set for the whole list or for every item. See actionDelay
for SwipeableList
. Value from the SwipeableListItem
takes precedence.
Type: boolean
(optional, default: false
)
If set to true
all defined swipe actions are blocked.
Type: milliseconds
(optional, default: 1000
)
Time in milliseconds after which swipe action should be called for destructive
swipe action (item deletion).
It can be set for the whole list or for every item. See destructiveCallbackDelay
for SwipeableList
. Value from the SwipeableListItem
takes precedence.
Type: LeadingActions component
Container component that sets up correct props in SwipeAction
. See examples for usage.
Type: 'number' (optional, default: 1.0
)
Limit the swipe to percent of width, e.g.: 0.5
will make swipe possible only for 50% of elements's width
Type: function
(optional)
Callback function that should be call after list item is clicked.
Type: (dragDirection: string) => void
Fired after swipe has started (after drag gesture passes the swipeStartThreshold
distance in pixels). dragDirection
can have value of left
or right
.
Type: (dragDirection: string) => void
Fired after swipe has ended. dragDirection
can have value of left
or right
.
Type: (progress: number, dragDirection: string) => void
Fired every time swipe progress changes. The reported progress
value is always an integer in range 0 to 100 inclusive. dragDirection
can have value of left
or right
.
Type: number
(default: 10
)
How far in pixels scroll needs to be done to block swiping. After scrolling is started and goes beyond the threshold, swiping is blocked.
It can be set for the whole list or for every item. See scrollStartThreshold
for SwipeableList
. Value from the SwipeableListItem
takes precedence.
Type: number
(default: 10
)
How far in pixels swipe needs to be done to start swiping on list item. After a swipe is started and goes beyond the threshold, scrolling is blocked.
It can be set for the whole list or for every item. See swipeStartThreshold
for SwipeableList
. Value from the SwipeableListItem
takes precedence.
Type: number
(default: 0.5
)
How far swipe needs to be done to trigger action. 0.5
means that item needs to be swiped to half of its width, 0.25
- one-quarter of width.
It can be set for the whole list or for every item. See threshold
for SwipeableList
. Value from the SwipeableListItem
takes precedence.
Type: TrailingActions component
Container component that sets up correct props in SwipeAction
. See examples for usage.
Type: boolean
(optional, default: false
)
If set to true
then remove animation is played and callback is called after destructiveCallbackDelay
.
Type: function
(required)
Callback function that should be call after swipe action is triggered.
Type: string
(optional, default: span
)
HTML tag that is used to create this component.
Thanks goes to these wonderful people (emoji key):
Marek Rozmus 💻 📖 ⚠️ 💡 🤔 🚧 🎨 | Adam Laycock 🐛 | Rathes Sachchithananthan 🤔 | Niels 🤔 | Glenn Harris 🚧 | Zdorovtsev Viktor 🤔 | Zakaria EL Mesaoudi 💻 |
Aron Rotteveel 💻 | Lucas Furini 🤔 | Canberk Akartuna 🐛 | Raul Escobar 🐛 | Starly26 🐛 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Swipeable list component for React
The npm package react-swipeable-list receives a total of 9,730 weekly downloads. As such, react-swipeable-list popularity was classified as popular.
We found that react-swipeable-list demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.