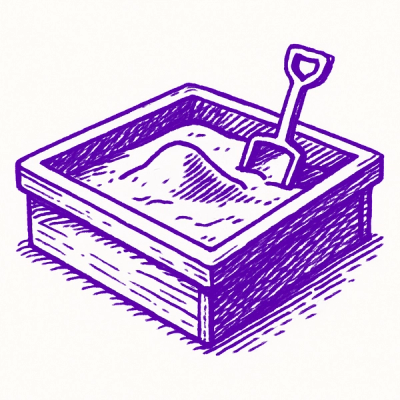
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
react-swipeable-views-utils
Advanced tools
The react-swipeable-views-utils package provides additional utilities for the react-swipeable-views library, which allows for the creation of swipeable views in React applications. These utilities enhance the functionality of swipeable views by offering features such as virtualized lists and auto-play capabilities.
AutoPlay
The AutoPlay utility enhances SwipeableViews by adding auto-play functionality, allowing the views to automatically transition between slides at a specified interval.
import { autoPlay } from 'react-swipeable-views-utils';
import SwipeableViews from 'react-swipeable-views';
const AutoPlaySwipeableViews = autoPlay(SwipeableViews);
function MyComponent() {
return (
<AutoPlaySwipeableViews>
<div>Slide 1</div>
<div>Slide 2</div>
<div>Slide 3</div>
</AutoPlaySwipeableViews>
);
}
Virtualize
The Virtualize utility allows for the creation of virtualized swipeable views, which can improve performance by only rendering the slides that are currently visible.
import { virtualize } from 'react-swipeable-views-utils';
import SwipeableViews from 'react-swipeable-views';
const VirtualizeSwipeableViews = virtualize(SwipeableViews);
function slideRenderer(params) {
const { index, key } = params;
return <div key={key}>Slide {index}</div>;
}
function MyComponent() {
return (
<VirtualizeSwipeableViews slideRenderer={slideRenderer} />
);
}
react-slick is a React component for creating carousels and sliders. It offers a wide range of features including auto-play, lazy loading, and responsive design. Compared to react-swipeable-views-utils, react-slick is more focused on providing a comprehensive slider solution with a rich set of features.
react-carousel is another React component for creating carousels. It provides basic carousel functionality with support for custom animations and auto-play. While it offers similar features to react-swipeable-views-utils, it is generally simpler and more lightweight.
pure-react-carousel is a highly customizable and accessible carousel component for React. It provides a range of features including auto-play, infinite scrolling, and custom controls. It is similar to react-swipeable-views-utils in terms of functionality but places a stronger emphasis on accessibility and customization.
FAQs
react-swipeable-views utility modules
The npm package react-swipeable-views-utils receives a total of 204,994 weekly downloads. As such, react-swipeable-views-utils popularity was classified as popular.
We found that react-swipeable-views-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.