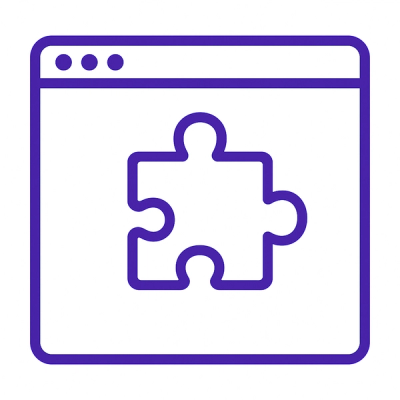
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
react-vega
Advanced tools
Use
vega
orvega-lite
inreact
application smoothly!
DEMO: http://vega.github.io/react-vega/
npm install react vega vega-lite react-vega --save
react-vega@7.x.x
is rewritten in typescript with several API changes and now support both vega
and vega-lite
. If you are upgrading from react-vega
or react-vega-lite
version 6.x.x
to 7.x.x
, read this migration guide.react-vega@6.x.x
is same with 5.x.x
but output are in different directories and exported as both commonjs
and es module
.react-vega@5.x.x
uses vega
again.react-vega@4.x.x
has same interface with 3.x.x
except it uses the lightweight vega-lib
instead of vega
.react-vega@3.x.x
was update with breaking changes to support vega@3.0
.react
with vega@2.x
, please use react-vega@2.3.1
.There are two approaches to use this library.
See the rest of the spec in spec1.ts.
import React, { PropTypes } from 'react';
import { createClassFromSpec } from 'react-vega';
export default createClassFromSpec('BarChart', {
"width": 400,
"height": 200,
"data": [{ "name": "table" }],
"signals": [
{
"name": "tooltip",
"value": {},
"on": [
{"events": "rect:mouseover", "update": "datum"},
{"events": "rect:mouseout", "update": "{}"}
]
}
],
... // See the rest in packages/react-vega-demo/stories/vega/spec1.ts
});
import React from 'react';
import ReactDOM from 'react-dom';
import BarChart from './BarChart.js';
const barData = {
table: [...]
};
function handleHover(...args){
console.log(args);
}
const signalListeners = { hover: handleHover };
ReactDOM.render(
<BarChart data={barData} signalListeners={signalListeners} />,
document.getElementById('bar-container')
);
<Vega>
generic class and pass in spec
for dynamic component.Provides a bit more flexibility, but at the cost of extra checks for spec changes.
import React from 'react';
import ReactDOM from 'react-dom';
import { Vega } from 'react-vega';
const spec = {
"width": 400,
"height": 200,
"data": [{ "name": "table" }],
"signals": [
{
"name": "tooltip",
"value": {},
"on": [
{"events": "rect:mouseover", "update": "datum"},
{"events": "rect:mouseout", "update": "{}"}
]
}
],
... // See the rest in packages/react-vega-demo/stories/vega/spec1.ts
}
const barData = {
table: [...]
};
function handleHover(...args){
console.log(args);
}
const signalListeners = { hover: handleHover };
ReactDOM.render(
<Vega spec={spec} data={barData} signalListeners={signalListeners} />,
document.getElementById('bar-container')
);
<VegaLite>
generic class and pass in spec
for dynamic component.Provides a bit more flexibility, but at the cost of extra checks for spec changes.
Also see packages/react-vega-demo/stories/ReactVegaLiteDemo.jsx for details
import React from 'react'
import ReactDOM from 'react-dom'
import { VegaLite } from 'react-vega'
const spec = {
width: 400,
height: 200,
mark: 'bar',
encoding: {
x: { field: 'a', type: 'ordinal' },
y: { field: 'b', type: 'quantitative' },
},
data: { name: 'table' }, // note: vega-lite data attribute is a plain object instead of an array
}
const barData = {
table: [
{ a: 'A', b: 28 },
{ a: 'B', b: 55 },
{ a: 'C', b: 43 },
{ a: 'D', b: 91 },
{ a: 'E', b: 81 },
{ a: 'F', b: 53 },
{ a: 'G', b: 19 },
{ a: 'H', b: 87 },
{ a: 'I', b: 52 },
],
}
ReactDOM.render(
<VegaLite spec={spec} data={barData} />,
document.getElementById('bar-container')
);
React class Vega
and any output class from createClassFromSpec
have these properties:
mode
, theme
, defaultStyle
, renderer
, logLovel
, tooltip
, loader
, patch
, width
, height
, padding
, actions
, scaleFactor
, config
, editorUrl
, sourceHeader
, sourceFooter
, hover
, i18n
, downloadFileName
class and style of the container <div>
element
For data
, this property takes an Object with keys being dataset names defined in the spec's data field, such as:
var barData = {
table: [{"x": 1, "y": 28}, {"x": 2, "y": 55}, ...]
};
Each value can be an array or function(dataset){...}
. If the value is a function, Vega's vis.data(dataName)
will be passed as the argument dataset
. If you are using <VegaLite>
make sure to enable your tooltip in the the spec, as described here.
var barData = {
table: function(dataset){...}
};
In the example above, vis.data('table')
will be passed as dataset
.
All signals defined in the spec can be listened to via signalListeners
.
For example, to listen to signal hover, attach a listener like this
// better declare outside of render function
const signalListeners = { hover: handleHover };
<Vega spec={spec} data={barData} signalListeners={signalListeners} />
Any class created from createClassFromSpec
will have this method.
spec
You can pass the vega-tooltip
handler instance to the tooltip
property.
import { Handler } from 'vega-tooltip';
<Vega spec={spec} data={barData} tooltip={new Handler().call} />
FAQs
Convert Vega spec into React class conveniently
The npm package react-vega receives a total of 50,948 weekly downloads. As such, react-vega popularity was classified as popular.
We found that react-vega demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.