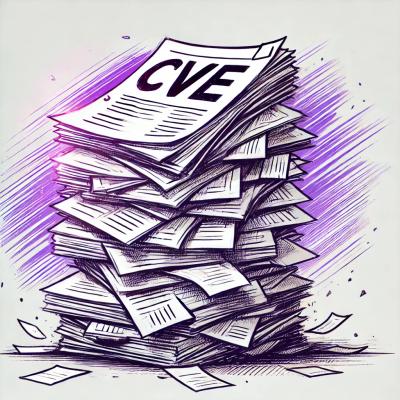
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Universal React+GraphQL starter kit: React, Apollo, Webpack 2, React Router 4, PostCSS, SSR
React for UI. Apollo for GraphQL. Browser + server-side rendering.
Maintained and updated regularly.
<head>
section, using react-helmet.gz
filesThis starter kit is built primarily for OS X, but should work with most flavours of *nix and Windows (hopefully.)
You'll need Node.js installed.
For now, simply clone the starter kit:
git clone --depth 1 https://github.com/leebenson/reactql <project_folder>
... then install the required packages:
cd <project_folder>
npm i
... and start writing over it.
In future, you'll be able to install globally via NPM and generate projects with a few other goodies such as code generation, deletion of the .git
folder, etc.
Simply run:
npm start
... and a server will spawn (by default) on http://localhost:8080
Changes to your React components/styles will update in the browser in real-time, with no refreshes. Changes to other code may require a refresh.
This starter kit includes a server.js
entry point, that spawns a Koa 2 web server.
To run it, you first need to build both the server and client bundles with:
npm run build
Then, simply run:
npm run server
... which (by default) spawns a server at http://localhost:4000
Or, you can run the short-cut: npm run build-run
, which has the same effect as running the above two commands separately.
This starter has been designed to provide you with a few skeletal pieces to get you going, and make it easier to layer in your own code.
Here's the folder layout:
.git
Git folder. By default, this points to the reactql starter kit, so you can safely delete this if you want to initialise your own git repo and start contributing to it.
kit
The bulk of reactql is found in ./kit
. If you need to dive into the Webpack config or add some custom functionality to your web server or browser instantiation, you'd do it here.
For the most part though, you probably won't need to touch this stuff.
kit/entry
This is where Webpack looks for entry points for building the browser and the server.
Note: There's no separate entry for 'vendors', since the bundle is built automatically by testing whether packages reside in node_modules
kit/lib
Custom libraries built for reactql. Currently empty.
kit/repo
Non-code files specific to the starter kit. For example, the reactql logo that github pulls. You can safely delete this folder, if you want.
kit/webpack
Webpack configuration files, notably:
base.js
: Base configuration that all others inherit fromeslint.js
: Entry point for the linter, to properly follow local module folders and avoid false positivesbrowser.js
: Base configuration for the browser. Inherits from base, and is extended by browser_dev and browser_prodbrowser_dev.js
: Config for the browser spawned at http://localhost:8080 when running npm start
browser_prod.js
: Production browser bundling. Minifies and crunches code and images, gzips assets, removes source maps and debug flags, builds your browser bundles ready for production. This is automatically served back to the client when you run npm run build-run
server.js
: Node.js web server bundle. Even though we're running this through Node, Webpack still gets involved and properly handles inline file and CSS loading, and generates a build that works with your locally installed Node.js. You can build the server with npm run build-server
or build and run with npm run build-run
src
This is where all of your own code will live. By default, it contains the <App>
component in app.js
which demonstrates a few of the concepts that this starter kit offers you, along with styles defined in app.css
.
You can overwrite these files directly with your own code and use this as a starter point.
static
Put static files here that you want to wind up serving in production.
Webpack will automatically crunch any of your GIF/JPEG/PNG/SVG images it finds in here, as well as generate pre-compressed .gz
versions of every file so that your Koa web server on http://localhost:4000 (after running npm run build-run
) can serve up the compressed versions instead of the full-sized originals.
There are various configuration files that you'll find in the root:
.babelrc
: This exists solely to transpile the ES6 Webpack config into something Node.js can natively run. It has no bearing on the Babel config that's used to transpile your Webpack code, so you can safely this leave alone.
.eslint.js
: ESLint configuration. If your editor/IDE has ESLint installed, it will use this file to lint your code. See Tweaked Airbnb style guide below to see the rules set for this starter kit.
.eslintignore
: Files the linter can safely ignore.
.gitignore
: Files to ignore when checking in your code. This is built around the reactql starter kit, but you will probably want to use it as a base for your own code since it ignores the usual Node stuff, along with dist
and some of the caching folders used by Webpack.
package.json
: NPM packages used in this starter kit. When you're extending this kit with your own code, you'll probably want to gut out the name, description and repo links and replace with your own. Just keep dependencies
and devDependencies
intact for the kit to continue to work.
paths.js
: File paths to different parts of the kit. Used by Webpack to determine what lives where.
webpack.config.babel.js
: The Webpack entry point. This will invoke the config found in kit/webpack
per the npm run...
command that spawned it.
If you're using an editor to write code against this starter kit with ESLint powers, you might notice squiggly red lines under perfectly innocent blocks of Javascript code.
That's because this starter kit's eslinting is based on Airbnb style guide, which enforces consistently across your code base.
I've made a few tweaks based on personal preference:
async/await
is perfectly valid - go nuts!for/of
loops are legal syntax (Airbnb forbids them in favour of functional programming; I happen to think they have a valid place alongside generators or Promise where serial await
s are a feature, not a bug)propTypes
should be a known shape-- 'any' is forbidden++
and --
are allowed as the final statement in a for
loop.js
or .jsx
filesrequire
statements are allowed. Since we're targeting a universal code base in dev and production, there may be code you need to import conditionallynode_modules
(so we can write import x from 'src/someLocalFile'
instead of requiring paths relative from the caller)<Component
someAttribute={true} />
(I just think it looks neater)
CSS can be written and placed anywhere. A common pattern I use is to write the .css
alongside regular .js
files, so they 'live' in the same place.
You can import and use styles in your code like regular Javascript:
Inside your CSS:
someCssFile.css
.someClass {
font-weight: bold;
text-align: center;
color: red;
}
someJsFile.js
import css from './someCssFile.css';
// .someClass will get transformed into a hashed, 'local' name, to avoid
// collisions with the global namespace
export default props => (
<div>
<p className={css.someClass}>Hello world</p>
</div>
)
.css stylesheets are parsed through CSSNext using PostCSS, using the default settings for cssnext.
That means you can use things like nested statements out-the-box:
.anotherClassName {
& h1 {
text-size: 2em;
}
& p {
color: green;
}
}
As well as variable names, and other goodies:
:root {
--mainColor: red;
}
a {
color: var(--mainColor);
}
Vendor prefixes will automatically be added, so you can write the bare CSS and let PostCSS worry about polyfilling browsers.
SASS code is also compiled through PostCSS in the same way. Simply use .sass
/.scss
files instead, and your stylesheets will pass through node-sass first.
By default, class styles in your CSS/SASS files will be exported as local modules. Class names will be hashed to prevent bleeding out to your global namespace, and those same class names are then injected into the React code that is bundled for you. e.g:
The benefit to this is tight coupling of style logic with your components. You don't have to worry that you've used globally unique class names, because Webpack will transpile your names into garbled, hashed versions to avoid conflicts.
If you want to override the 'local by default' classnames, simply prefix :global
before the rule and it'll be made available across your entire app:
:global (.thisClassWontRename) {
font-color: blue;
}
In this case, .thisClassWontRename
will be the exact name used in the output to your final .css file, so you can use this class name wherever you want the style to apply - anywhere in your code.
In development, your styles will be bundled with full source maps into the resulting Javascript that's generated by Webpack Dev Server. No external CSS file is created or called.
In production, code is extracted out into a final style.css
file that is automatically minified and included in the first-page HTML via a <link>
tag that's sent from the server-side. Webpack will do the heavy lifting and match up your React names with the CSS localised styles, so it'll work exactly the same way as in dev.
You don't need to do anything for this to happen- reactql takes care of it for you.
Yes! In the server bundle, styles are extracted out and use the same local names as your hashed classes, so you can import styles on the server in exactly the same way.
When you import
or require
modules, Webpack (and ESLint) will first look in your root project folder before checking node_modules
.
This allows you to short-hand writing long, relative path names.
e.g. instead of writing:
import x from '../../../components/some/file.js';
... you can instead simply write:
import x from 'src/components/some/file.js';
The same is true of any filetype that Webpack recognises- .jpg, .css, .sass, .json, etc:
// If, say, you wish to put all CSS code in a `styles` folder in the project root
import style from 'styles/some/file.css';
This starter kit is the product of over 2 years working with React, and GraphQL since its initial public release.
IMO, this represents the best available tooling for web apps in 2017. I use this stuff every day in production, so you can be confident it'll be maintained and updated regularly.
Use ReactQL if you want to skip the set-up, and get your next project up in record time.
Some of the third-party packages in my stack may be in alpha or beta stages, so it's up to you to evaluate if they're a good fit for your application.
I personally use this starter kit for every new React project I undertake, many of which are running successfully under heavy production load -- so I'm quite comfortable with the tech choices.
Anything 'front end' that's backed by a GraphQL server. I typically break my projects down into a few pieces:
Front end, with a built-in web server that serves public traffic. This usually sits behind an Nginx proxy/load balancer and compiles and dumps back the UI. This is basically ReactQL.
GraphQL API. This could be Node.js, Python, Go, etc. But it's usually separate to the front-end code base, and is something the front-end talks to directly via the Apollo GraphQL client. I generally don't use ReactQL for this unless there's a good reason to build a monolithic app (e.g. speed, the back-end not doing a whole lot, etc.)
Microservices. Smaller pieces of the stack that do one thing, and do it well. The API server is responsible for talking to this stuff. Again, ReactQL doesn't really get a look in.
I'd generally recommend a similar layout to keep your code light and maintainable. With that said, if you want Node.js to handle both your front and back-office concerns, there's no reason you couldn't write that stuff here too, and keep it scoped to the server. I just prefer to keep it separate.
Hopefully. I haven't tested it. But there's no OS X/Linux specific commands that are used to build stuff, so it should work.
There's currently no test library, so feel free to add your own.
src/app.js
is the main React component, that both the browser and the serve will look to. Overwrite it with your own code.
If you need to edit the build defaults, you can start digging into the kit
dir which contains the 'under the hood' build stuff. But that generally comes later.
git clone --depth 1 https://github.com/leebenson/reactql <project_folder>
cd
into the folder, then install packages with npm i
npm start
This will spawn a dev server on http://localhost:8080
To build in production, do steps 1-2 above then run npm run build-run
FAQs
Universal React+GraphQL starter kit: React, Apollo, Webpack 2, React Router 4, PostCSS, SSR
The npm package reactql receives a total of 10 weekly downloads. As such, reactql popularity was classified as not popular.
We found that reactql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.